Properties
Properties are a simple and powerful way to store user-data
What are properties?
Properties are key/value pairs you can use to describe a user. Properties work the same way Tags do, but they allow you to store more sophisticated data like numbers, booleans, strings.
Properties can be used to target users in your Segments, as well as automate the sending of push notifications.
They can also be used to personalize push notifications (like calling the user by his/her name in a notification).
Common examples of properties are:
- the age of the user,
- the user's full name,
- a customer's all-time revenue.
Setting properties
Adding properties requires very little coding. Just call the putProperties
method of our SDK:
window.WonderPush = window.WonderPush || [];
WonderPush.push(['putProperties', {
"string_name": "John Doe",
"int_age": 32
}]);
JSONObject properties = new JSONObject();
properties.put("string_name", "John Doe");
properties.put("int_age", 32);
WonderPush.putProperties(properties);
WonderPush.putProperties([
"string_name": "John Doe",
"int_age": 32
]);
[WonderPush putProperties:@{
@"string_name": @"John Doe",
@"int_age": @32
}];
WonderPush.putProperties({
"string_name": "John Doe",
"int_age": 32
});
WonderPush.putProperties({
"string_name": "John Doe",
"int_age": 32
});
WonderPush.putProperties({
string_name: "John Doe",
int_age: 32
});
Property names
Property names are subject to the following rules:
- they are prefixed according to their value type. For example, string properties have names that start with
string_
. See available prefixes below. - they must only contains letters, numbers and the underscore
_
character.
Available prefixes are:
Prefix | Usage |
---|---|
byte_ | An integer value between -128 and 127, inclusive. |
short_ | An integer value between -32,768 and 32,767, inclusive |
int_ | An integer value between -231 and 231-1, inclusive. |
long_ | An integer value between -263 and 263-1, inclusive. |
float_ | An single precision floating point number. |
double_ | An double precision floating point number. |
bool_ | A boolean value, either true of false . |
string_ | A string value. Values above 128 characters will be ignored. |
date_ | A UTC timestamp in milliseconds, a Date object, or a string in the following format: YYYY[-MM[-DD]][T[HH[:MM[:SS[.SSS]]]][Z|+HH[:MM[:SS[.SSS]]]]] Here are valid examples: 2019 , 2019-12 , 2019-12-31 , 2019-12-31T23 , 2019-12-31T23:59 , 2019-12-31T23:59:59 , 2019-12-31T23:59:59.999 .Here are valid offsets you can also append: Z for UTC, -06 , +02:00 . In the absence of an offset, the date is taken as UTC. Don’t forget to write T before the offset if you gave no time-part.Unless explicitly mentioned, any missing part is taken as January 1st for date-part and midnight for the time-part. |
geoloc_ | A geographic localisation given as latitude and longitude, or geohash. Value as an object: {"lat": 48.85837, "lon": 2.294481} .Value as a string: "48.85837,2.294481" , latitude first, longitude next.Value as an array: [2.294481, 48.85837] , longitude first, latitude next. Note the reversed order, conforming to GeoJSON.Value as a geohash: "u09tunqu9k31" . See the Geohash article on Wikipedia. |
Setting properties in response to a user click on a notification
Just head over to the On click actions section of the Create/edit notification page and choose Add action and Set property for the notification itself or for any button.
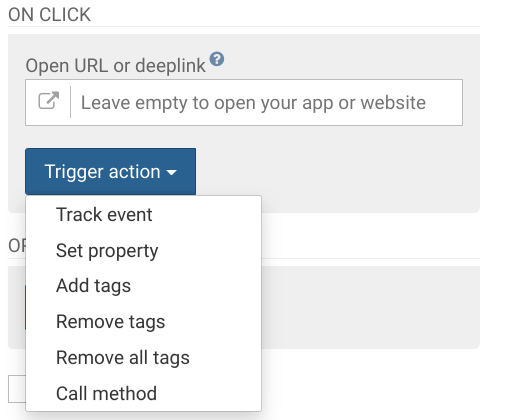
Setting properties on the server side
This is easily achieved using the PATCH /installations/{installationId} call of our REST API. Properties added this way will not be taken into account for in-app messaging segmentation.
Creating segments based on properties
You can add property-related criteria to segments by choosing Installation property in the segmentation form. See the Segments guide for more on creating segments.
In this example, only users with an int_age
property greater than or equal to 32 will be in the audience.
Triggering a push notification when a property changes
You can trigger a push notification automatically whenever a property reaches a certain value, after a certain delay, directly from the push notification editor. This is explained in detail in the Push notification editor documentation.
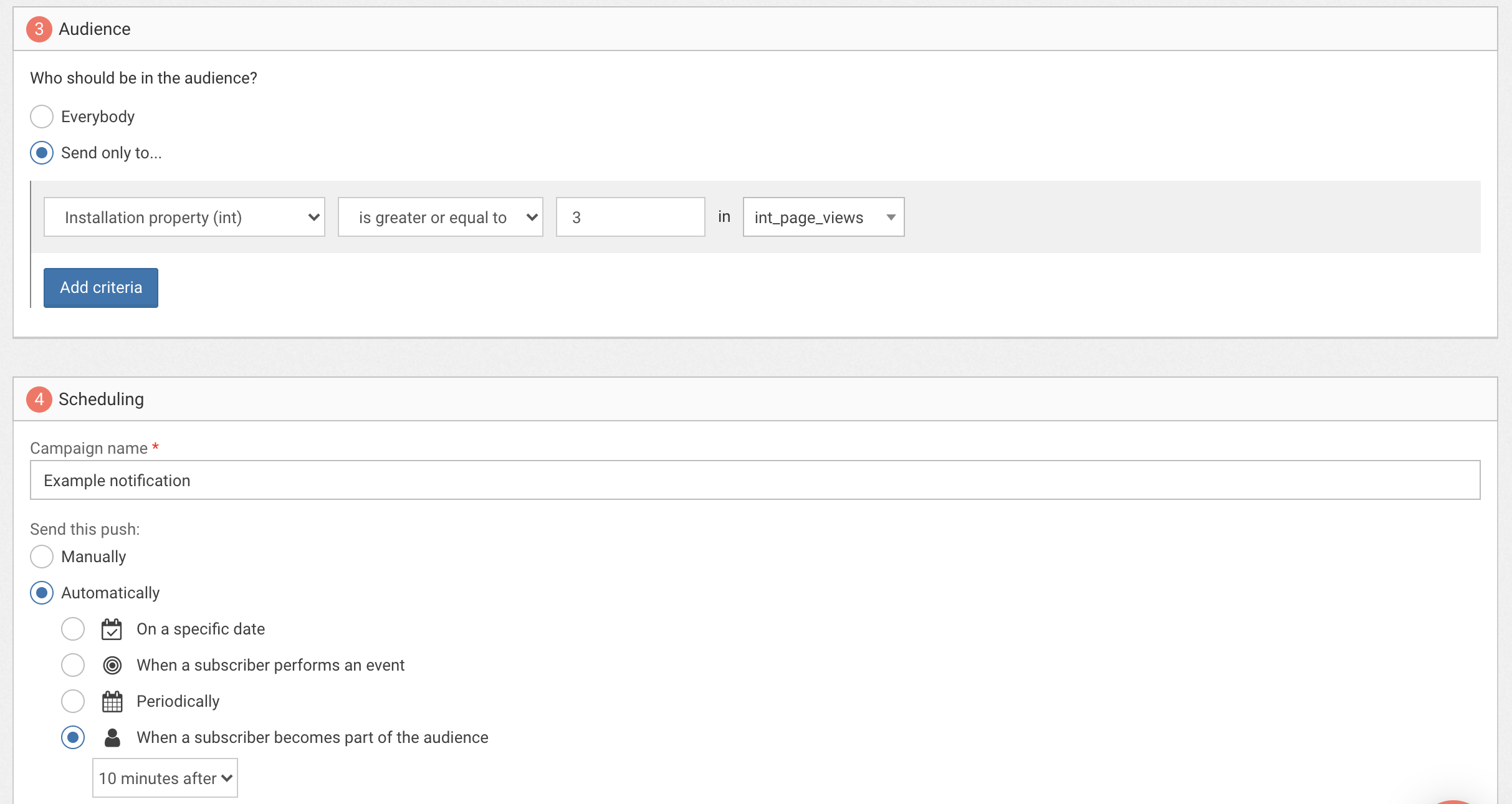
Example of an automated notification scheduled to be sent 10 minutes after the user reaches 3 page views.
Built-in properties
WonderPush SDKs automatically collects some properties from user devices such as Operating system, Timezone, etc. You can use these properties just like any other.
Updated about 1 year ago