React Native Push Notifications
How to set up WonderPush push notifications on React Native cross-platform apps
Install push notifications in React Native apps in 4 steps.
Setting up push notifications for your React Native app is easy. Push notifications are the ideal solution to re-engage users and bring them back to your app.
Estimated setup time: 15 minutes.
Prerequisites
You'll need:
- NodeJS, v8.3 or ulterior
- React Native
react-native >= 0.61.5
- For Android:
- A device or emulator with Google Play services installed and up-to-date.
- A Firebase account.
- Android Studio.
- For iOS:
- XCode
- An iOS device such as an iPhone or an iPad
- An iOS push credentials
To upgrade to the latest version of our SDK, follow these instructions.
Expo usersIf you're using expo, you'll need to "eject" your app. Simply go into your project's folder and run:
expo eject
If you haven't already, sign up for a free account on wonderpush.com.
Step 1. Create your project
Click on New Project:
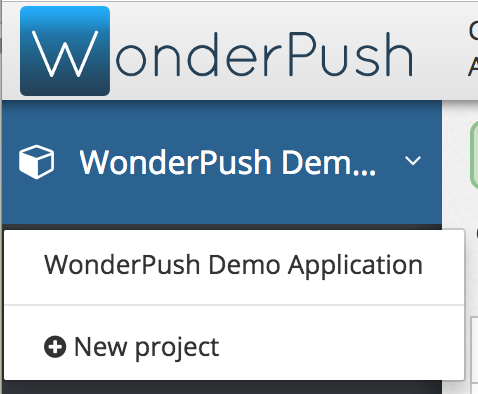
Choose a name for your project and select Android and iOS as platforms then click Create:
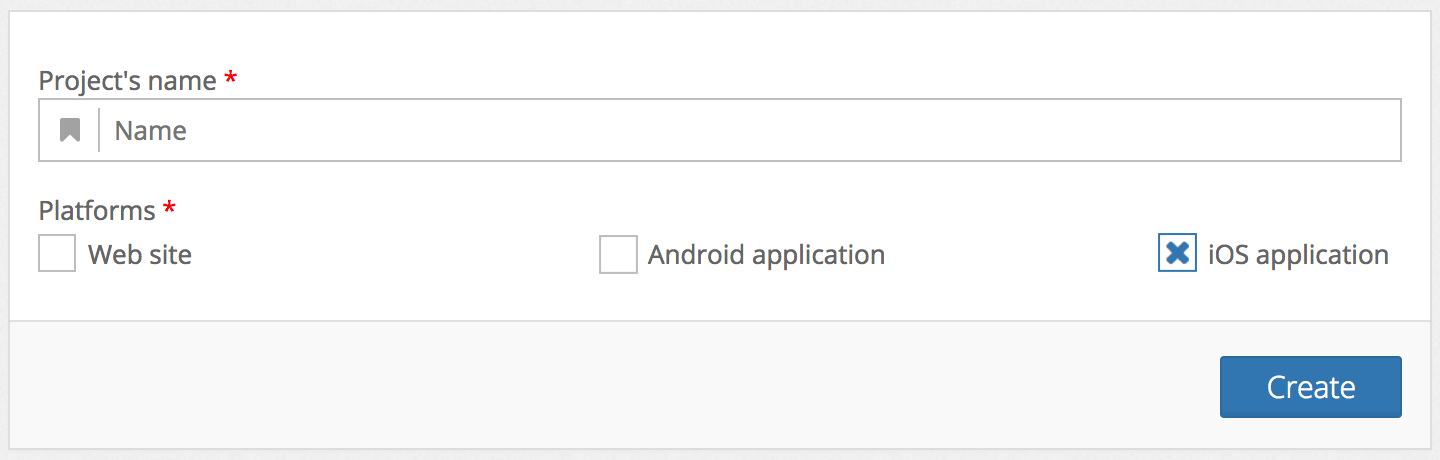
Already have a project?Just add the Android or iOS platforms to any existing project by going to Settings, selecting the first tab named after your project and clicking Edit. You'll be presented a form that lets you add a platform.
Step 2. Setup your push credentials
Android - Add your Firebase credentials
Follow the steps outlined in the Firebase / Filling the Firebase credentials in the WonderPush dashboard article.
Keep note of your Project number, also known as the Sender ID, as you will need in the below steps.
iOS - Upload your push credentials
Get your Apple key with the Apple Push Notifications service (APNs) service enabled. You can list your existing keys here. Simply check the Enabled Services and note the Key ID as well as the Team ID displayed below your name in the top-right corner.
If you don't have one, here's how to create one. In a few words, head to the Apple developer website, go to Accounts, then click on Keys in the Certificates, Identifiers & Profiles column, and click the + button on the top left to add a new key.
Check Apple Push Notifications service (APNs), then click Continue:
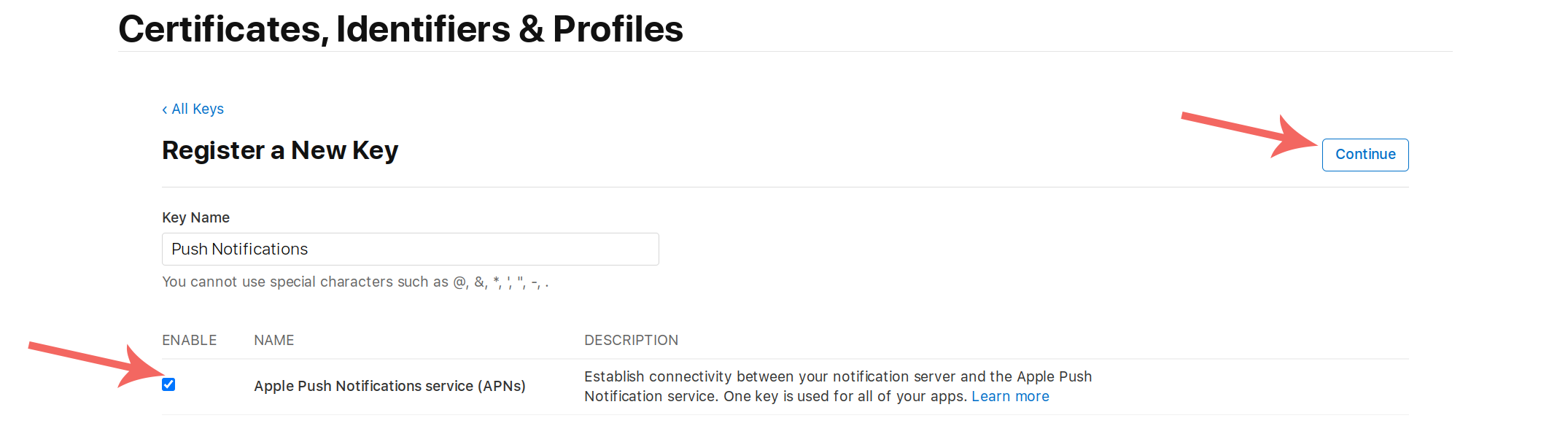
If instead you see the following red message, this means that you should instead reuse an existing key. As Apple will not let you download the key again after its registration, you will need to use for the .p8
file that you downloaded at that time.

How should Apple keys be managed for the Apple Push Notifications service (APNs)Apple imposes a maximum of 2 keys simultaneously valid for the Apple Push Notifications service (APNs). Such keys are valid for all applications (Bundle ID or App ID) on your account (Team ID).
Limiting to 2 keys enables you to have one key used for all your push notification needs across all your WonderPush projects (you'll need to upload the same key for each of your projects) or even push providers, and perform a rolling replacement of your key with no downtime.
You would create a second key, temporarily having 2 valid keys at a time, replace every WonderPush project or services that need to send push notifications, and finally revoke the previous key, leaving you again with a single existing key.
In the next screen click Register.
In the next screen, note your Key ID, and your Team ID in the top right corner.
Then click Download. Please do keep this.p8
file in a secure location (see the above callout about key management.)
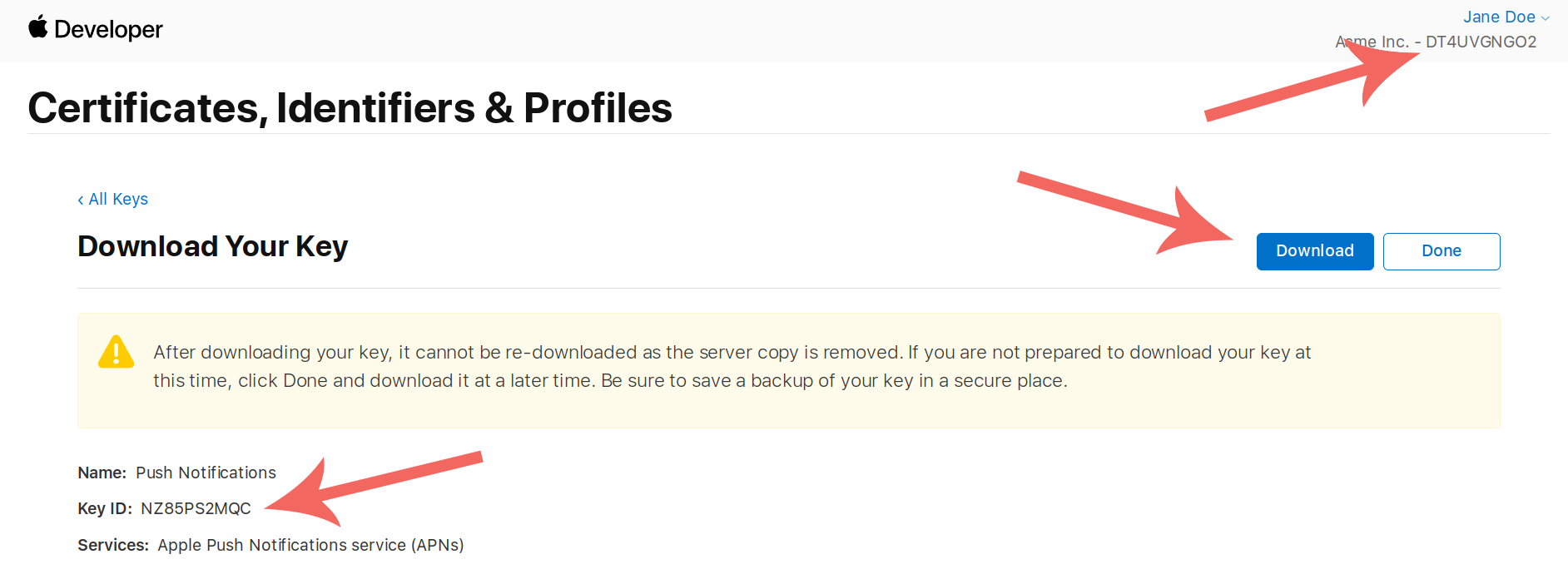
You will then need to know your Bundle ID:
- Open your project in Xcode
- Click on your project in the file browser on the left
- Click on your app target in the list
- Open the General tab
- Your Bundle identifier (aka Bundle ID) is in the Identity section
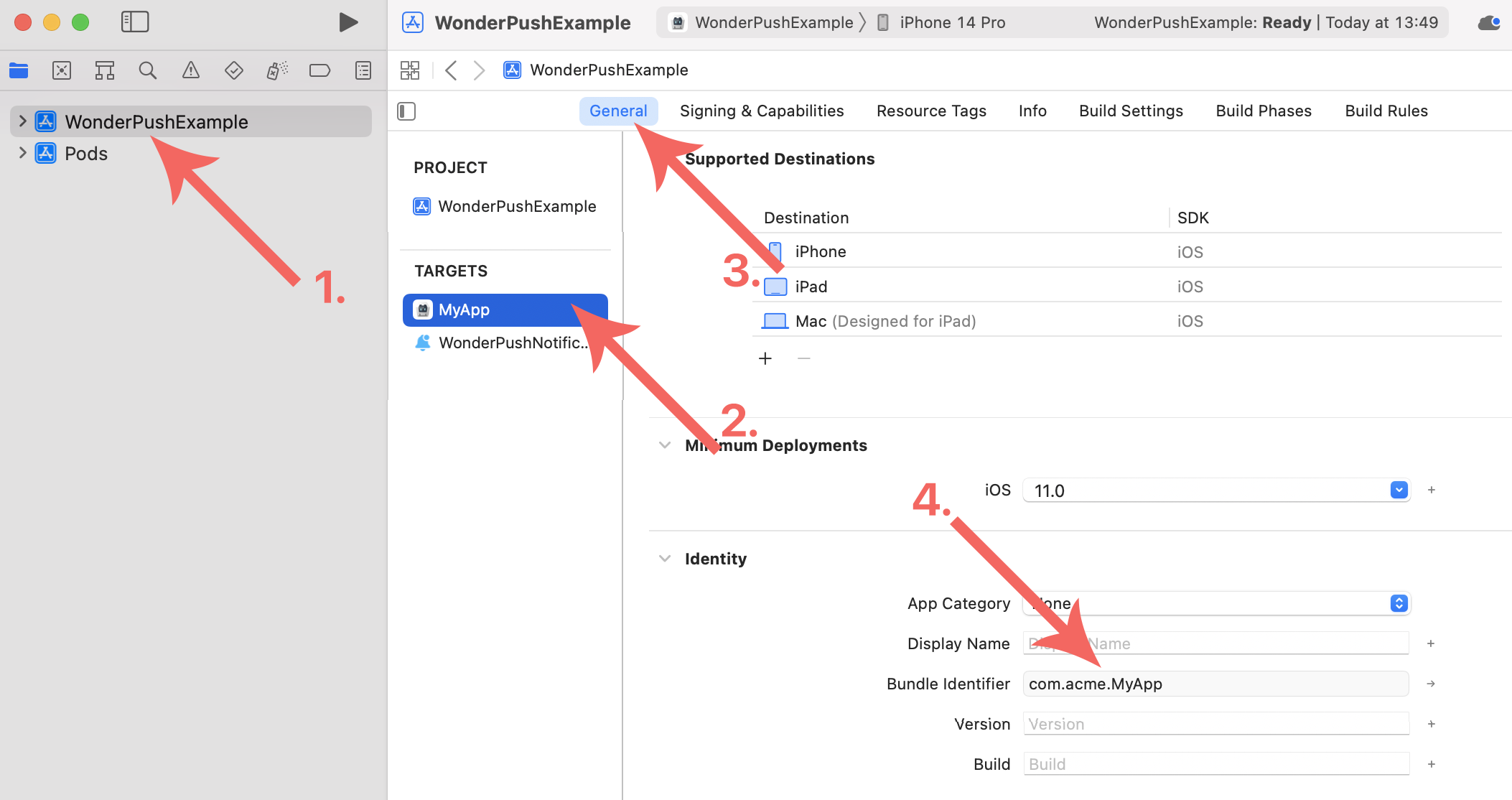
Now go to Settings / Platforms, click the iOS application section title and fill drop your .p8
file:
- Fill the Key ID, Team ID and Bundle ID if necessary. Check their values if they are pre-filled.
- Click Save.
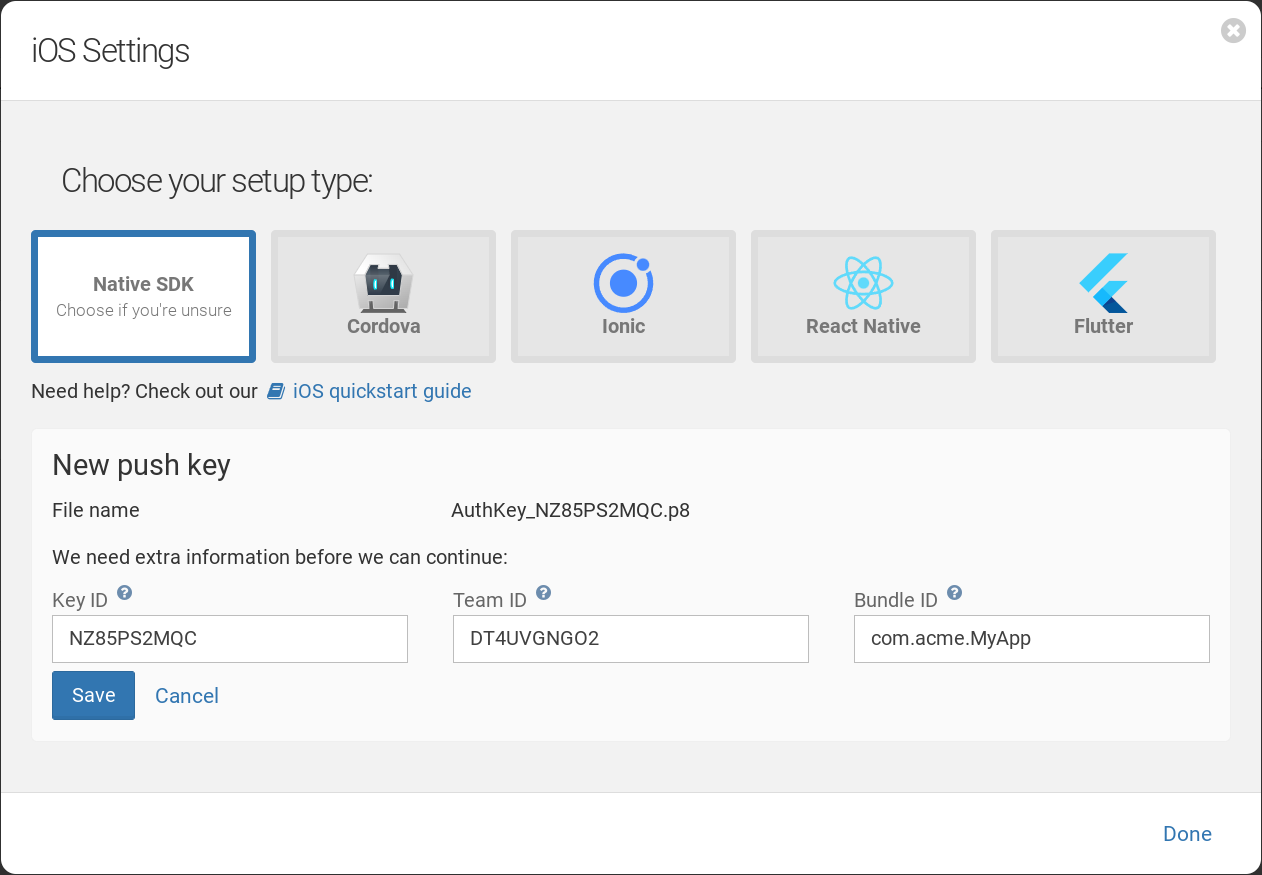
Step 3. Add the SDK
Create your React Native application if you haven't already, see the official React Native guide and click React Native CLI Quickstart, for more details.
Add the WonderPush React Native SDK:
npm install react-native-wonderpush --save
npm install react-native-wonderpush-fcm --save
If you're using a version of react-native earlier than 8, link the WonderPush React Native SDK:
react-native link react-native-wonderpush
react-native link react-native-wonderpush-fcm
Huawei mobiles supportBecause Huawei mobiles no longer ship with Google Play Services necessary for using Firebase Cloud Messaging, you will need to follow the Huawei mobiles support guide to support them.
iOS - Add push entitlement, background mode and service extension
Open the xcworkspace
file of your project in Xcode:
open ios/*.xcworkspace
Add device capabilities
Select your app's main target and, under the Signing and Capabilities tab, click “+ Capability”
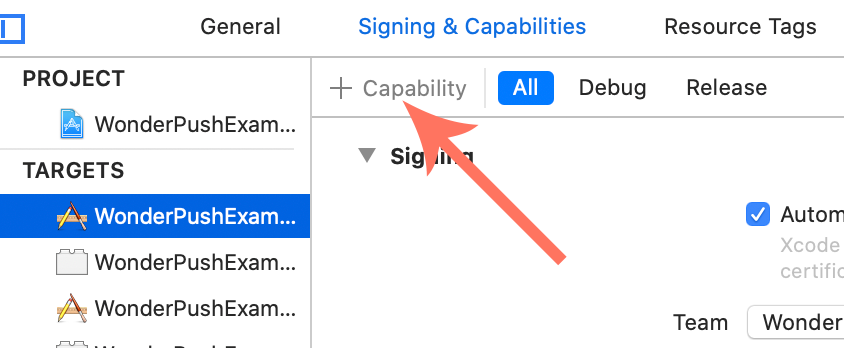
Add the following capabilities, one by one:
- Background Modes,
- Push Notifications.
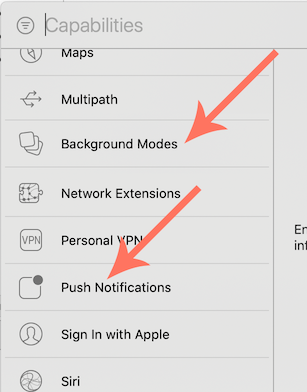
Make sure the Remote notifications background mode is checked:
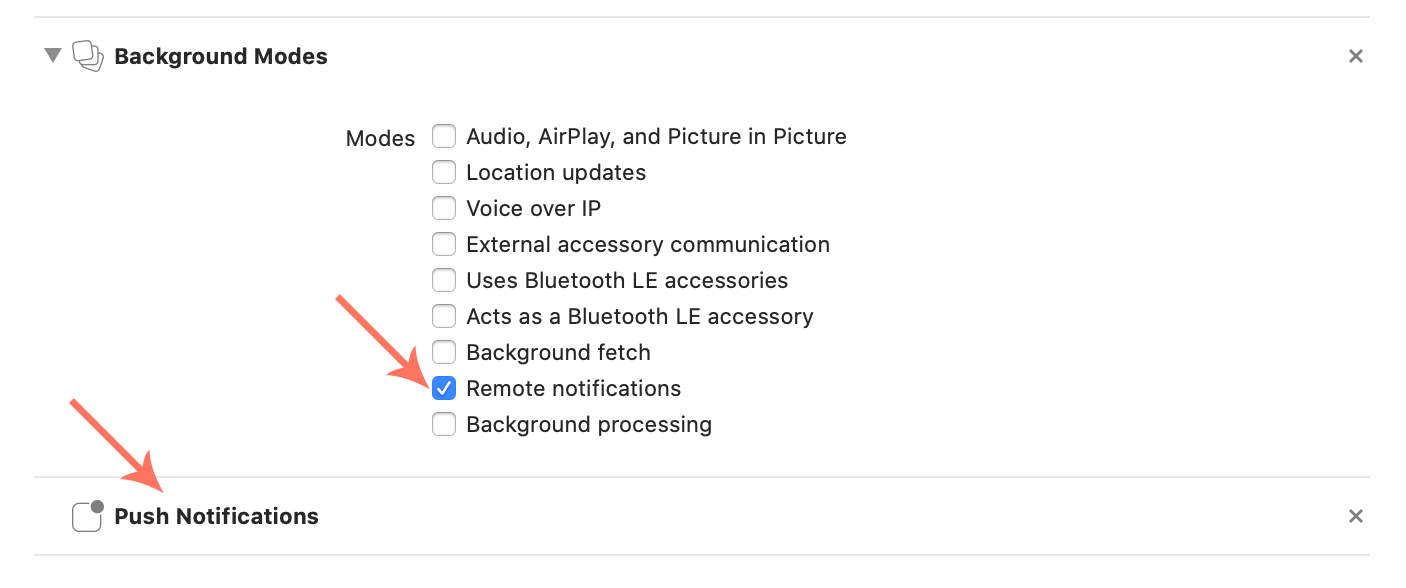
Create the notification service extension
In Xcode, select File / New / Target..., and choose Notification Service Extension:
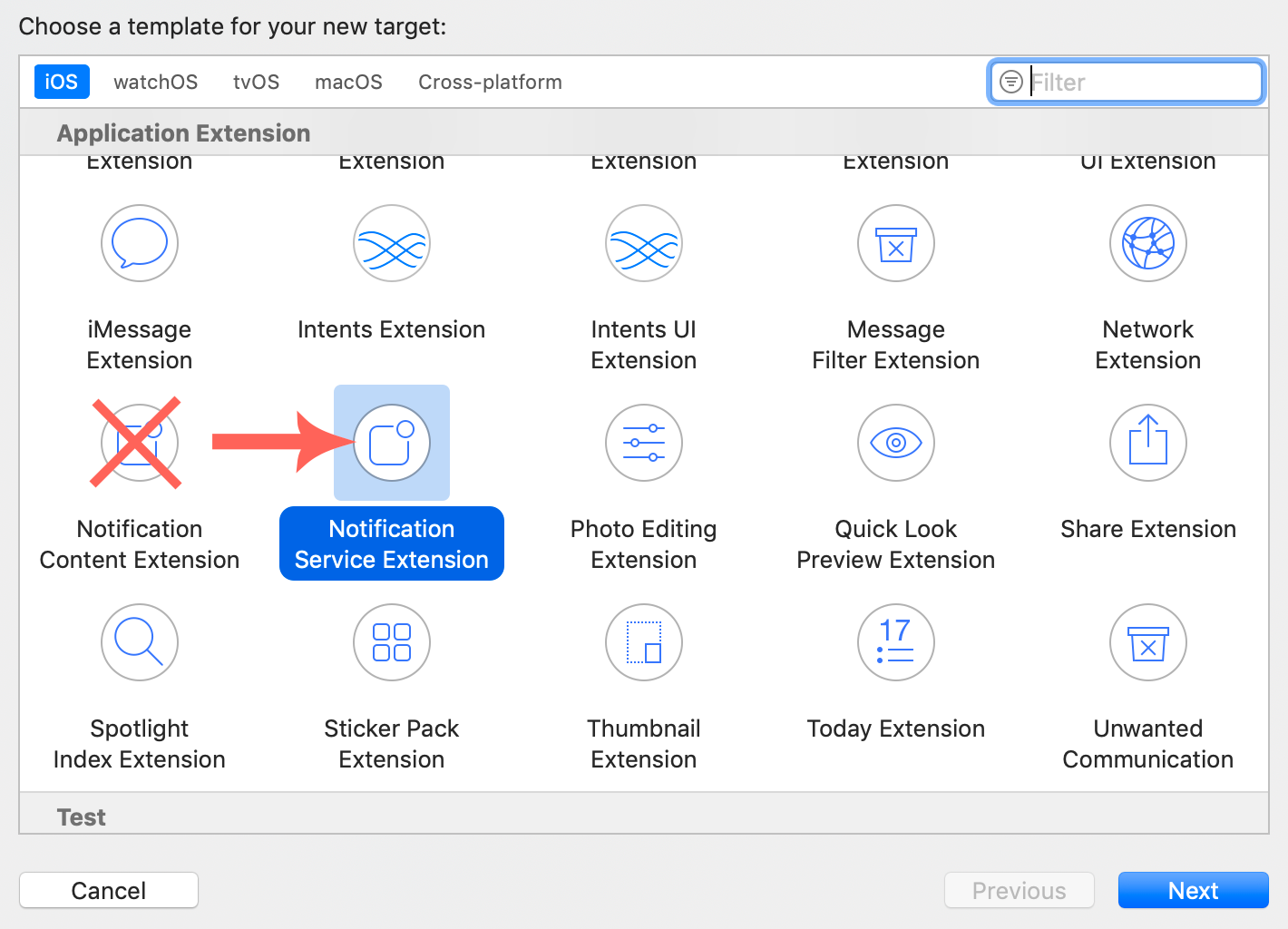
Enter WonderPushNotificationServiceExtension
as the name for your new target:
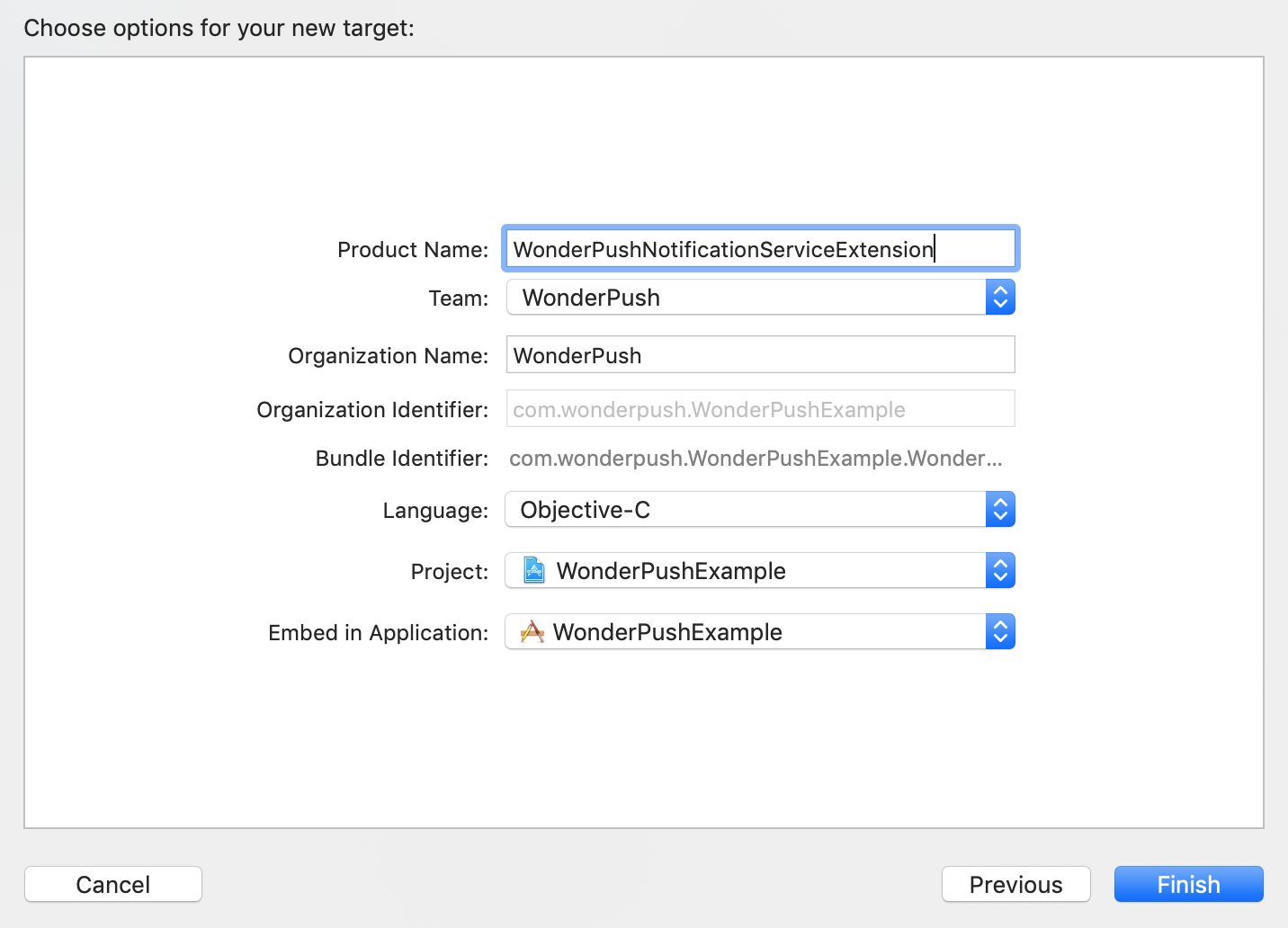
When Xcode prompts you to activate the new scheme, answer Cancel to keep Xcode building and debugging your app instead of the extension:
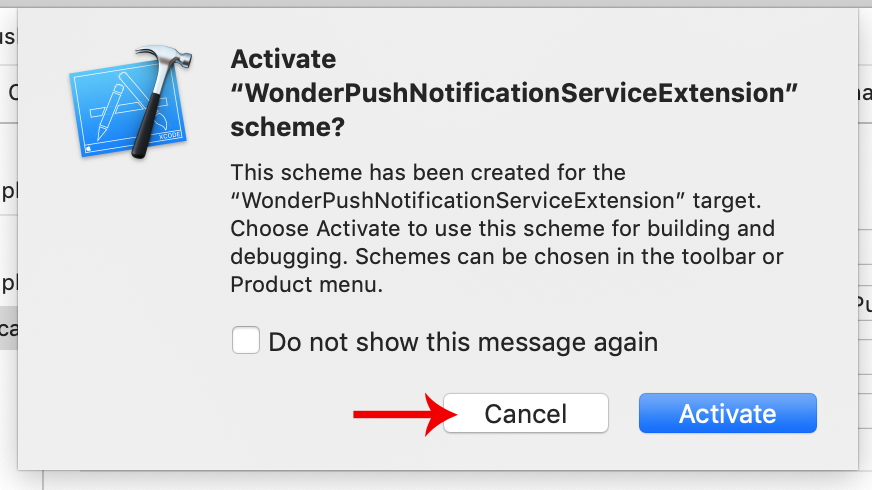
If you activate by mistake, switch back to your app's scheme from the dropdown menu located next to the play button.
Set the Deployment Target of your Notification Service Extension to the same value as your main application target:
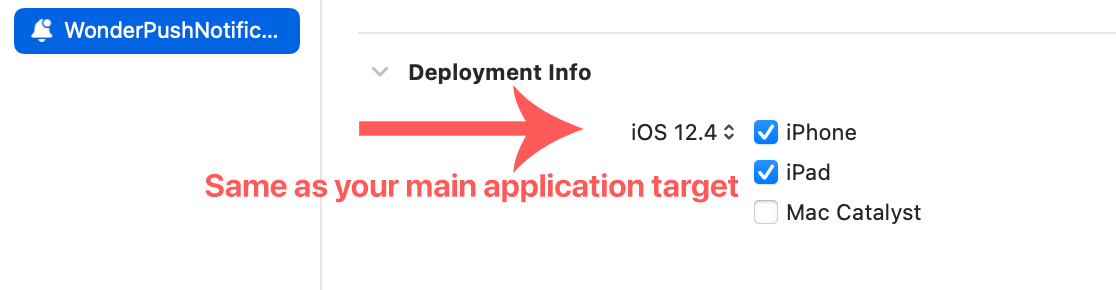
Make sure to use Automatic Signing for the extension target:
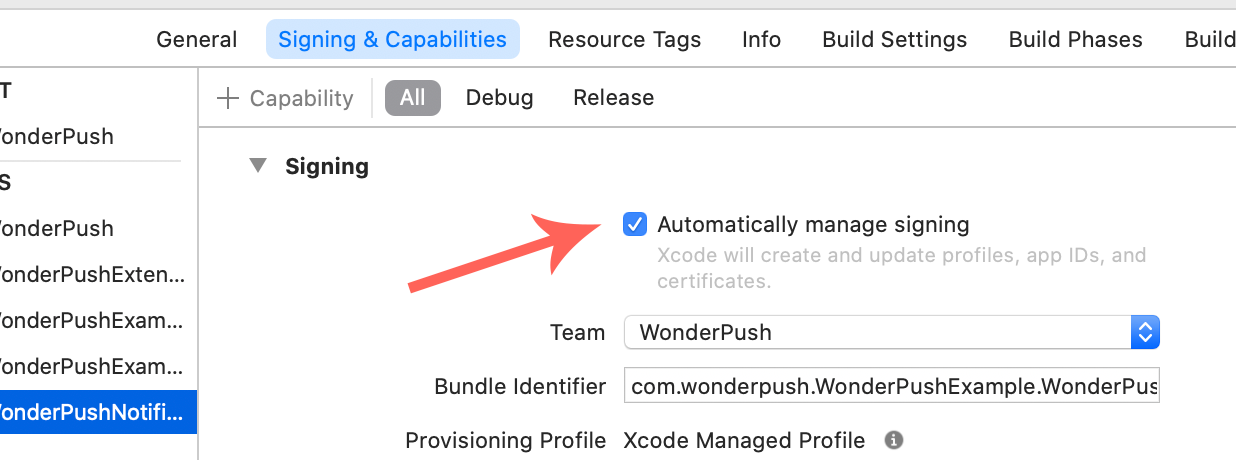
If you're getting the following error, make sure to set up Automatic Signing for the extension:
Provisioning profile "XXX" has app ID "YYY", which does not match the bundle ID "YYY.WonderPushNotificationServiceExtension".
Edit the file ios/Podfile
and add the following at the bottom:
target 'WonderPushNotificationServiceExtension' do
platform :ios, '10.0'
# Pods for WonderPushNotificationServiceExtension
pod 'WonderPushExtension', '~> 4.0'
end
cd ios
pod install
open *.xcworkspace
If you get the following message:
CocoaPods could not find compatible versions for pod "WonderPush"
, just runpod update
andpod install
again.
Step 4. Add required code
Take note of your Client ID and Client Secret from the Platforms tab of the Settings page:
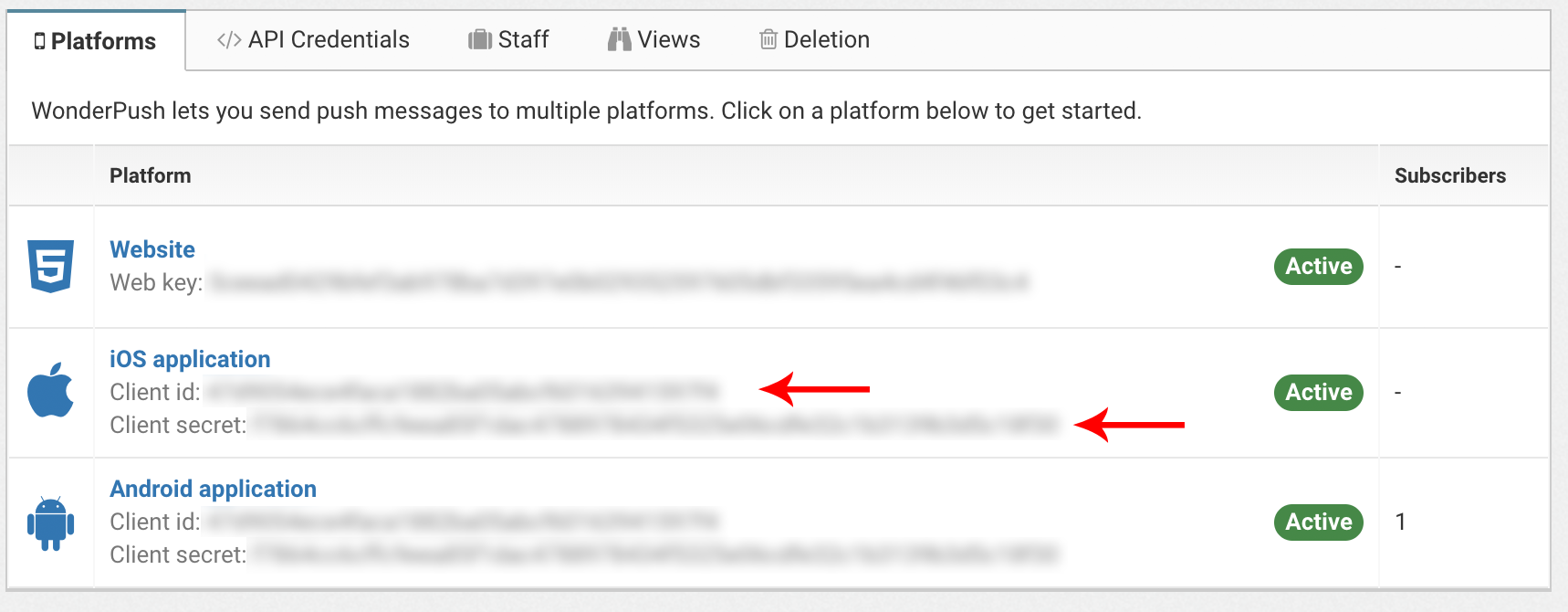
Let's add the code that will prompt users to subscribe to push notifications. We recommend you place this code in a more suitable place in the user journey, but we'll do it at launch time for now. In your App
class:
import React from 'react';
// Import WonderPush
import WonderPush from 'react-native-wonderpush';
const App: () => React$Node = () => {
// Prompts users to subscribe to push notifications.
// Move this in a more suitable place in the user journey.
WonderPush.subscribeToNotifications();
return (
// ...
);
};
export default App;
import WonderPush from 'react-native-wonderpush';
export default class App extends Component {
constructor() {
super();
// Prompts users to subscribe to push notifications.
// Move this in a more suitable place in the user journey.
WonderPush.subscribeToNotifications();
}
render() {
// ...
}
}
For Android
Open the Android Studio and configure the WonderPush Android SDK from your android/app/build.gradle
file:
android {
defaultConfig {
// Note that it's important to keep the double quotes as part of the third argument
// as this represents a string in Java code
buildConfigField 'String', 'WONDERPUSH_CLIENT_ID', '"YOUR_CLIENT_ID"'
buildConfigField 'String', 'WONDERPUSH_CLIENT_SECRET', '"YOUR_CLIENT_SECRET"'
buildConfigField 'String', 'WONDERPUSH_SENDER_ID', '"YOUR_SENDER_ID"'
buildFeatures {
buildConfig = true
}
}
}
Replace YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with the appropriate values you find in the Settings page.
Replace YOUR_SENDER_ID
with the Firebase Sender ID from step 2.
WonderPush needs Android SDK 21, so you need to ensure your project does as well. Open android/build.gradle
, find the line containing minSdkVersion
and ensure it looks like this:
minSdkVersion = 21
Controlling the Android 13+ permission prompt timingIn order to control when the permission prompt is triggered when running on Android 13 devices, you will need to update your
android/build.gradle
to use:buildscript { ext { compileSdkVersion = 33 targetSdkVersion = 33 } }
If you do not do so, the user will be prompted for permission right at application launch.
Click Sync now in the banner that showed up in your editor, or click the Sync project with Gradle files button in the toolbar.
Then build your project.
For iOS
Open your AppDelegate
and add the following, adapting YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with the values you've noted above:
import WonderPush
func application(_ application: UIApplication, willFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
WonderPush.setClientId("YOUR_CLIENT_ID", secret: "YOUR_CLIENT_SECRET")
WonderPush.setupDelegate(for: application)
WonderPush.setupDelegateForUserNotificationCenter()
return true
}
#import <WonderPush/WonderPush.h>
- (BOOL)application:(UIApplication *)application willFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[WonderPush setClientId:@"YOUR_CLIENT_ID" secret:@"YOUR_CLIENT_SECRET"];
[WonderPush setupDelegateForApplication:application];
[WonderPush setupDelegateForUserNotificationCenter];
return YES;
}
Finally, we'll modify the code of the Notification Service Extension you created in Step 3. Replace the whole contents of NotificationService.swift
or (NotificationService.h
and NotificationService.m
for Objective-C) with:
import WonderPushExtension
class NotificationService: WPNotificationServiceExtension {
override class func clientId() -> String {
return "YOUR_CLIENT_ID"
}
override class func clientSecret() -> String {
return "YOUR_CLIENT_SECRET"
}
}
#import <WonderPushExtension/WonderPushExtension.h>
@interface NotificationService : WPNotificationServiceExtension
@end
#import "NotificationService.h"
@implementation NotificationService
+ (NSString *)clientId {
return @"YOUR_CLIENT_ID";
}
+ (NSString *)clientSecret {
return @"YOUR_CLIENT_SECRET";
}
@end
Adapt YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with the values you've noted above, the same you just gave to your AppDelegate
.
Clean your project by choosing Product / Clean Build Folder
in Xcode or by running:
cd ios
xcodebuild clean
Receive your first push!
Use XCode or Android Studio to build and run your application and get the permission prompt you've configured:\
Wait a couple of minutes and receive the default welcome notification:
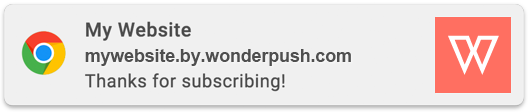
Setup deep links
If you are using deep links (links of the form myapp://some/path
), follow this guide.
Congratulations, you're done!
Updated 28 days ago