iOS SDK Reference
iOS SDK Swift and Objective-C Reference
Initialization | |
setClientId:secret: | Initializes the SDK. |
setupDelegateForApplication | Installs WonderPush as your AppDelegate. |
setupDelegateForUserNotificationCenter | Installs WonderPush as UNUserNotificationCenter delegate. |
setLogging | Controls SDK logging. |
isInitialized | Returns whether the WonderPush SDK has been given the clientId and clientSecret. |
isReady | Returns whether the WonderPush SDK is ready to operate. |
setDelegate | Sets the delegate for the WonderPushSDK. |
Subscribing users | |
subscribeToNotifications | Prompts user to subscribe to push notifications. |
unsubscribeFromNotifications | Unsubscribes user from push notifications. |
isSubscribedToNotifications | Tells whether user is subscribed to push notifications. |
Segmentation | |
trackEvent | Sends an event with a name and payload of your choice. |
addTag / addTags | Adds one or more tags to this installation. |
removeTag / removeTags | Removes one or more tags from this installation. |
removeAllTags | Removes all tags from this installation. |
hasTag | Tests whether a given tag is attached to this installation. |
getTags | Returns all the tags attached of this installation. |
getPropertyValue | Returns the value of a given property associated to this installation. |
getPropertyValues | Returns an immutable list of the values of a given property associated to this installation. |
addProperty | Adds the value to a given property associated to this installation. |
removeProperty | Removes the value from a given property associated to this installation. |
setProperty | Sets the value to a given property associated to this installation. |
unsetProperty | Removes the value of a given property associated to this installation. |
putProperties | Associates the provided name/value pairs to this installation. |
getProperties | Returns all the name/value pairs associated to this installation using putProperties. |
country | Returns the user's country. |
setCountry | Overrides the user's country. |
currency | Returns the user's currency. |
setCurrency | Overrides the user's currency. |
locale | Returns the user's locale. |
setLocale | Overrides the user's locale. |
timeZone | Returns the user's time zone. |
setTimeZone | Overrides the user's time zone. |
User IDs | |
setUserId | Assigns your own user ID to an installation. |
userId | Returns the user ID you've assigned to this installation if any. |
Installation info | |
installationId | Returns the installationId identifying this installation in your application. |
pushToken | Returns the push token (also called device token by Apple). |
Privacy | |
setRequiresUserConsent | Sets whether user consent is required before the SDK is allowed to work. |
setUserConsent | Provides privacy consent. |
disableGeolocation | Disables collection of the geolocation. |
enableGeolocation | Enables collection of the geolocation. |
setGeolocation | Overrides the user's current geolocation. |
clearEventsHistory | Clears all events recorded using trackEvent. |
clearPreferences | Clears all the name/value pairs associated to this installation using putProperties. |
clearAllData | Deletes any event, installation and potential user objects associated with all installations present on the device. |
downloadAllData: | Initiates the download of all the WonderPush data related to the current installation, in JSON format. |
Delegate protocol | |
wonderPushWillOpenURL: | Lets you overwrite URLs that WonderPush will open using UIApplication:openURL: . |
wonderPushWillOpenURL: withCompletionHandler: | Lets you overwrite URLs that WonderPush will open using UIApplication:openURL: . |
NSNotificationCenter | |
WP_NOTIFICATION_OPENED_BROADCAST | NSNotificationCenter notification fired when user clicks a broadcast push notification |
Popups (formerly known as in-app messaging) | |
messageDisplayComponent: | Lets you handle the display of popups yourself. |
delegate | Gets called whenever a popup is displayed, clicked, dismissed or generates an error. |
messageDisplaySuppressed | Setting this property to true prevents the display of any popup message. Defaults to false . |
Initialization
setClientId:secret:
setClientId:secret:
Call this function from your AppDelegate
's application:willFinishLaunchingWithOptions:
to initialize WonderPush. You can find your clientId
and secret
on your dashboard, in the Configuration / Settings / iOS app section.
func application(_ application: UIApplication, willFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
WonderPush.setClientId("YOUR_CLIENT_ID", secret:"YOUR_CLIENT_SECRET")
}
- (BOOL)application:(UIApplication *)application willFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[WonderPush setClientId:@"YOUR_CLIENT_ID" secret:@"YOUR_CLIENT_SECRET"];
}
setupDelegateForApplication
setupDelegateForApplication
Installs WonderPush
as your application delegate. All calls that are not intercepted by WonderPush
will be forwarded to your original application delegate.
func application(_ application: UIApplication, willFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
WonderPush.setupDelegateForApplication(application)
}
- (BOOL)application:(UIApplication *)application willFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[WonderPush setupDelegateForApplication:application];
}
In order to operate, WonderPush
needs to handle:
application:didFinishLaunchingWithOptions:
,application:didReceiveRemoteNotification:
,application:didRegisterForRemoteNotificationsWithDeviceToken:
,application:didFailToRegisterForRemoteNotificationsWithError:
,application:didReceiveLocalNotification:
,application:didReceiveRemoteNotification:fetchCompletionHandler:
,applicationDidBecomeActive:
,applicationDidEnterBackground:
,application:didRegisterUserNotificationSettings:
.
If you decide not to call setupDelegateForApplication
, you must forward all these methods to the WonderPush
object from your application delegate like this:
#import <WonderPush/WonderPush.h>
- (BOOL)application:(UIApplication*)application didFinishLaunchingWithOptions:(NSDictionary*)launchOptions
{
// Other code, including WonderPush initialization
// ...
[WonderPush application:application didFinishLaunchingWithOptions:launchOptions];
return YES;
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo {
[WonderPush application:application didReceiveRemoteNotification:userInfo];
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[WonderPush application:application didRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
}
- (void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error {
[WonderPush application:application didFailToRegisterForRemoteNotificationsWithError:error];
}
- (void)application:(UIApplication *)application didReceiveLocalNotification:(UILocalNotification *)notification {
[WonderPush application:application didReceiveLocalNotification:notification];
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler {
[WonderPush application:application didReceiveRemoteNotification:userInfo fetchCompletionHandler:completionHandler];
}
- (void)applicationDidBecomeActive:(UIApplication *)application {
[WonderPush applicationDidBecomeActive:application];
}
- (void)applicationDidEnterBackground:(UIApplication *)application {
[WonderPush applicationDidEnterBackground:application];
}
- (void)application:(UIApplication *)application didRegisterUserNotificationSettings:(UIUserNotificationSettings *)notificationSettings {
[WonderPush application:application didRegisterUserNotificationSettings:notificationSettings];
}
import WonderPush
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
// Other code, including WonderPush initialization
// ...
WonderPush.application(application, didFinishLaunchingWithOptions: launchOptions)
return true
}
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
WonderPush.application(application, didRegisterForRemoteNotificationsWithDeviceToken: deviceToken)
}
func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
WonderPush.application(application, didFailToRegisterForRemoteNotificationsWithError: error)
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
WonderPush.application(application, didReceiveRemoteNotification: userInfo, fetchCompletionHandler: completionHandler)
}
func applicationDidBecomeActive(_ application: UIApplication) {
WonderPush.applicationDidBecomeActive(application)
}
func applicationDidEnterBackground(_ application: UIApplication) {
WonderPush.applicationDidEnterBackground(application)
}
func application(_ application: UIApplication, didRegister notificationSettings: UIUserNotificationSettings) {
WonderPush.application(application, didRegister: notificationSettings)
}
If you get the following warning
WARNING: method swizzling detected! It's potentially incompatible with WonderPush.setupDelegate(for:) and you might experience some AppDelegate methods not being called. Please read: https://docs.wonderpush.com/docs/ios-sdk#setupdelegateforapplication
Some frameworks like Firebase do method swizzling. Sometimes, this doesn't play well with WonderPush. In particular, some AppDelegate methods might not get called. You have 2 options:
- forward the above methods and stop using
WonderPush.setupDelegate(for:)
- or disable method swizzling for the other framework, here's how to achieve this in Firebase.
setupDelegateForUserNotificationCenter
setupDelegateForUserNotificationCenter
Installs WonderPush as UNUserNotificationCenter
delegate. All calls that are not intercepted by WonderPush
will be forwarded to your original UNUserNotificationCenterDelegate
. This method is available starting iOS 10.
func application(_ application: UIApplication, willFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
if #available(iOS 10.0, *) {
WonderPush.setupDelegateForUserNotificationCenter(application)
}
}
- (BOOL)application:(UIApplication *)application willFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
if (@available(iOS 10.0, *)) {
[WonderPush setupDelegateForUserNotificationCenter];
}
}
In order to operate, WonderPush
needs to handle:
userNotificationCenter:willPresentNotification:withCompletionHandler:
,userNotificationCenter:didReceiveNotificationResponse:withCompletionHandler:
.
If you decide not to call setupDelegateForUserNotificationCenter
, you must forward all these methods to the WonderPush
object from your UNUserNotificationCenterDelegate
.
setLogging:
setLogging:
You can use this method to activate WonderPush verbose logging. By default, verbose logging is disabled and only errors and warnings are logged.
WonderPush.setLogging(true)
[WonderPush setLogging:YES];
isInitialized
isInitialized
This method returns a boolean indicating whether the SDK has been initialized with a call to setClientId:secret
.
No network can be performed before the SDK is initialized. Further use of the SDK methods will be dropped until initialized. Such call will be ignored and logged in the device console.
isReady
isReady
Returns whether the WonderPush SDK is ready to operate. You can also listen to the WP_NOTIFICATION_INITIALIZED
notification center notification.
if (WonderPush.isReady) {
// Do something.
}
// Alternatively, you can listen to the WP_NOTIFICATION_INITIALIZED notification:
NotificationCenter.default.addObserver(forName: NSNotification.Name(rawValue: WP_NOTIFICATION_INITIALIZED), object: nil, queue: nil, using: {notification in
// Do something.
})
if ([WonderPush isReady]) {
// Do something.
}
// Alternatively, you can listen to the WP_NOTIFICATION_INITIALIZED notification:
[[NSNotificationCenter defaultCenter] addObserverForName:WP_NOTIFICATION_INITIALIZED object:nil queue:nil usingBlock:^(NSNotification *note) {
// Do something.
}];
setDelegate:
setDelegate:
Sets the delegate for the WonderPushSDK. Setting the delegate lets you control various behaviors of the WonderPushSDK at runtime.
It is advised to set the delegate as early as possible, preferably in application:didFinishLaunchingWithOptions:
.
See Delegate Protocol for details on what the delegate can do.
Subscribing users
subscribeToNotifications
subscribeToNotifications
Subscribes to push notifications. Triggers the system permission prompt:
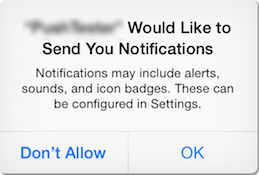
WonderPush.subscribeToNotifications()
[WonderPush subscribeToNotifications];
unsubscribeFromNotifications
unsubscribeFromNotifications
Unsubscribes from push notifications.
Please note that calling this function does not affect the push notification permission. See Soft opt-out.
WonderPush.unsubscribeFromNotifications()
[WonderPush unsubscribeFromNotifications];
isSubscribedToNotifications
isSubscribedToNotifications
Returns a boolean indicating whether the user is subscribed to push notifications.
Please note that by default, iOS users are subscribed to notifications and no action is needed to receive data-only notifications.
In order to show notifications to the user, you need to call
subscribeToNotifications
To check the state of the display permission, you can use Apple's
UserNotifications
framework like this:import UserNotifications UNUserNotificationCenter.current().getNotificationSettings(completionHandler: {settings in let notificationsVisible = settings.authorizationStatus == .authorized // ... do something with that information })
#import <UserNotifications/UserNotifications.h> [UNUserNotificationCenter.currentNotificationCenter getNotificationSettingsWithCompletionHandler:^(UNNotificationSettings *settings) { UNAuthorizationStatus status = settings.authorizationStatus; BOOL notificationsVisible = status == UNAuthorizationStatusAuthorized; // ... do something with this information }];
Segmentation
Segmentation functions allow you to mark installations so they can be added to segments and you can send them targeted notifications later.
There are many ways of performing segmentation:
Tags are like labels you can stick on users. Use tags to create segments in your dashboard and send targeted push notifications. Example: all users that have the "customer" tag.
Events have a date, a type
of your choice and attributes
. Use events to create segments in your dashboard and send targeted push notifications. Example: all users that purchased in the last hour is a typical event-based segment.
Installation properties represent traits of the user. That's a good place to store age, gender and other data traditionally used for segmentation. Create property-based segments in your dashboard. Example: all users above 18 is a typical property-based segment.
trackEvent
trackEvent
Tracks a custom event of your choice. E.g. purchase
.
Parameter | Type | Description |
---|---|---|
eventType | String | Required The type of the event to track. Event names starting with @ are reserved for internal use and cannot be used here. |
attributes | NSDictionary | Attributes associated with this event. See format of property names for detailed syntax. |
Example:
WonderPush.trackEvent("purchase", attributes: [
"string_product": "Some product",
"float_price": 1.99,
])
[WonderPush trackEvent:@"purchase"
attributes:@{
@"string_product": @"Some product",
@"float_price": @(1.99),
}];
addTag
/ addTags
addTag
/ addTags
Adds one or more tags to the current installation.
WonderPush.addTag("customer");
WonderPush.addTags(["economics", "sport", "politics"]);
[WonderPush addTag:@"customer"];
[WonderPush addTags:@[@"economics", @"sport", @"politics"]];
removeTag
/ removeTags
removeTag
/ removeTags
Removes one or more tags from the current installation.
[WonderPush removeTag:@"customer"];
[WonderPush removeTags:@[@"economics", @"sport", @"politics"]];
removeAllTags
removeAllTags
Removes all tags from the current installation.
hasTag
hasTag
Tests whether a given tag is attached to the current installation.
if (WonderPush.hasTag("customer")) {
// Display paid features
}
if ([WonderPush hasTag:@"customer"]) {
// Display paid features
}
getTags
getTags
Returns all the tags attached to the current installation as an NSOrderedSet<NSString*> *
.
getPropertyValue
getPropertyValue
Returns the value of a given property associated to the current installation.
If the property stores an array, only the first value is returned. This way you don’t have to deal with potential arrays if that property is not supposed to hold one. Returns NSNull
instead of nil
if the property is absent or has an empty array value.
let value = WonderPush.getPropertyValue("string_lastname")
// You should always check the type of the returned value, especially as `NSNull` is used when there is no value
if let lastName = value as? String {
// Use lastName
}
id value = [WonderPush getPropertyValue:@"string_lastname"];
// You should always check the type of the returned value, especially as `NSNull` is used when there is no value
if ([value isKindOfClass:[NSString class]) {
NSString *lastName = (NSString *) value;
// Use lastName
}
getPropertyValues
getPropertyValues
Returns an array of the values of a given property associated to the current installation.
If the property does not store an array, an array is returned nevertheless. This way you don’t have to deal with potential scalar values if that property is supposed to hold an array. Returns an empty array instead of nil
or NSNull
if the property is absent or is NSNull
. Returns an array wrapping any scalar value held by the property.
let values = WonderPush.getPropertyValues("string_favoritePlayers")
NSArray *values = [WonderPush getPropertyValues:@"string_favoritePlayers"];
addProperty
addProperty
Adds the value to a given property associated to the current installation.
The stored value is made an array if not already one. If the given value is an NSArray
, all its values are added. If a value is already present in the stored value, it won’t be added.
See format of property names for detailed syntax.
// You can add a single value
WonderPush.addProperty("string_interests", "sport")
// You can add an array of values
WonderPush.addProperty("string_interests", ["sport", "entertainment"])
// You can add a single value
[WonderPush addProperty:@"string_interests" value:@"sport"];
// You can add an array of values
[WonderPush addProperty:@"string_interests" value:@[@"sport", @"entertainment"]];
removeProperty
removeProperty
Removes the value from a given property associated to the current installation.
The stored value is made an array if not already one. If the given value is an NSArray
, all its values are removed. If a value is present multiple times in the stored value, they will all be removed.
// You can remove a single value
WonderPush.removeProperty("string_interests", "sport")
// You can remove an array of values
WonderPush.removeProperty("string_interests", ["sport", "entertainment"])
// You can remove a single value
[WonderPush removeProperty:@"string_interests" value:@"sport"];
// You can remove an array of values
[WonderPush removeProperty:@"string_interests" value:@[@"sport", @"entertainment"]];
setProperty
setProperty
Sets the value to a given property associated to the current installation.
The previous value is replaced entirely. The value can be an NSString
, NSNumber
, NSDictionary
, NSArray
, or NSNull
(which has the same effect as unsetProperty).
See format of property names for detailed syntax.
// You can set a single value
WonderPush.setProperty("bool_isCustomer", true)
// You can remove a field using NSNull
WonderPush.setProperty("int_age", NSNull())
// You can set an array of values
WonderPush.setProperty("string_interests", ["sport", "entertainment"])
// You can set a single value
[WonderPush setProperty:@"bool_isCustomer" value:@YES];
// You can remove a field using NSNull
[WonderPush setProperty:@"int_age" value:[NSNull null]];
// You can set an array of values
[WonderPush setProperty:@"string_interests" value:@[@"sport", @"entertainment"]];
unsetProperty
unsetProperty
Removes the value of a given property associated to the current installation.
The previous value is replaced with NSNull
.
WonderPush.unsetProperty("string_favoritePlayers")
[WonderPush unsetProperty:@"string_favoritePlayers"];
putProperties
putProperties
Updates the properties of the current installation. Omitting a previously set property leaves it untouched. To remove a property, you must pass it explicitly with a value of NSNull
.
Parameter | Type | Description |
---|---|---|
properties | NSDictionary | Properties to add or update. See format of property names for detailed syntax. |
Example:
WonderPush.putProperties([
"int_age": 34
])
[WonderPush putProperties:@{
@"int_age": @34,
}];
getProperties
getProperties
Returns an NSDictionary
containing the properties of the current installation.
let properties = WonderPush.getProperties()
let name = properties?["string_name"]
NSDictionary *properties = [WonderPush getProperties];
NSString *name = properties[@"string_name"];
country
country
Returns the user's country, either as previously stored, or as guessed from the system.
setCountry
setCountry
Overrides the user's country.
Defaults to getting the country code from the system default locale.
You should use an ISO 3166-1 alpha-2 country code, eg: US
, FR
, GB
.
Use null
to disable the override.
currency
currency
Returns the user's currency, either as previously stored, or as guessed from the system.
setCurrency
setCurrency
Overrides the user's currency.
Defaults to getting the currency code from the system default locale.
You should use an ISO 4217 currency code, eg: USD
, EUR
, GBP
.
Use null
to disable the override.
locale
locale
Returns the user's locale, either as previously stored, or as guessed from the system.
setLocale
setLocale
Overrides the user's locale.
Defaults to getting the language and country codes from the system default locale.
You should use an xx-XX
form of RFC 1766, composed of a lowercase ISO 639-1 language code, an underscore or a dash, and an uppercase ISO 3166-1 alpha-2 country code.
Use null
to disable the override.
timeZone
timeZone
Returns the user's time zone, either as previously stored, or as guessed from the system.
setTimeZone
setTimeZone
Overrides the user's time zone.
Defaults to getting the time zone code from the system default locale.
You should use an IANA time zone database codes, Continent/Country
style preferably like Europe/Paris
, or abbreviations like CET
, PST
, UTC
, which have the drawback of changing on daylight saving transitions.
Use null
to disable the override.
User IDs
setUserId:
setUserId:
Assigns your own user ID to an installation. See User IDs.
WonderPush.setUserId("YOUR_OWN_USER_ID")
[WonderPush setUserId:@"YOUR_OWN_USER_ID"];
userId
userId
Returns the user ID you've assigned to this installation if any.
Installation info
installationId
installationId
Returns a string representing the installationId identifying this installation in your application.
pushToken
pushToken
Returns a string representing the push token (device token) for this device.
Privacy
This section details methods you can use to achieve GDPR compliance and respect user privacy in general.
setRequiresUserConsent
setRequiresUserConsent
Sets whether user consent is required before the SDK is allowed to work. Call this method before setClientId:secret:
.
When user consent is required, the SDK will refuse to work until setUserConsent
is called with a value of true
. All SDK methods will return nil
or have no effect. In addition, warning logs will be emitted.
Example:
func application(_ application: UIApplication, willFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
WonderPush.setRequiresUserConsent(true)
WonderPush.setClientId("YOUR_CLIENT_ID", secret:"YOUR_CLIENT_SECRET")
}
- (BOOL)application:(UIApplication *)application willFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[WonderPush setRequiresUserConsent:YES];
[WonderPush setClientId:@"YOUR_CLIENT_ID" secret:@"YOUR_CLIENT_SECRET"];
}
setUserConsent
setUserConsent
Sets user privacy consent. When you've called setRequiresUserConsent(true)
, calling this method with true
will unlock the SDK. Calling setUserConsent
with false
blocks the SDK again.
WonderPush.setUserConsent(true)
[WonderPush setUserConsent:YES];
disableGeolocation
disableGeolocation
Disables the collection of the user's geolocation.
You can call this method before initializing the SDK.
enableGeolocation
enableGeolocation
Enables the collection of the user's geolocation.
You can call this method before initializing the SDK.
setGeolocation
setGeolocation
Overrides the user's geolocation.
You can call this method before initializing the SDK.
Using this method you can have the user's location be set to wherever you want.
This may be useful to use a pre-recorded location.
Note that the value is not persisted.
Calling this method with nil
has the same effect as calling disableGeolocation()
.
clearEventsHistory
clearEventsHistory
Instructs to delete any event associated with the all installations present on the device, locally and on WonderPush servers.
WonderPush.clearEventsHistory()
[WonderPush clearEventsHistory];
clearPreferences
clearPreferences
Instructs to delete any custom data (including installation properties) associated with the all installations present on the device, locally and on WonderPush servers.
WonderPush.clearPreferences()
[WonderPush clearPreferences];
clearAllData
clearAllData
Instructs to delete any event, installation and potential user objects associated with all installations present on the device, locally and on WonderPush servers.
WonderPush.clearAllData()
[WonderPush clearAllData];
downloadAllData:
downloadAllData:
Initiates the download of all the WonderPush data relative to the current installation, in JSON format.
Parameter | Type | Description |
---|---|---|
completion | Block | Completion handler called upon success or error |
Example:
WonderPush.downloadAllData({(data:Data?, error:Error?) in
if let error = error {
print("Could not download data: %@", error)
return
}
if let data = data, let string = String(data: data, encoding: String.Encoding.utf8) {
let controller = UIActivityViewController(activityItems: [string], applicationActivities: nil)
// self should be a UIViewController
self.present(controller, animated: true, completion: nil)
}
})
[WonderPush downloadAllData:^(NSData *data, NSError *error) {
if (error) {
NSLog(@"Could not download data: %@", error);
return;
}
// Upon success, present a UIActivityViewController
NSString *string = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];
UIActivityViewController *controller = [[UIActivityViewController alloc] initWithActivityItems:@[string] applicationActivities:nil];
// self should be a UIViewController
[self presentViewController:controller animated:YES completion:nil];
}];
Delegate protocol
The WonderPushDelegate
protocol lets you customize various aspects of the WonderPush behavior at runtime. Implement it, then call setDelegate
on the WonderPush
object with your implementation as argument.
wonderPushWillOpenURL:
wonderPushWillOpenURL:
This method is deprecated, you should implement wonderPushWillOpenURL:withCompletionHandler:
instead.
Lets you overwrite URLs that WonderPush will open. This method is called with a URL object as parameter and returns a URL object that WonderPush will pass to UIApplication
by calling openURL:
.
func wonderPushWillOpenURL(_ url:URL) -> URL {
// Decide what URL to return
return url;
}
- (NSURL *) wonderPushWillOpenURL:(NSURL *)URL {
// Decide what URL to return
return URL;
}
wonderPushWillOpenURL:withCompletionHandler:
wonderPushWillOpenURL:withCompletionHandler:
Lets you overwrite URLs that WonderPush will open. This method is called with a URL object as parameter and returns a URL object that WonderPush will pass to UIApplication
by calling openURL:
.
func wonderPushWillOpenURL(_ url:URL, withCompletionHandler completionHandler: ((URL?) -> Void)) {
// Decide what URL to use
completionHandler(url);
}
- (void) wonderPushWillOpenURL:(NSURL *)URL withCompletionHandler:(void (^)(NSURL *))completionHandler {
// Decide what URL to use
completionHandler(URL);
}
NSNotificationCenter
WP_NOTIFICATION_OPENED_BROADCAST
WP_NOTIFICATION_OPENED_BROADCAST
When a notification is clicked whose action is to open the special URL wonderpush://notificationOpen/broadcast
, our SDKs forward this click to your app or website without taking any other action.
Such notifications can be created from the online dashboard by choosing the option to Let application code decide from the compose tab of the notification edition interface.
You can listen to these clicks and decide what to do by adding a small amount of code.
// Listen to a NotificationCenter notification.
// A good place to put this code is your AppDelegate's application:didFinishLaunchingWithOptions:
NotificationCenter.default.addObserver(forName: NSNotification.Name(rawValue: WP_NOTIFICATION_OPENED_BROADCAST), object: nil, queue: nil, using: {notification in
if let pushNotification = notification.userInfo {
debugPrint("Notification clicked:", pushNotification)
}
})
// Listen to a NSNotificationCenter notification.
// A good place to put this code is your AppDelegate's application:didFinishLaunchingWithOptions:
[[NSNotificationCenter defaultCenter] addObserverForName:WP_NOTIFICATION_OPENED_BROADCAST object:nil queue:nil usingBlock:^(NSNotification *note) {
NSDictionary *pushNotification = note.userInfo;
NSLog(@"Notification clicked: %@", pushNotification);
}];
Popups (formerly known as in-app messaging)
Most popups methods are located on the global WonderPush.InAppMessaging
object (WPInAppMessaging
in objective-c).
messageDisplayComponent
messageDisplayComponent
Setting the messageDisplayComponent
lets you handle the display of popups yourself via an object that implements the InAppMessagingDisplay
protocol (WPInAppMessagingDisplay
in objective-c).
InAppMessaging.inAppMessaging().messageDisplayComponent = MessageDisplayComponent()
WPInAppMessaging.inAppMessaging.messageDisplayComponent = [MessageDisplayComponent new];
See handling the display of popups yourself for details on how to implement MessageDisplayComponent
.
inAppMessaging.delegate
inAppMessaging.delegate
The delegate
property of the InAppMessaging.inAppMessaging()
interface can be set to an object implementing the InAppMessagingDisplayDelegate
protocol (WPInAppMessagingDisplayDelegate
in objective-c).
Views, clicks, dismisses and errors are all forwarded to that object when present.
InAppMessaging.inAppMessaging().delegate = myDelegate; // an object implementing the InAppMessagingDisplayDelegate protocol
WPInAppMessaging.inAppMessaging.delegate = myDelegate; // an object implementing the WPInAppMessagingDisplayDelegate protocol
inAppMessaging.messageDisplaySuppressed
inAppMessaging.messageDisplaySuppressed
The boolean messageDisplaySuppressed
property of the InAppMessaging.inAppMessaging
interface can be set to true
to prevent the display of any popup. Setting the property to false
(its default value) permits such display again.
InAppMessaging.inAppMessaging().messageDisplaySuppressed = true;
WPInAppMessaging.inAppMessaging.messageDisplaySuppressed = YES;
Updated almost 2 years ago