Supporting iOS Live Activities
How to create Live Activities in your app and update them using WonderPush
What are iOS Live Activities
Starting from iOS 16.1 release on October 24th 2022, developers can create a new type of dynamic content displayed to the user when he is not in your application, prominently visible in the lockscreen and in the dynamic island for compatible phones like the iPhone 14 Pro. Live Activities, as they are called, are a new type of rich push notifications displaying a custom UI, and updatable in the background.
A few particularities, compared to push notifications:
- You do not need to prompt the user for permission prior to displaying a Live Activity. Instead, the first two Live Activities your application will display contain two additional buttons to let the user accept or decline seeing more Live Activities.
- You can only start a new Live Activity when your application is in the foreground, typically after a user gesture.
- Live Activities can be updated and ended in the background, using WonderPush API.
- A Live Activity is persistent in the lockscreen and the dynamic island for compatible phones like the iPhone 14 Pro.
- Like push notifications, the user can however dismiss a Live Activity at any time by swiping it to the left.
- Live Activities can last for up to 8 hours before being ended. They can be updated during this period. They can then linger on screen for a configurable duration of up to 4 hours before being dismissed by the system.
- A Live Activity has a completely custom UI that you need to develop using SwiftUI.
- A single application can display up to 5 simultaneous Live Activities.
- You need to have a P8 key configured to send push notifications, using a P12 certificate is not supported.
Read more about Live Activities: Human interface guidelines for Live Activities, How to create a Live Activity in your application
Example of Live Activities
Here are a few ways a Live Activity can be displayed:
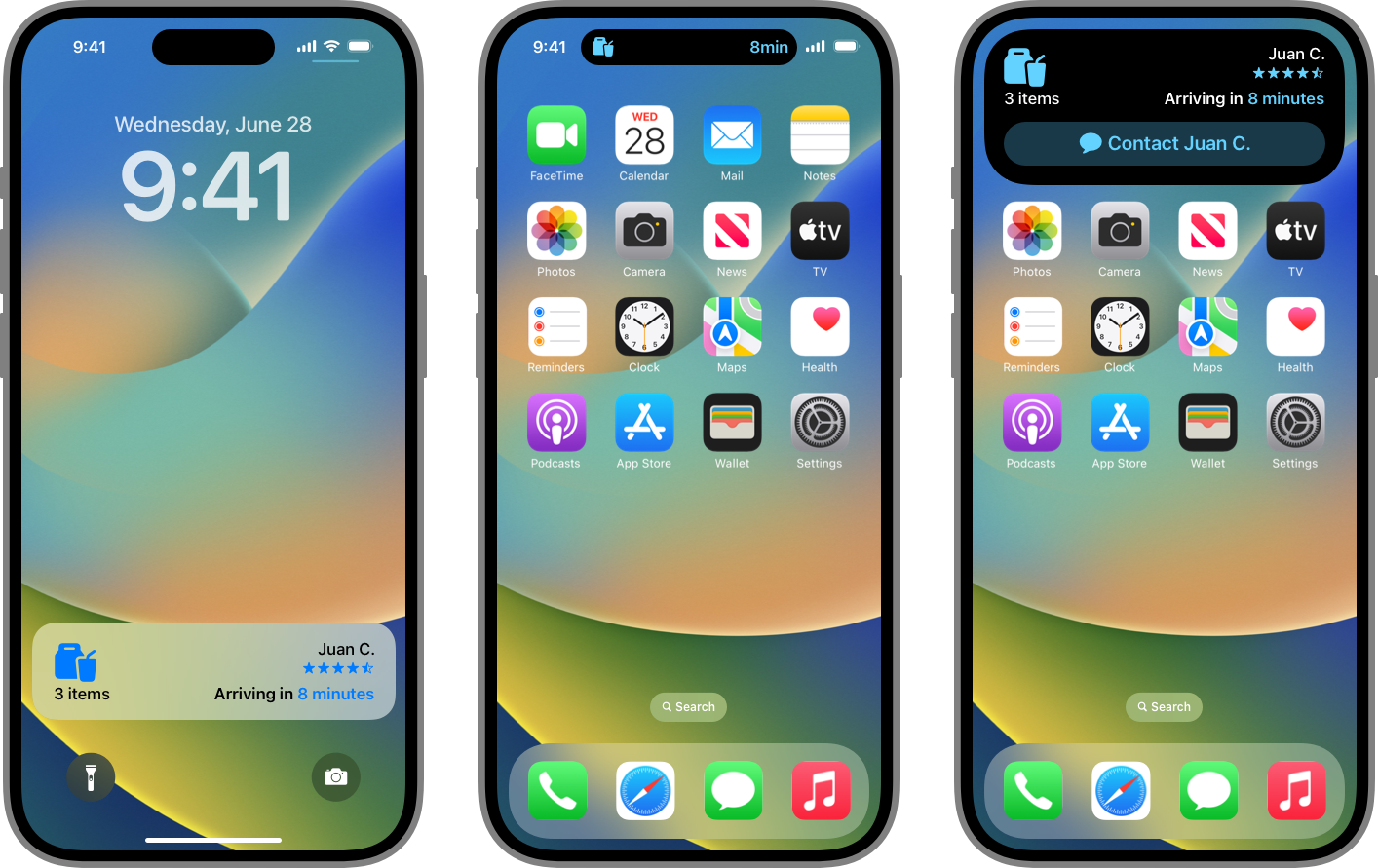
An example of Live Activity displayed in the Lock Screen (left), compact Dynamic Island (middle), and expanded Dynamic Island (right).
A few example use case might include:
- Tracking the progress of a food delivery
- Getting the next step of your travel journey at a glance
- Viewing your current workout session statistics
- Following your favorite sports team upcoming and ongoing game scores
- Monitoring the latest news headlines for a while
Creating your first Live Activity
Follow Apple's guide on how to create your first Live Activity.
Here's an overview of its steps:
- Declaring support for Live Activities in your application's
Info.plist
. - Creating a new Widget Extension target in Xcode, checking Include Live Activity box.
- Structuring the Attributes and Content State fields you will need to access to display the proper data.
- Crafting a UI using SwiftUI for the different display states a Live Activity can take.
- Giving a default deeplink to your application and optionaly adding buttons to specific actions.
- Start a Live Activity when the user takes an appropriate action in your application.
- Apple recommends listing existing Live Activities when opening your application to refresh their state or end any outdated ones.
Updating a Live Activity consists of pushing a new Content State to an existing Live Activity, optionally updating it's state date, relevance score, playing a sound, or even ending the Live Activity with an optional dismissal date. You will need to provide it with a full Content State at each update. Your UI code will then be called to update the Live Activity display using both its Attributes and new Content State.
You do not need to read Apple's guide on Updating and ending your Live Activity with ActivityKit push notifications because WonderPush takes care of the nitty-gritty details for you. You will simply need to tell the WonderPush iOS SDK about your Live Activities, and send updates using the WonderPush Management API.
Registering your Live Activities with WonderPush
Upgrade first!
Make sure to upgrade to the WonderPush iOS SDK version 4.2.0+ before starting.
When your application starts, you need to register your Live Activity class(es) with WonderPush.
First, let's make the class accessible to your application: Find your Live Activity's .swift
file in the Project Navigator (left pane first tab with a folder icon) and click on it, then in the File Inspector (right pane first tab with a file icon) under Target Membership and check your application's target.
Second, let's register it with WonderPush:
// Add the following import if not already there
import WonderPush
// Let's assume your Live Actitivity has its attributes class named: MyLiveActivityExtensionAttributes.
// Choose one of the options and call it once and for all, when your application starts.
// Option 1:
// You can register any Live Activity of this class with a single topic.
// This is the easiest way to get started, if you only have a single Live Activity at a time.
WonderPush.registerActivityAttributes(MyLiveActivityExtensionAttributes.self, topic: "latestNews")
// Option 2:
// You can also register multiple Live Activities by deriving a topic from their Attributes.
// This is required if start multiple Live Activities of a given class,
// because you will need to update each one separately.
WonderPush.registerActivityAttributes(MyLiveActivityExtensionAttributes.self, topic: { activity in "match-\(activity.attributes.matchId)" })
// Other options:
// There are a few other overloaded definitions to help you with the most advanced use cases, take a look if you're curious.
With this simple call, WonderPush will automatically list all existing and future Live Activities, register them with the API, and keep them up-to-date.
Check you are using a P8 push key, not a P12 push certificate
Head over to your Settings / Platforms page, and click on Edit iOS settings.
If you see an orange warning telling you ”Prefer using an APNs Key (.p8) over an APNs Push Certificate (.p12); it does not expire every year and supports more iOS features, like Live Activities.”, then you should follow the Creating or using an Apple Push Notification service (APNs) Key guide.
If you see Current push key you can continue.
Sending updates to your Live Activities
To send an update to your Live Activity, you must know it's topic, and the expected format for it's content state, and call our classic POST /v1/deliveries
endpoint.
The topic is what you configured in the WonderPush.registerActivityAttributes()
call. It can be a constant if you only ever have a single Live Activity (latestNews
in our first option's example), or it can include some identifier dynamically (match-123
in our second option's example). You need to give this value in the targetLiveActivityTopic
parameter in the API call.
Take a look at your LiveActivity ContentState
struct inside its Attributes
struct in your code. You will need to provide your Live Activity with a complete ContentState
object in the liveActivity.contentState
property of the notification
parameter. Any optional property can be left out, but every other must be filled. Otherwise iOS won't be able to reconstruct a valid ContentState
struct instance to provide your Live Activity with, and you appart from a quiet log you will be left thinking the update never reached your device.
You can also add a sound by filling the alert.ios.sound
property of the notification
parameter, and a text for the user's Apple Watch in the alert.title
and alert.text
properties of the notification
parameter.
curl -XPOST https://management-api.wonderpush.com/v1/deliveries \
-d accessToken=YOUR_PROJECT_ACCESS_TOKEN \
-d targetLiveActivityTopic='YOUR_LIVEACTIVITY_TOPIC' \
-d notification:='
{
"liveActivity": {
"contentState": { PAYLOAD_FOR_LIVEACTIVITY_CONTENT_STATE }
},
"alert": {
"title": "TITLE_FOR_APPLE_WATCH",
"text": "TEXT_FOR_APPLE_WATCH",
"ios": {"sound":"default"}
}
}'
Ending a Live Activity remotely
And update can cause the Live Activity to end, and automatically dismiss at the desired date, upto 4 hours in the future.
curl -XPOST https://management-api.wonderpush.com/v1/deliveries \
-d accessToken=YOUR_PROJECT_ACCESS_TOKEN \
-d targetLiveActivityTopic='YOUR_LIVEACTIVITY_TOPIC' \
-d notification:='
{
"liveActivity": {
"event": "end",
"dismissalTime": "5 minutes",
"contentState": { PAYLOAD_FOR_LIVEACTIVITY_CONTENT_STATE }
}
}'
Even if you do not end a Live Activity remotely, iOS will automatically end it 8 hours after its start, and automatically dismiss it 4 hours after its end.
Updated over 1 year ago