Popup SDK reference
About the Popup Javascript SDK
The popup Javascript SDK is intended for use within Popups. If you are not familiar with Popups, please see Getting started with Popups.
To include the Popup Javascript SDK in your popups, make sure the following script
tag is in your popup HTML:
<script type="text/javascript" src="https://cdn.by.wonderpush.com/inapp-sdk/1/wonderpush-loader.min.js"></script>
Before you get started
All methods in the Popup SDK return Promise
objects, even those who do not send a result back. If you are not familiar with Promise
objects, there is a good documentation on MDN
Methods of the WonderPushPopupSDK
are all asynchronous. Promises guarantee that a method has finished its job.
Make sure methods have finished their jobs before dismissing the popup or opening a link:
WonderPushPopupSDK.trackEvent('SomeEvent').then(function() {
// We wait for the event to be tracked before dismissing the popup
WonderPushPopupSDK.dismiss();
});
Popups are available since the version 4.1.2 of our iOS SDK, and the version 4.3.0 of our Android SDK. See the complete compatibility table for Cordova, Ionic, Flutter and ReactNative. All methods in this reference are available since the same versions, unless otherwise stated.Websites automatically get the latest version of our SDK and can use all methods.
Thewindow.WonderPushPopupSDK
variable used to be calledwindow.WonderPushInAppSDK
, starting iOS SDK v4.1.2 and Android SDK v4.3.0
Both names are valid in following releases.
Reference
Linking | |
Tries to open the provided URL inside the app, or in the current window (websites). Dismisses the popup. | |
Opens the provided URL in the default browser (apps) or in a new window (websites). Dismisses the popup. | |
Tracks a click in WonderPush. You can get click data from the popup's detail page. | |
Dismissing | |
Dismisses the popup | |
Subscribing users | |
Prompts user to subscribe to web push notifications. | |
Unsubscribes user from web push notifications. | |
Tells whether user is subscribed to web push notifications. | |
Returns a Promise that resolves to a string equal to the user ID or | |
Returns a Promise that resolves to a string equal to the installation ID, | |
Segmentation | |
Sends an event with a name and payload of your choice. | |
Adds one or more tags to this installation. | |
Removes one or more tags from this installation. | |
Removes all tags from this installation. | |
Tests whether a given tag is attached to this installation. | |
Returns all the tags attached of this installation. | |
Returns the value of a given property associated to this installation. | |
Returns an immutable list of the values of a given property associated to this installation. | |
Adds the value to a given property associated to this installation. | |
Removes the value from a given property associated to this installation. | |
Sets the value to a given property associated to this installation. | |
Removes the value of a given property associated to this installation. | |
Associates the provided name/value pairs to this installation. | |
Returns all the name/value pairs associated to this installation using putProperties. | |
Returns the device's country on native apps, returns | |
Returns the device's currency on native apps, returns | |
Returns the device's locale. | |
Returns the device's IANA timezone. | |
Other | |
Returns a promise with the payload you provided in the popup editor. | |
Triggers the system location prompt. | |
Opens the AppStore or the Android Market rating form. Does nothing on websites. | |
Returns "iOS", "Android" or "Web" depending on the platform the device is running on. | |
Communicates with your website or native application |
Linking
openDeepLink
openDeepLink
This method behaves differently for apps and websites.
It dismisses the popup on all platforms.
Apps
openDeepLink
tries to open the provided URL inside the app.
This is ideal for URLs with a custom scheme (such as myapp://
), Android app links and iOS universal links. It falls back to the default browser of the device.
WonderPushPopupSDK.openDeepLink("myapp://some/path");
Websites
openDeepLink
simply replaces the _top
frame with the provided URL, opening the link in the same window. It is the equivalent of linking with a target="_top"
attribute, which is the preferred way of opening links in the same window.
<a href="https://www.example.com/" target="_top">link</a>
openExternalUrl
openExternalUrl
This method opens the provided URL in the device's default browser (apps) or in a new window (websites).
WonderPushPopupSDK.openExternalUrl("https://example.com/");
trackClick
trackClick
This method tracks a click in WonderPush, with the provided button label argument.
WonderPushPopupSDK.trackClick("Button label");
You can see the clicks on the detail page of your Popup:
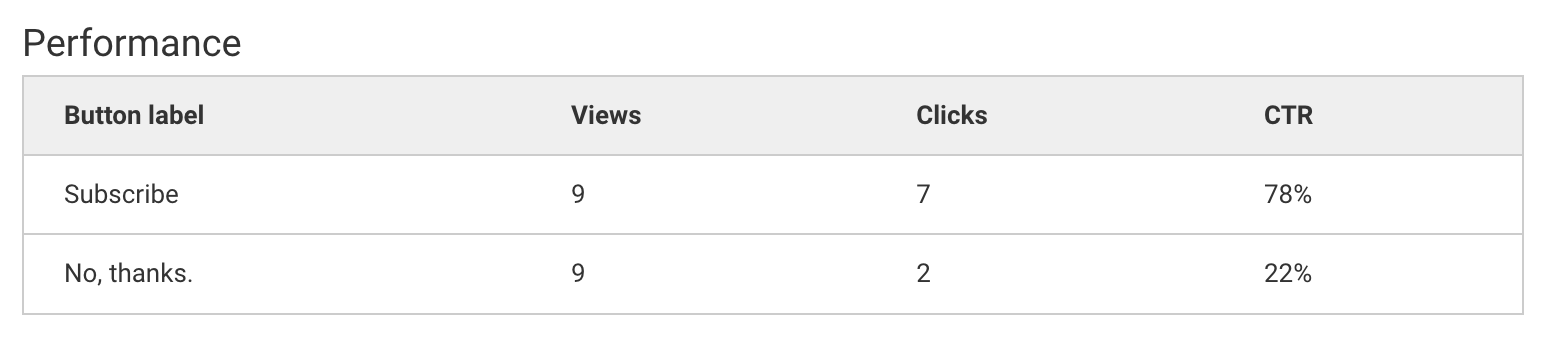
Dismissing
dismiss
dismiss
Dismisses the popup.
If your popup does not provide a close button or opens a link, users will have no means to close it
WonderPushPopupSDK.dismiss();
You can also dismiss the popup using the data-wonderpush-dismiss
data attribute:
<div class="close-button" data-wonderpush-dismiss>Close</div>
Subscribing users
subscribeToNotifications
subscribeToNotifications
Subscribes to push notifications.
<a href="#" onclick="WonderPushPopupSDK.subscribeToNotifications(); return false;">Subscribe to push notifications</a>
unsubscribeFromNotifications
unsubscribeFromNotifications
Unsubscribes from push notifications.
Please note that calling this function does not affect the push notification permission.
<a href="#" onclick="WonderPushPopupSDK.unsubscribeFromNotifications(); return false;">Unsubscribe from push notifications</a>
isSubscribedToNotifications
isSubscribedToNotifications
Returns a Promise
object that resolves to a boolean
indicating whether the user is subscribed to push notifications.
WonderPushPopupSDK.isSubscribedToNotifications().then(function (isSubscribed) {
console.log("is user subscribed to notifications: ", isSubscribed);
});
In this example, we call isSubscribedToNotifications
and log the result to the console once the Promise
resolves.
getUserId
getUserId
Returns a Promise resolving to the current user ID or null.
WonderPushPopupSDK.getUserId().then(function(userId) {
console.log("User ID is", userId);
});
In this example, we wait for the promise result and log it with console.log
.
getInstallationId
getInstallationId
Returns a Promise resolving to the current Installation ID, null
or undefined
.
WonderPushPopupSDK.getInstallationId().then(function(installationId) {
console.log('Installation ID is', installationId);
});
Segmentation
Segmentation functions allow you to mark installations so they can be added to segments and you can send them targeted notifications later.
There are many ways of performing segmentation:
Tags are like labels you can stick on users. Use tags to create segments in your dashboard and send targeted push notifications. Example: all users that have the "customer" tag.
Events have a date, a type
of your choice and attributes
. Use events to create segments in your dashboard and send targeted push notifications. Example: all users that purchased in the last hour is a typical event-based segment.
Installation properties represent traits of the user. That's a good place to store age, gender and other data traditionally used for segmentation. Create property-based segments in your dashboard. Example: all users above 18 is a typical property-based segment.
trackEvent
trackEvent
Tracks a custom event of your choice. E.g. purchase
.
Parameter | Type | Description |
---|---|---|
| String | Required The type of the event to track. Event names starting with |
| Object | Attributes associated with this event. See format of property names for detailed syntax. |
WonderPushPopupSDK.trackEvent('purchase', {
string_product: "Some product",
float_price: 1.99,
});
addTag
addTag
Adds one or more tags to the current installation.
WonderPushPopupSDK.addTag("customer");
WonderPushPopupSDK.addTag("economics", "sport", "politics");
removeTag
removeTag
Removes one or more tags from the current installation.
WonderPushPopupSDK.removeTag("customer");
WonderPushPopupSDK.removeTag("economics", "sport", "politics");
removeAllTags
removeAllTags
Removes all tags from the current installation.
hasTag
hasTag
Tests whether a given tag is attached to the current installation.
WonderPushPopupSDK.hasTag("customer").then(function(isCustomer) {
if (isCustomer) {
// Display paid features
}
});
getTags
getTags
Returns all the tags attached to the current installation as a promise resolving to an array of strings.
WonderPushPopupSDK.getTags().then(function(tags) {
// Work on the tags variable
});
getPropertyValue
getPropertyValue
Returns a Promise
that resolves to the value of a given property associated to the current installation.
If the property stores an array, only the first value is returned. This way you don't have to deal with potential arrays if that property is not supposed to hold one. Returns null
if the property is absent or has an empty array value.
Parameter | Type | Description |
---|---|---|
| String | The name of the property to read values from. |
WonderPushPopupSDK.getPropertyValue("string_lastname").then(function(lastName) {
// Use lastName
});
getPropertyValues
getPropertyValues
Returns a Promise
that resolves to an array of the values of a given property associated to the current installation.
If the property does not store an array, an array is returned nevertheless. This way you don't have to deal with potential scalar values if that property is supposed to hold an array. Returns an empty array instead of null
if the property is absent. Returns an array wrapping any scalar value held by the property.
Parameter | Type | Description |
---|---|---|
| String | The name of the property to read values from. |
WonderPushPopupSDK.getPropertyValues("string_favoritePlayers").then(function(favoritePlayers) {
// Use favoritePlayers array
});
addProperty
addProperty
Adds the value to a given property associated to the current installation.
The stored value is made an array if not already one. If the given value is an array, all its values are added. If a value is already present in the stored value, it won't be added.
Parameter | Type | Description |
---|---|---|
| String | The name of the property to add values to. |
| any, any[], any... | The value(s) to be added. Can be an array, or multiple arguments. |
// You can add a single value
WonderPushPopupSDK.addProperty("string_interests", "sport");
// You can add multiple values
WonderPushPopupSDK.addProperty("string_interests", "sport", "entertainment");
// You can add an array of values
WonderPushPopupSDK.addProperty("string_interests", ["sport", "entertainment"]);
removeProperty
removeProperty
Removes the value from a given property associated to the current installation.
The stored value is made an array if not already one. If the given value is an array, all its values are removed. If a value is present multiple times in the stored value, they will all be removed.
Parameter | Type | Description |
---|---|---|
| String | The name of the property to remove values from. |
| any, any[], any... | The value(s) to be removed. Can be an array, or multiple arguments. |
// You can remove a single value
WonderPushPopupSDK.removeProperty("string_interests", "sport");
// You can remove multiple values
WonderPushPopupSDK.removeProperty("string_interests", "sport", "entertainment");
// You can remove an array of values
WonderPushPopupSDK.removeProperty("string_interests", ["sport", "entertainment"]);
setProperty
setProperty
Sets the value to a given property associated to the current installation.
The previous value is replaced entirely. Setting undefined
or null
has the same effect as unsetProperty.
Parameter | Type | Description |
---|---|---|
| String | The name of the property to set. |
| any, any[] | The value to be set. Can be an array. |
// You can set a single value
WonderPushPopupSDK.setProperty("bool_isCustomer", true);
// You can remove a field using null or undefined
WonderPushPopupSDK.setProperty("int_age", null);
// You can set an array of values
WonderPushPopupSDK.setProperty("string_interests", ["sport", "entertainment"]);
unsetProperty
unsetProperty
Removes the value of a given property associated to the current installation.
The previous value is replaced with null
.
Parameter | Type | Description |
---|---|---|
| String | The name of the property to unset. |
WonderPushPopupSDK.unsetProperty("string_favoritePlayers");
putProperties
putProperties
Updates the properties of the current installation. Omitting a previously set property leaves it untouched. To remove a property, you must pass it explicitly with a value of null
.
Parameter | Type | Description |
---|---|---|
| Object | Properties to add or update. See format of property names for detailed syntax. |
// Puts the int_age property with a value of 34 in the installation
WonderPushPopupSDK.putProperties({
int_age: 34
});
getProperties
getProperties
Returns a Promise
that resolves to an Object containing the properties of the current installation.
WonderPushPopupSDK.getProperties().then(function(properties) {
console.log("Properties of the current installation are:", properties);
});
getCountry
getCountry
Returns a Promise with the device's country code on native apps, and undefined
on websites.
WonderPushPopupSDK.getCountry().then(function(country) {
// country will be undefined on websites
console.log('Country', country);
});
getCurrency
getCurrency
Returns a Promise with the device's ISO 4217 currency code on native apps, and undefined
on websites.
WonderPushPopupSDK.getCurrency().then(function(currency) {
// currency will be undefined on websites
console.log('Currency', currency);
});
getLocale
getLocale
Returns a Promise with the device's RFC 1766 locale.
WonderPushPopupSDK.getLocale().then(function(locale) {
console.log('Locale', locale);
});
getTimeZone
getTimeZone
Returns a Promise with the device's IANA timezone name.
WonderPushPopupSDK.getTimeZone().then(function(timeZone) {
console.log('Timezone', timeZone);
});
Other
getPayload
getPayload
Returns a promise with the JSON payload provided in the popup editor of your dashboard.

The payload can be used to pass data from the dashboard to your popup. You get the payload like this:
WonderPushPopupSDK.getPayload().then(function(payload) {
console.log('The payload is', payload);
});
triggerLocationPrompt
triggerLocationPrompt
Triggers the location permission prompt on iOS, Android and web browsers.
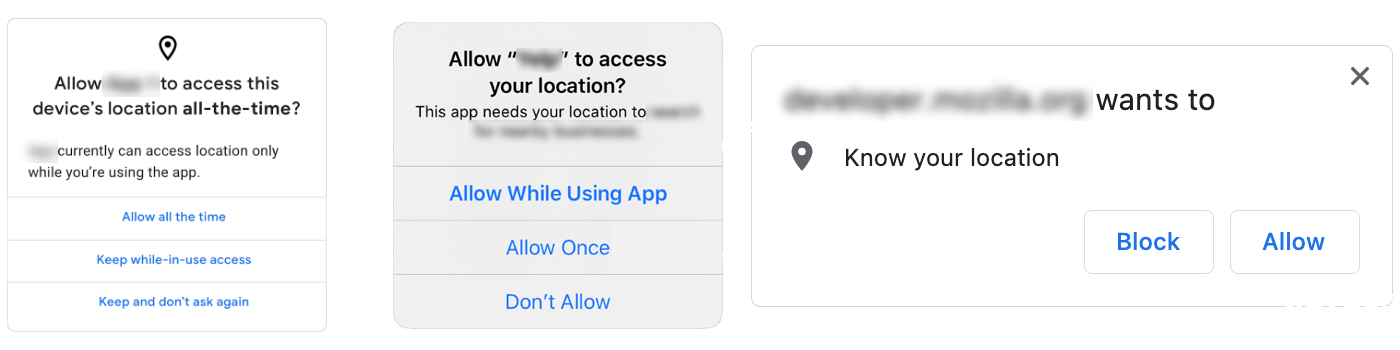
Images of location prompts on Android, iOS and Google Chrome
The prompt can be triggered like this:
WonderPushPopupSDK.triggerLocationPrompt();
iOS location prompts
Native iOS apps have to provide an explanation for the permission request in their Info.plist
file.
Apps requiring location data at all time provide the NSLocationAlwaysUsageDescription
key and call the requestAlwaysAuthorization
method of the CLLocationManager
.
Apps requiring location data when in-use provide the NSLocationWhenInUseUsageDescription
key and call the requestWhenInUseAuthorization
method of the CLLocationManager
.
WonderPush will look at existing Info.plist
entries in that order:
- if
requestAlwaysAuthorization
is provided, WonderPush callsrequestAlwaysAuthorization
- otherwise, if
NSLocationWhenInUseUsageDescription
is provided, WonderPush callsrequestWhenInUseAuthorization
- otherwise WonderPush does nothing
Android location prompts
Native Android apps can request coarse or fine location access. In order to achieve this, their Manifest.xml
file must contain the relevant <uses-permission>
tags.
WonderPush will request fine location access for apps whose Manifest declares the android.permission.ACCESS_FINE_LOCATION
permission.
Otherwise, WonderPush will request coarse location access for apps whose Manifest declares the android.permission.ACCESS_COARSE_LOCATION
permission.
For apps that contain none of the above permission declarations in their Manifest, WonderPush will do nothing.
openAppRating
openAppRating
This method opens the AppStore rating form on iOS native apps, the Android Market rating form on Android apps, and does nothing on websites.
WonderPushPopupSDK.openAppRating();
getDevicePlatform
getDevicePlatform
This method allows you to adapt the behavior of your popups based on the platform the device is running on. Native iOS apps will get the value iOS
, native Android apps will get the value Android
and websites will get the value Web
.
WonderPushPopupSDK.getDevicePlatform().then(function(devicePlatform) {
switch (devicePlatform) {
case "iOS":
// Native iOS app
break;
case "Android":
// Native Android app
break;
case "Web":
// Website
break;
}
});
callMethod
callMethod
Use this method to communicate with your app or website. You can transmit a single string argument.
Parameter | Type | Description |
---|---|---|
methodName | string | The name of the method you wish to call on your website or native app |
methodArg | string | A string that will be passed along. Optional. |
WonderPushPopupSDK.callMethod("someMethod", "someArgument");
Now, in the code of your website, or in the native code of your mobile app, you can intercept the result like this for a method called someMethod
:
window.addEventListener('WonderPushEvent', function(ev) {
if (ev.detail.name === 'method') {
var method = ev.detail.method;
var arg = ev.detail.arg;
if (method === 'someMethod') {
// Do something useful with arg
}
}
});
// Put the following call in the [application:didFinishLaunchingWithOptions:] method of your AppDelegate
// Here is how to register the callback named "example"
NotificationCenter.default.addObserver(forName: NSNotification.Name(rawValue: WP_NOTIFICATION_REGISTERED_CALLBACK), object: nil, queue: nil, using: {notification in
let method = notification.userInfo?[WP_REGISTERED_CALLBACK_METHOD_KEY] as? String
let arg = notification.userInfo?[WP_REGISTERED_CALLBACK_PARAMETER_KEY] as? String
// Do something useful here
})
// Put the following call in the [application:didFinishLaunchingWithOptions:] method of your AppDelegate
// Here is how to register the callback named "example"
[[NSNotificationCenter defaultCenter] addObserverForName:WP_NOTIFICATION_REGISTERED_CALLBACK object:nil queue:nil usingBlock:^(NSNotification *note) {
NSString *method = [note.userInfo objectForKey:WP_REGISTERED_CALLBACK_METHOD_KEY];
NSString *arg = [note.userInfo objectForKey:WP_REGISTERED_CALLBACK_PARAMETER_KEY];
// Do something useful here
}];
// Put the following call before you initialize the SDK, in your Application class
// Here is how to register the callback named "example"
IntentFilter exampleMethodIntentFilter = new IntentFilter();
exampleMethodIntentFilter.addAction(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_ACTION);
exampleMethodIntentFilter.addDataScheme(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_SCHEME);
exampleMethodIntentFilter.addDataAuthority(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_AUTHORITY, null);
exampleMethodIntentFilter.addDataPath("/someMethod", PatternMatcher.PATTERN_LITERAL); // Note: prepend a / to the actual method name
LocalBroadcastManager.getInstance(this).registerReceiver(new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String arg = intent.getStringExtra(WonderPush.INTENT_NOTIFICATION_BUTTON_ACTION_METHOD_EXTRA_ARG);
// Do something useful here
}
}, exampleMethodIntentFilter);
document.addEventListener('wonderpush.registeredCallback', function(event) {
console.log("Callback called for method", event.method, "with arg", event.arg);
}, false);
This feature is not supported yet on React Native.
We'd like to hear about your use case.
This feature is not supported yet on Flutter.
We'd like to hear about your use case.
Updated 19 days ago