Deprecated ways of handling notification clicks
If you are looking for our legacy ways of handling notification clicks, we've saved the documentation for you.
Handling click broadcasts (deprecated)
When a notification is clicked whose action is to open the special URL wonderpush://notificationOpen/broadcast
, our SDKs forward this click to your app or website without taking any other action.
Such notifications can be created from the online dashboard by entering wonderpush://notificationOpen/broadcast
as the On-click URL in the composition form.
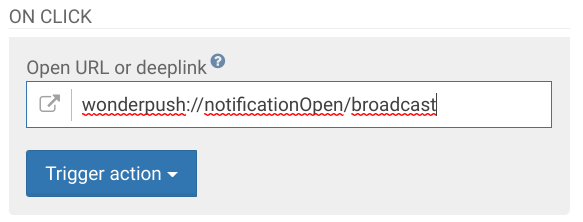
You can listen to these clicks and decide what to do by adding a small amount of code.
Handling click broadcasts on a Website requires updating a Service WorkerIf you are not familiar with Service Workers, you'll find Getting started articles on MDN or Google.
Handling click broadcasts on a Website is only possible if you are using your own push notification domain. If you are using a *.by.wonderpush.com
domain, it is not currently possible to consume data from push notification payloads.
// Only websites using their own push notification domain can handle click broadcasts
// This code goes in the wonderpush-worker-loader.min.js service worker file you've installed at the root of your website when setting up WonderPush
self.addEventListener('WonderPushEvent', function(event) {
// Click broadcasts are events with .detail.type === 'notificationOpened'
if (event && event.detail && event.detail.type === 'notificationOpened') {
// The event object contains the original 'notificationclick' event object
// in its .detail.event property.
// The notification clicked is available like so:
console.log('Notification clicked:', event.detail.event.notification);
}
});
// Listen to a NotificationCenter notification.
// A good place to put this code is your AppDelegate's application:didFinishLaunchingWithOptions:
NotificationCenter.default.addObserver(forName: NSNotification.Name(rawValue: WP_NOTIFICATION_OPENED_BROADCAST), object: nil, queue: nil, using: {notification in
if let pushNotification = notification.userInfo {
debugPrint("Notification clicked:", pushNotification)
}
})
// Listen to a NSNotificationCenter notification.
// A good place to put this code is your AppDelegate's application:didFinishLaunchingWithOptions:
[[NSNotificationCenter defaultCenter] addObserverForName:WP_NOTIFICATION_OPENED_BROADCAST object:nil queue:nil usingBlock:^(NSNotification *note) {
NSDictionary *pushNotification = note.userInfo;
NSLog(@"Notification clicked: %@", pushNotification);
}];
LocalBroadcastManager.getInstance(this).registerReceiver(new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
if (!WonderPush.INTENT_NOTIFICATION_WILL_OPEN_EXTRA_NOTIFICATION_TYPE_DATA.equals(
intent.getStringExtra(WonderPush.INTENT_NOTIFICATION_WILL_OPEN_EXTRA_NOTIFICATION_TYPE))) {
Intent pushNotif = intent.getParcelableExtra(WonderPush.INTENT_NOTIFICATION_WILL_OPEN_EXTRA_RECEIVED_PUSH_NOTIFICATION);
Log.d("WonderPush", pushNotif.toString());
// You should now start your application on the appropriate screen.
}
}
}, new IntentFilter(WonderPush.INTENT_NOTIFICATION_WILL_OPEN));
document.addEventListener('wonderpush.notificationOpen', function onNotificationOpen(event) {
// This is called for data notifications when they are received and for regular notifications when they are clicked
if (event.notificationType != "data") {
console.log("Clicked notification:", event.notification);
// On Android, you should now start your application on the appropriate screen.
}
}, false);
WonderPush.eventEmitterWonderPush.addListener('wonderpushNotificationWillOpen', (event) => {
console.log('Notification clicked:', event);
// On Android, you should now start your application on the appropriate screen.
});
This feature is not supported yet on Flutter.
We'd like to hear about your use case.
Registering a callback (deprecated)
Registering a callback allows you to execute some code when a push notification is clicked.
Please note that deep links are a good way of achieving the same thing on mobile applications.
Users of the online dashboard can plug your code directly inside their notification buttons by choosing the option to trigger a Call method from the composition form of the notification edition interface:
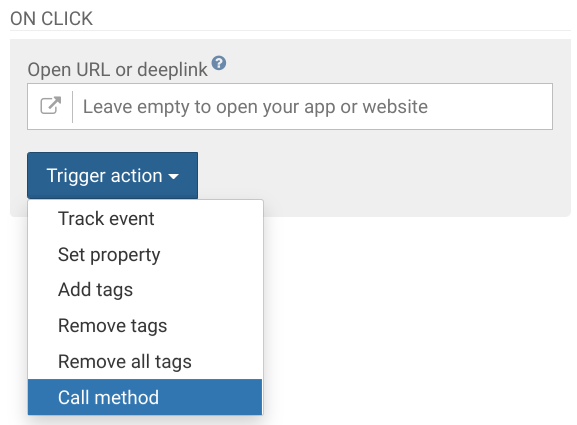
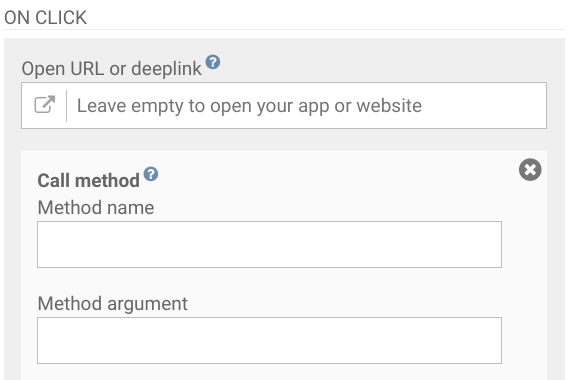
This technique is not available in our Website SDK
To register a callback, you'll need a few lines of code. In the following example, we register a callback named example
:
// Put the following call in the [application:didFinishLaunchingWithOptions:] method of your AppDelegate
// Here is how to register the callback named "example"
NotificationCenter.default.addObserver(forName: NSNotification.Name(rawValue: "example"), object: nil, queue: nil, using: {notification in
let arg = notification.userInfo?[WP_REGISTERED_CALLBACK_PARAMETER_KEY] as? String
// Do something useful here
})
// Put the following call in the [application:didFinishLaunchingWithOptions:] method of your AppDelegate
// Here is how to register the callback named "example"
[[NSNotificationCenter defaultCenter] addObserverForName:@"example" object:nil queue:nil usingBlock:^(NSNotification *note) {
NSString *arg = [note.userInfo objectForKey:WP_REGISTERED_CALLBACK_PARAMETER_KEY];
// Do something useful here
}];
// Put the following call before you initialize the SDK, in your Application class
// Here is how to register the callback named "example"
IntentFilter exampleMethodIntentFilter = new IntentFilter();
exampleMethodIntentFilter.addAction(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_ACTION);
exampleMethodIntentFilter.addDataScheme(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_SCHEME);
exampleMethodIntentFilter.addDataAuthority(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_AUTHORITY, null);
exampleMethodIntentFilter.addDataPath("/example", PatternMatcher.PATTERN_LITERAL); // Note: prepend a / to the actual method name
LocalBroadcastManager.getInstance(this).registerReceiver(new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String arg = intent.getStringExtra(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_EXTRA_ARG);
// Do something useful here
}
}, exampleMethodIntentFilter);
document.addEventListener('wonderpush.registeredCallback', function(event) {
console.log("Callback called for method", event.method, "with arg", event.arg);
}, false);
This feature is not supported yet on React Native.
We'd like to hear about your use case.
In the meantime, you can probably achieve what you're trying to do with a deep link
This feature is not supported yet on Flutter.
We'd like to hear about your use case.
In the meantime, you can probably achieve what you're trying to do with a deep link
Updated 19 days ago