Website SDK Reference
Website SDK Javascript Reference
Initialization | |
init | Initializes the Web SDK. |
push | Gives access to the rest of the SDK API asynchronously, when it is loaded and ready to use. |
Subscribing users | |
subscribeToNotifications | Prompts user to subscribe to web push notifications. |
unsubscribeFromNotifications | Unsubscribes user from web push notifications. |
isSubscribedToNotifications | Tells whether user is subscribed to web push notifications. |
sendSelfNotification | Sends a notification back to the user, for testing, or with a given message. |
setUserId | Assigns the provided user ID to the user. When the user ID changes, a new installation is created. |
unsetUserId | Clears out the userId. Equivalent to setUserId(null) |
getUserId | Returns a Promise that resolves to a string equal to the user ID or null . |
getInstallationId | Returns a Promise that resolves to a string equal to the installation ID or null . |
User Interfaces | |
showSubscriptionDialog | Shows a system-like dialog box at the top of the screen prompting users to subscribe to push notifications. |
showSubscriptionBell | Shows a small widget at the lower-left corner of the screen that prompts users to subscribe to push notifications when clicked. |
showSubscriptionSwitch | Shows a switch-like widget inside the specified container DOM element that allows users to subscribe and unsubscribe from push notifications. |
showTagSwitches | Shows a collection of switch-like widgets inside the specified container DOM element that allows users to subscribe and unsubscribe from push notifications, and tag themselves at the same time. |
Segmentation | |
trackEvent | Sends an event with a name and payload of your choice. |
addTag | Adds one or more tags to this installation. |
removeTag | Removes one or more tags from this installation. |
removeAllTags | Removes all tags from this installation. |
hasTag | Tests whether a given tag is attached to this installation. |
getTags | Returns all the tags attached of this installation. |
getPropertyValue | Returns the value of a given property associated to this installation. |
getPropertyValues | Returns an immutable list of the values of a given property associated to this installation. |
addProperty | Adds the value to a given property associated to this installation. |
removeProperty | Removes the value from a given property associated to this installation. |
setProperty | Sets the value to a given property associated to this installation. |
unsetProperty | Removes the value of a given property associated to this installation. |
putProperties | Associates the provided name/value pairs to this installation. |
getProperties | Returns all the name/value pairs associated to this installation using putProperties. |
useGeolocation | Enables or disables the collection of the geolocation position. |
getCountry | Returns the user's country. |
setCountry | Overrides the user's country. |
getCurrency | Returns the user's currency. |
setCurrency | Overrides the user's currency. |
getLocale | Returns the user's locale. |
setLocale | Overrides the user's locale. |
getTimeZone | Returns the user's time zone. |
setTimeZone | Overrides the user's time zone. |
Privacy | |
setUserConsent | Provides privacy consent. |
clearEventsHistory | Clears all events recorded using trackEvent. |
clearPreferences | Clears all the name/value pairs associated to this installation using putProperties. |
clearAllData | |
downloadAllData | Initiates the download of all the WonderPush data related to the current installation, in JSON format. |
Custom DOM Events | |
subscription | A custom DOM event fired on the window object when the user subscribes or unsubscribes. |
Popups (formerly known as In-app messaging) | |
setInAppMessagingDisplayCallback | Lets you handle the display of popups yourself. |
setInAppMessagesSuppressed | Lets you disable or enable popups. |
getInAppMessagesSuppressed | Whether popup is disabled. |
Initialization
Loading SDK Asynchronously
WonderPush recommends loading wonderpush-loader.min.js
with the async
flag so your page load times don't increase. To use it, place the following code before calling any other WonderPush functions.
<script src="https://cdn.by.wonderpush.com/sdk/1.1/wonderpush-loader.min.js" async></script>
<script>window.WonderPush = window.WonderPush || [];</script>
Our API functions can be called asynchronously using either:
WonderPush.push(["functionName", param1, param2]);
WonderPush.push(function() { WonderPush.functionName(param1, param2); });
See push
for more details.
init
init
Call this function from every page of your site to initialize WonderPush. This call is required before any other function can be used. You must use push
with the array syntax to call init
.
window.WonderPush = window.WonderPush || [];
WonderPush.push(["init", {
webKey: "YOUR_WEBKEY",
// other initialization options
}]);
Please note that calling
init
more than once has no effect. If you are using Google Tag Manager, you will have to replace the WonderPush tag with a regular script tag that callsWonderPush.push(['init', { webKey: "YOUR_WEBKEY" }])
Parameter | Type | Description |
---|---|---|
options | Object | Initialization options described below |
Initialization options are:
Option | Type | Description |
---|---|---|
webKey | String | Required The web key of your project. Find it your dashboard under the Settings / Configuration page, in the Website tab. |
userId | String | The unique identifier of the user in your systems. Provide this whenever the value should change. |
applicationName | String | The name of your website. Defaults to the value you've chosen in your dashboard, or your website hostname. Displayed during the registration process. |
applicationVersion | String | The version of your website. Can be used for Segmentation. |
geolocation | "auto" or Boolean | Report the geolocation of this installation. Possible values are: - "auto" (default): watch and report the geolocation of this installation if the permission is already granted, but never prompt for the GeoLocation permission.- true : prompt the user at page load if necessary, watch and report the geolocation of this installation if the permission is granted.- false : do not report geolocation.Use "auto" and call WonderPush.useGeolocation(true) if you wish to prompt users at a more appropriate time than page load. |
notificationIcon | String | The absolute URL to the notification icon. Prefer https when possible. Displayed in the registration process and push notifications.Defaults to the icon uploaded in your dashboard. |
notificationDefaultUrl | String | The default URL that will be opened when users click on notifications. Defaults to the value chosen in your dashboard. Usually set to the homepage of your website. |
requiresUserConsent | Boolean | Delay the initialization of the SDK until setUserConsent is called. Defaults to false . Set this totrue for GDPR compliance. |
allowedSubscriptionDomains | Array | undefined | Pass an array of external domains you wish to allow subscription from. Pass an empty array to disallow cross-domain subscription altogether. Pass undefined to allow subscription from any domain. The notification domain configured in your dashboard is always allowed. |
subscriptionDialog | Object | See Subscription Dialog Options. |
subscriptionBell | Object | See Subscription Bell Options. |
subscriptionSwitch | Object | See Subscription Switch Options. |
subscriptionNative | Object | See Native Subscription Options |
optInOptions | Object | See Cross-domain Options |
push
push
Pass in a callback and it will be executed when WonderPush is ready to operate. Pass in an array starting with a string and the corresponding function will be called.
WonderPush is ready to use when the async
script tag has been loaded,
and its configuration fetched.
If you've specified requiresUserConsent: true
in your init
, WonderPush will also wait for setUserConsent
to be called with true
.
Callback syntax
This syntax is the most convenient to call multiple WonderPush functions in a single block, and to read their return values.
Please note that this syntax can not be used for functions available prior to initialization such as init
and setUserConsent
.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
// Do something with the WonderPush object.
})
Array syntax
This syntax is more compact when you don't need to read the result of the function. It is also the only option when calling functions available prior to initialization such as init
and setUserConsent
.
// call some function on the WonderPush object when ready
window.WonderPush = window.WonderPush || [];
WonderPush.push(["someFunction", param1, param2]);
Previous SDKs ready
ready
In previous versions of our SDK, push
used to be called ready
. If you're using the deprecated ready
, your app will continue to work.
You should consider upgrading by following the migration instructions.
Subscribing users
subscribeToNotifications
subscribeToNotifications
Subscribes to push notifications.
If you're calling this function from a domain or subdomain that is not the push notification domain as specified in your dashboard, or if you're calling this function from a non-HTTPS page, the registration process must open a pop-up window. To avoid pop-up blockers, specify the event related to the user click as first argument of the subscribeToNotifications
function.
Parameter | Type | Description |
---|---|---|
event | Event | RECOMMENDED. If you call this function in response to a user event, give that event here. |
<a href="#" onclick="WonderPush.subscribeToNotifications(event); return false;">Subscribe to push notifications</a>
In this example we create a link that triggers the push notification subscription process.
unsubscribeFromNotifications
unsubscribeFromNotifications
Unsubscribes from push notifications.
Please note that calling this function does not affect the push notification permission.
<a href="#" onclick="WonderPush.unsubscribeFromNotifications(); return false;">Unsubscribe from push notifications</a>
isSubscribedToNotifications
isSubscribedToNotifications
Returns a Promise
object that resolves to a boolean
indicating whether the user is subscribed to push notifications.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.isSubscribedToNotifications().then(function (isSubscribed) {
console.log("is user subscribed to notifications: ", isSubscribed);
});
});
In this example, we wait for WonderPush to be ready with the push
function, then we call isSubscribedToNotifications
and log the result to the console once the Promise
resolves.
sendSelfNotification
sendSelfNotification
Request the WonderPush servers to send a notification back to the calling user.
If called with no argument, a default testing message is used.
Parameter | Type | Description |
---|---|---|
options | Object | Optional options for controlling which message to send back to the calling user. |
This method works like a stripped down version of the POST /v1/deliveries
endpoint of the Management API.
The allowed options, all optional, are:
Option | Type | Description |
---|---|---|
campaignId | String | The campaign identifier of the notification to trigger. If notification is not given, the notification composed inside that campaign will be used.Otherwise, the given notification is used, but the statistics will still be attached to the given campaign. |
notificationId | String | The notification identifier within the given campaign. Used to choose among multiple A/B variants. |
notification | Object See The Notification object for more information on accepted values. | The notification to deliver. If campaignId is given, this parameter is optional. If given, this parameter will replace the notification composed in the campaign. If you want to control which message to send from the client side, then this is the parameter you are looking for. |
notificationOverride | Object See The Notification object for more information on accepted values. | Applies partial modifications to the notification taken from the campaign. This contrasts from the notification parameter that replaces the notification altogether. |
notificationParams | Object or array of a single object | Fills any notification parameter with the given values. If using the array syntax, it must hold a single object as value. |
Typical usage includes:
- Testing proper configuration, when called with no parameter.
- Manually triggering a notification directly from the user's device, using texts generated from the website itself, when using the
notification
option. - Manually triggering a notification directly from the user's device, using texts entered in the dashboard, when using the
campaignId
option. - Manually triggering a notification directly from the user's device, using texts entered in the dashboard, while filling some parameters from the website itself, when using the
campaignId
andnotificationParams
options.
setUserId
setUserId
Sets the user ID to the provided value. When the user ID changes, a new installation is created. See User IDs for more on using your own user IDs.
window.WonderPush = window.WonderPush || [];
WonderPush.push(['setUserId', "ADAPT_THIS_USER_ID"]);
unsetUserId
unsetUserId
Clears out the userId. Equivalent to setUserId(null)
.
window.WonderPush = window.WonderPush || [];
WonderPush.push(['unsetUserId']);
getUserId
getUserId
Returns a Promise resolving to the current user ID or null
.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.getUserId().then(console.log);
});
getInstallationId
getInstallationId
Returns a Promise resolving to the current installation ID or null
.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.getInstallationId().then(console.log);
});
Native Subscription Options
These initialization options can be passed to init under the subscriptionNative
field, to trigger the native subscription dialog of your web browser.
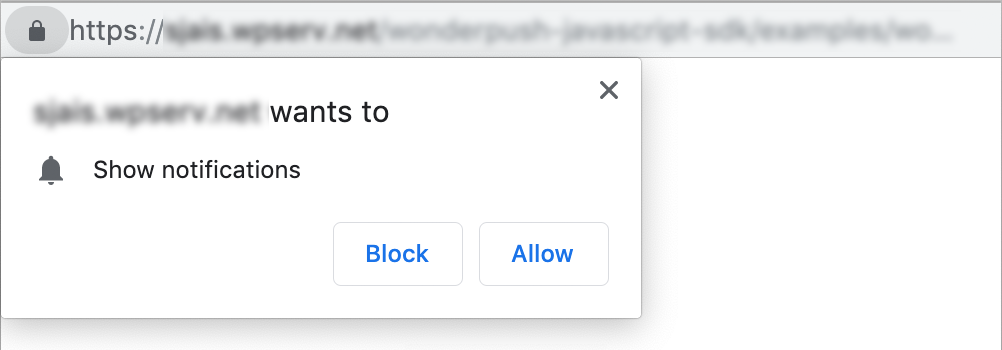
Option | Type | Description |
---|---|---|
triggers | Object | Options to automatically show the native subscription dialog. See Trigger Options. |
User Interfaces
showSubscriptionDialog
showSubscriptionDialog
Show the subscription dialog as configured by the subscriptionDialog
init options.
The subscription dialog is shown from the top of the window and looks like this:
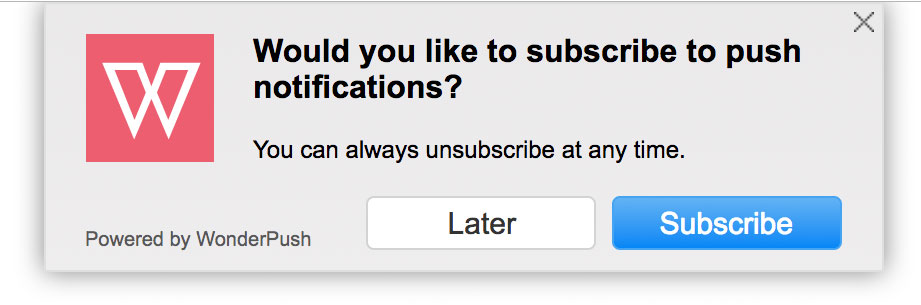
Subscription Dialog Options
These initialization options must be placed within your call to init
, inside the subscriptionDialog
field.
Option | Type | Description |
---|---|---|
triggers | Object | Options to automatically show the subscription dialog. See Trigger Options. |
title | String | Title of the dialog. Defaults “Would you like to subscribe to push notifications?” |
message | String | Message displayed in the dialog. Defaults to “You can always unsubscribe at any time.”. |
positiveButton | String | Label of the button that triggers the subscription process. Defaults to “Subscribe”. |
negativeButton | String | Label of the button that closes the dialog. Defaults to “Later”. |
style | Object | An object containing CSS properties, applied to the dialog top level element style property. Use camel-case property names. For example: { backgroundColor: 'white' } .You can also add any CSS rules in your pages targeting the wp-optin-dialog-* classes. Use your browser inspector to experiment and guide you. |
positiveButtonBackgroundColor | String | The hex color code of the positive button. |
negativeButtonBackgroundColor | String | The hex color code of the negative button. |
positiveButtonTextColor | String | The hex color code of the positive button. |
negativeButtonTextColor | String | The hex color code of the negative button. |
backgroundColor | String | The hex color code of the dialog background. |
textColor | String | The hex color code of the dialog text. |
icon | String | URL to the icon file. Prefer https . Defaults to the icon configured in your dashboard. |
hidePoweredBy | Boolean | Hides the “Powered by WonderPush” mention. Defaults to false. |
closeSnooze | Number or Boolean | How long to force to wait before presenting the dialog again, if the user clicks the close button. Use a duration in milliseconds, false to not set any extra snooze, true to never present again.Defaults to false , which relies on the SDK's default trigger snooze of 12 hours, without forcing extra snooze. |
negativeSnooze | Number or Boolean | How long to force to wait before presenting the dialog again, if the user clicks the negative button. Use a duration in milliseconds, false to not set any extra snooze, true to never present again.Defaults to 604800 , 7 days. |
For examplem this corresponds to the default options:
window.WonderPush = window.WonderPush || [];
WonderPush.push(["init", {
// ... other options
subscriptionDialog: {
triggers: {},
title: "Would you like to subscribe to push notifications?",
message: "You can always unsubscribe at any time.",
positiveButton: "Subscribe",
negativeButton: "Later",
},
}]);
Trigger Options
Trigger options are used to trigger behaviors based on the navigation of the user. Trigger options can be combined to create complex behaviors.
For example:
window.WonderPush = window.WonderPush || [];
WonderPush.push(["init", {
// ... other options
subscriptionDialog: {
triggers: {
minPages: 3,
snooze: 86400000, // 24 hours
}
},
}]);
In this example, the subscription dialog automatically appears after 3 page views. If the user closes or dismiss the dialog, the dialog will appear again after the user performed 3 more page views and 24 hours have elapsed.
Trigger options are:
Option | Type | Description |
---|---|---|
minVisits | Number | How many visits should the user have performed before triggering automatic behavior. |
minPages | Number | How many pages should the user have viewed before triggering automatic behavior. |
minVisitPages | Number | How many pages should the user have viewed within its current visit before triggering automatic behavior. |
delay | Number | How much time (in milliseconds) should the automatic behavior be delayed from page load. |
snooze | Number | How long to wait before automatically triggering the behavior again, in milliseconds. Defaults to 43200000 (12 hours). For example, a value of 3600000 ensures the dialog doesn't appear more than once every hour. |
manual | Boolean | Setting this value to true completely disables the automatic behavior. Use in conjunction with showSubscriptionDialog to show the dialog at a time of your choosing. Defaults to false . |
showSubscriptionBell
showSubscriptionBell
Show or hide the subscription bell as configured by the subscriptionBell
init options. The subscription bell sits at the bottom left or right corner of the window and can be used to give users a permanent access to subscribe to notifications, unsubscribe, and manage their private data
The subscription bell looks like this:
Parameter | Type | Description |
---|---|---|
show | Boolean | Whether to show the subscription bell. |
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
// Hide the subscription bell
WonderPush.showSubscriptionBell(false);
});
<!-- A button that shows the subscription bell -->
<a href="#"
onclick="WonderPush.showSubscriptionBell(true); return false;"
>Show subscription bell</a>
Subscription Bell Options
These initialization options must be specified within your call to init
, inside the subscriptionBell
field.
Option | Type | Description |
---|---|---|
hideWhenSubscribed | Boolean | Hide the bell when the user is subscribed. Defaults to false . |
style | Object | An object containing CSS properties, applied to the dialog top level element style property. Use camel-case property names. For example: { backgroundColor: "white" } . |
cssPrefix | String | A prefix to be used in front of all CSS classes. Use this to reset our styles and put your own. Defaults to "wonderpush-" . |
color | String | Main color of the widget. Defaults to "#ff6f61" . |
position | String | Acceptable values are "left" or "right" . Defaults to "left" . |
bellIcon | String | URL of the bell icon. Prefer https . |
dialogTitle | String | Title of the settings dialog. Defaults to "Manage Notifications" . |
subscribeButtonTitle | String | Title of the subscribe button. Defaults to "Subscribe" . |
unsubscribeButtonTitle | String | Title of the subscribe button. Defaults to "Unsubscribe" . |
notificationIcon | String | Url of the mock notification icon in the settings dialog. Defaults to your app's notification icon as specified in your dashboard. Using https:// is strongly recommended. |
subscribeInviteText | String | Text displayed to invite user to subscribe. Defaults to "Click to subscribe to notifications" . |
alreadySubscribedText | String | Text displayed when already subscribed users hover the notification bell. Defaults to "You're subscribed to notifications" . |
alreadyUnsubscribedText | String | Text displayed when unsubscribed users hover the notification bell. Defaults to "You are not receiving any notifications" . |
blockedText | String | Text displayed when already users have blocked notifications. Defaults to "You've blocked notifications" . |
subscribedText | String | Text displayed when users subscribe. Defaults to "Thanks for subscribing!" . |
unsubscribedText | String | Text displayed when users unsubscribe. Defaults to "You won't receive more notifications" . |
advancedSettingsDescription | String | Text displayed above advanced settings. Defaults to "Your personal notification data:" . |
advancedSettingsFineprint | String | Text displayed below advanced settings. Defaults to "WonderPush fully supports european GDPR" . |
downloadDataButtonTitle | String | Title of the download button. Defaults to "Download" . |
clearDataButtonTitle | String | Title of the clear button. Defaults to "Clear" . |
hidePrivacySettings | Boolean | By default, subscribed users see a Settings button giving them access to privacy settings. When hidePrivacySettings is specified, the settings button is hidden. Defaults to false . |
showBadge | Boolean | When true, a badge with an unread count of "1" will be displayed the first time users see the bell. Defaults to true . |
showSubscriptionSwitch
showSubscriptionSwitch
Appends a subscription switch dynamically to the provided parentNode
, typically used for Single Page Applications.
WonderPush = window.WonderPush || [];
WonderPush.push(function() {
var parentNode = document.getElementById('theIdOfSomeElementYouWantToAddASwitchTo');
WonderPush.showSubscriptionSwitch(parentNode);
});
The subscription switch allows users to subscribe to notifications and unsubscribe.
An alternative to using showSubscriptionSwitch
is to create an element with id wonderpush-subscription-switch
. At page load time, WonderPush will turn this element into a subscription switch. Most of the options below can be specified directly on the wonderpush-subscription-switch
element itself via data-
attributes:
<div id="wonderpush-subscription-switch"
data-sentence="Subscribe to notifications: "></div>
The subscription switch looks like this:
Parameter | Type | Description |
---|---|---|
parentNode | HTMLElement | The node where we'll put the subscription switch using appendChild . |
options | Object | Customize the appearance of the subscription switch. See subscriptionSwitch init options. |
Subscription Switch Options
Most of these initialization options can be specified within your call to init
inside the subscriptionSwitch
field, or directly in your call to showSubscriptionSwitch
, to the exception of switchElementId
that can only be specified at init time.
Option | Type | Description |
---|---|---|
switchElementId | String | The id of the placeholder element this the SDK will use to flesh out a subscription switch. Defaults to wonderpush-subscription-switch . This option cannot be overridden from the placeholder element. |
classPrefix | String | The prefix to prepend to all the CSS classes names used. Defaults to wp- . |
prepend | String | Optional HTML code to inject before the actual switch element. |
append | String | Optional HTML code to inject after the actual switch element. |
sentence | String | HTML snippet to inject in a SPAN tag right before the switch. |
cssClass | String | CSS class to add to the switch element. |
on | String | Label of the switch in the ON state. |
off | String | Label of the switch in the OFF state. |
colorOn | String | Name of a predefined color to use for the ON state. Valid values are blue and green . Defaults to blue . |
colorOff | String | Name of a predefined color to use for the OFF state. Valid values are gray , grey or red . Defaults to gray . |
showTagSwitches
showTagSwitches
Appends a collection of tag switches to the provided parentNode
.
The tag switches allow users to subscribe to notifications and tag themselves at the same time. You can use tag switches to let users subscribe to topics of their choice for example.
An alternative to using showTagSwitches
is to create an element with class wonderpush-tag-switch
. At page load time, WonderPush will turn this element into a tag switch. Most of the options below can be specified directly on the wonderpush-tag-switch
element itself via data-
attributes:
<script>
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.showTagSwitches(document.body, [
{sentence: 'Sports: ', tag: 'sports'},
]);
});
</script>
Would be equivalent to:
<div class="wonderpush-tag-switch"
data-sentence="Sports: "
data-tag="sports"></div>
And would render something like this:
Parameter | Type | Description |
---|---|---|
parentNode | HTMLElement | The node where we'll put the tag switches using appendChild . |
options | Array | Specify the switches. See tagSwitch options. |
Tag Switch Options
* Either the tag
or the field
together with value
options are mandatory. They define what tag is manipulated, or what value is to be put or removed and into which property field, when the switch is flipped.
Option | Type | Description |
---|---|---|
tag | String | The name of the tag to manipulate. Either this option or both field and value must be given. |
field | String | The name of the property to manipulate. Either this option along with value , or tag must be given, |
value | String | The value to manipulate in the field -named property. Either this option along with field , or tag must be given, |
classPrefix | String | The prefix to prepend to all the CSS classes names used. Defaults to wp-tag- . |
prepend | String | Optional HTML code to inject before the actual switch element. |
append | String | Optional HTML code to inject after the actual switch element. |
sentence | String | HTML snippet to inject in a SPAN tag right before the switch. |
cssClass | String | CSS class to add to the switch element. |
on | String | Label of the switch in the ON state. |
off | String | Label of the switch in the OFF state. |
colorOn | String | Name of a predefined color to use for the ON state. Valid values are blue and green . Defaults to blue . |
colorOff | String | Name of a predefined color to use for the OFF state. Valid values are gray , grey or red . Defaults to gray . |
Cross-domain popup window
When subscribing users from another domain than the one configured in your dashboard, a pop-up window will appear. This process is called cross-domain subscription.
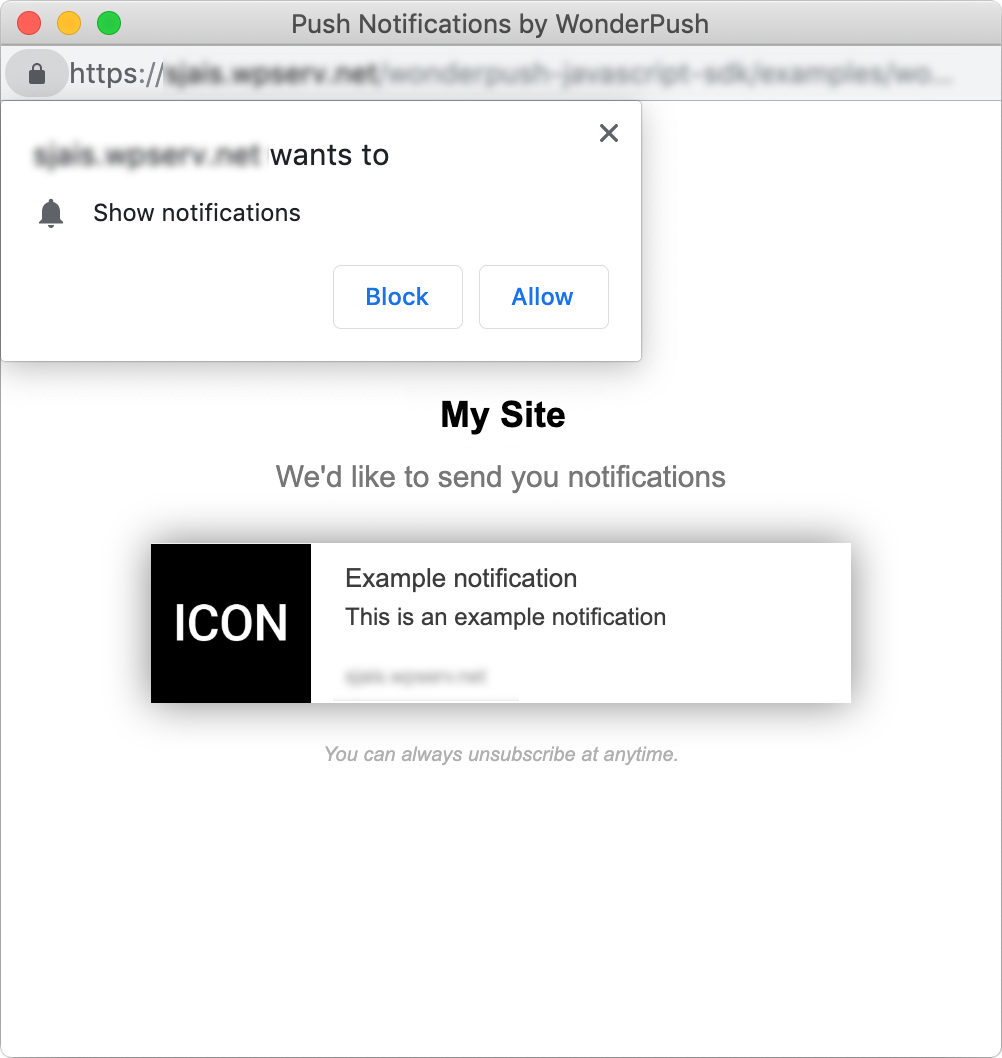
Elements of this popup window are configurable via the Cross-domain options below.
Cross-domain Options
These options control the texts displayed by the cross-domain popup window.
Option | Type | Description |
---|---|---|
externalBoxMessage | String | The main message below your website name. Defaults to “We'd like to send you notifications”. |
externalBoxExampleTitle | String | The title of the example notification. Defaults to “Example notification”. |
externalBoxExampleMessage | String | The message of the example notification. Defaults to “This is an example notification”. |
externalBoxDisclaimer | String | The fineprint displayed below the example notification. Defaults to “You can always unsubscribe at anytime.”. |
externalBoxProcessingMessage | String | The message written when user accepts and subscription is in progress. Defaults to “Subscribing...”. |
externalBoxSuccessMessage | String | The message displayed when subscription is successful. Defaults to “Thanks for subscribing!”. |
externalBoxFailureMessage | String | The default error message when subscription fails. Defaults to “Sorry, something went wrong.”. |
externalBoxTooLongHint | String | The error message displayed when subscribing takes too long. Defaults to “Poor connection or private browsing?”. |
positiveButtonText | String | The text of the subscription button (shown on Firefox only) |
negativeButtonText | String | The text of the cancel button (shown on Firefox only) |
For example, this corresponds to the default values:
window.WonderPush = window.WonderPush || [];
WonderPush.push(["init", {
// ... other options
optInOptions: {
externalBoxMessage: "We'd like to send you notifications",
externalBoxExampleTitle: "Example notification",
externalBoxExampleMessage: "This is an example notification",
externalBoxDisclaimer: "You can always unsubscribe at anytime.",
externalBoxProcessingMessage: "Subscribing...",
externalBoxSuccessMessage: "Thanks for subscribing!",
externalBoxFailureMessage: "Sorry, something went wrong.",
externalBoxTooLongHint: "Poor connection or private browsing?",
externalBoxCloseHint: "Close",
positiveButtonText: "Subscribe",
negativeButtonText: "Later",
},
}]);
Segmentation
Segmentation functions allow you to mark installations so they can be added to segments and you can send them targeted notifications later.
There are many ways of performing segmentation:
Tags are like labels you can stick on users. Use tags to create segments in your dashboard and send targeted push notifications. Example: all users that have the "customer" tag.
Events have a date, a type
of your choice and attributes
. Use events to create segments in your dashboard and send targeted push notifications. Example: all users that purchased in the last hour is a typical event-based segment.
Installation properties represent traits of the user. That's a good place to store age, gender and other data traditionally used for segmentation. Create property-based segments in your dashboard. Example: all users above 18 is a typical property-based segment.
trackEvent
trackEvent
Tracks a custom event of your choice. E.g. purchase
.
Parameter | Type | Description |
---|---|---|
type | String | Required The type of the event to track. Event names starting with @ are reserved for internal use and cannot be used here. |
attributes | Object | Attributes associated with this event. See format of property names for detailed syntax. |
Example:
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.trackEvent('purchase', {
string_product: "Some product",
float_price: 1.99,
});
});
addTag
addTag
Adds one or more tags to the current installation.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.addTag("customer");
WonderPush.addTag("economics", "sport", "politics");
});
removeTag
removeTag
Removes one or more tags from the current installation.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.removeTag("customer");
WonderPush.removeTag("economics", "sport", "politics");
});
removeAllTags
removeAllTags
Removes all tags from the current installation.
hasTag
hasTag
Tests whether a given tag is attached to the current installation.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.hasTag("customer").then(function(isCustomer) {
if (isCustomer) {
// Display paid features
}
});
});
getTags
getTags
Returns all the tags attached to the current installation as a promise resolving to an array of strings.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.getTags().then(function(tags) {
// Work on the tags variable
});
});
getPropertyValue
getPropertyValue
Returns a Promise
that resolves to the value of a given property associated to the current installation.
If the property stores an array, only the first value is returned. This way you don't have to deal with potential arrays if that property is not supposed to hold one. Returns null
if the property is absent or has an empty array value.
Parameter | Type | Description |
---|---|---|
field | String | The name of the property to read values from. |
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.getPropertyValue("string_lastname").then(function(lastName) {
// Use lastName
});
});
getPropertyValues
getPropertyValues
Returns a Promise
that resolves to an array of the values of a given property associated to the current installation.
If the property does not store an array, an array is returned nevertheless. This way you don't have to deal with potential scalar values if that property is supposed to hold an array. Returns an empty array instead of null
if the property is absent. Returns an array wrapping any scalar value held by the property.
Parameter | Type | Description |
---|---|---|
field | String | The name of the property to read values from. |
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.getPropertyValues("string_favorityPlayers").then(function(favoritePlayers) {
// Use the favoritePlayers array
});
});
addProperty
addProperty
Adds the value to a given property associated to the current installation.
The stored value is made an array if not already one. If the given value is an array, all its values are added. If a value is already present in the stored value, it won't be added.
Parameter | Type | Description |
---|---|---|
field | String | The name of the property to add values to. See format of property names for detailed syntax. |
value | any, any[], any... | The value(s) to be added. Can be an array, or multiple arguments. |
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
// You can add a single value
WonderPush.addProperty("string_interests", "sport");
// You can add multiple values
WonderPush.addProperty("string_interests", "sport", "entertainment");
// You can add an array of values
WonderPush.addProperty("string_interests", ["sport", "entertainment"]);
});
removeProperty
removeProperty
Removes the value from a given property associated to the current installation.
The stored value is made an array if not already one. If the given value is an array, all its values are removed. If a value is present multiple times in the stored value, they will all be removed.
Parameter | Type | Description |
---|---|---|
field | String | The name of the property to remove values from. |
value | any, any[], any... | The value(s) to be removed. Can be an array, or multiple arguments. |
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
// You can remove a single value
WonderPush.removeProperty("string_interests", "sport");
// You can remove multiple values
WonderPush.removeProperty("string_interests", "sport", "entertainment");
// You can remove an array of values
WonderPush.removeProperty("string_interests", ["sport", "entertainment"]);
});
setProperty
setProperty
Sets the value to a given property associated to the current installation.
The previous value is replaced entirely. Setting undefined
or null
has the same effect as unsetProperty.
Parameter | Type | Description |
---|---|---|
field | String | The name of the property to set. See format of property names for detailed syntax. |
value | any, any[] | The value to be set. Can be an array. |
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
// You can set a single value
WonderPush.setProperty("bool_isCustomer", true);
// You can remove a field using null or undefined
WonderPush.setProperty("int_age", null);
// You can set an array of values
WonderPush.setProperty("string_interests", ["sport", "entertainment"]);
});
unsetProperty
unsetProperty
Removes the value of a given property associated to the current installation.
The previous value is replaced with null
.
Parameter | Type | Description |
---|---|---|
field | String | The name of the property to unset. |
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.unsetProperty("string_favoritePlayers");
});
putProperties
putProperties
Updates the properties of the current installation. Omitting a previously set property leaves it untouched. To remove a property, you must pass it explicitly with a value of null
.
Parameter | Type | Description |
---|---|---|
properties | Object | Properties to add or update. See format of property names for detailed syntax. |
Example:
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
// Puts the int_age property with a value of 34 in the installation
WonderPush.putProperties({
int_age: 34
});
});
getProperties
getProperties
Returns a Promise
that resolves to an Object containing the properties of the current installation.
Example:
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.getProperties().then(function(properties) {
console.log("Properties of the current installation are:", properties);
});
});
useGeolocation
useGeolocation
Attach GeoLocation position to this installation.
Parameter | Type | Description |
---|---|---|
enable | "auto" or Boolean | Possible values are: - "auto" : watch and report position to this installation if the permission is already granted, but never prompt for the GeoLocation permission.- true : prompt the user at page load if necessary, watch and report position to the installation if the permission is granted.- false (default): do not collect or report GeoLocation position.See the geolocation initialization option to prompt users at page load. |
getCountry
getCountry
Returns the user's country, either as previously stored, or undefined
.
setCountry
setCountry
Overrides the user's country.
Defaults to getting the country code from the system default locale.
You should use an ISO 3166-1 alpha-2 country code, eg: US
, FR
, GB
.
Use null
to disable the override.
getCurrency
getCurrency
Returns the user's currency, either as previously stored, or undefined
.
setCurrency
setCurrency
Overrides the user's currency.
Defaults to getting the currency code from the system default locale.
You should use an ISO 4217 currency code, eg: USD
, EUR
, GBP
.
Use null
to disable the override.
getLocale
getLocale
Returns the user's locale, either as previously stored, or as guessed from the system.
setLocale
setLocale
Overrides the user's locale.
Defaults to getting the language and country codes from the system default locale.
You should use an xx-XX
form of RFC 1766, composed of a lowercase ISO 639-1 language code, an underscore or a dash, and an uppercase ISO 3166-1 alpha-2 country code.
Use null
to disable the override.
getTimeZone
getTimeZone
Returns the user's time zone, either as previously stored, or as guessed from the system.
setTimeZone
setTimeZone
Overrides the user's time zone.
Defaults to getting the time zone code from the system default locale.
You should use an IANA time zone database codes, Continent/Country
style preferably like Europe/Paris
, or abbreviations like CET
, PST
, UTC
, which have the drawback of changing on daylight saving transitions.
Use null
to disable the override.
Privacy
setUserConsent
setUserConsent
Sets user privacy consent. You must use push
with the array syntax to call setUserConsent
.
Works in conjunction with the requiresUserConsent
init
option.
When this option is specified, calls to push
are blocked and any method of the SDK results in rejecting Promises until setUserConsent
is called with true
.
Calling setUserConsent
with false
blocks the SDK again.
window.WonderPush = window.WonderPush || [];
WonderPush.push(["setUserConsent", true]);
clearEventsHistory
clearEventsHistory
Instructs to delete any event associated with the all installations present on the device, locally and on WonderPush servers.
clearPreferences
clearPreferences
Instructs to delete any custom data (including installation properties) associated with the all installations present on the device, locally and on WonderPush servers.
clearAllData
clearAllData
Instructs to delete any event, installation and potential user objects associated with all installations present on the device, locally and on WonderPush servers.
downloadAllData
downloadAllData
Initiates the download of all the WonderPush data relative to the current installation, in JSON format.
Custom DOM Events
The WonderPush SDK fires a few custom DOM events on the window
object. The type of event is always WonderPushEvent
and the payload's name
property determines what type of WonderPushEvent is emitted.
window.addEventListener('WonderPushEvent', function(event) {
var eventType = event.detail ? event.detail.name : undefined;
// Do something in response to this eventType
});
subscription
subscription
The event type subscription
allows you to listen to users subscribing and unsubscribing. The value of the event.detail.state
property tells you what just happened. It is WonderPush.SubscriptionState.SUBSCRIBED
when user just subscribed, WonderPush.SubscriptionState.UNSUBSCRIBED
when user just unsubscribed.
This event is fired as soon as the SDK is loaded, to give you the initial state, plus everytime it changes, so you can follow the current state.
For example:
// Store the previous subscription state to observe transitions
var previousWonderPushSubscriptionState;
window.addEventListener('WonderPushEvent', function(event) {
if (!event.detail || event.detail.name !== 'subscription') return;
if (previousWonderPushSubscriptionState === WonderPush.SubscriptionState.NOT_SUBSCRIBED && event.detail.state === WonderPush.SubscriptionState.SUBSCRIBED) {
// Transitioning from not subscribed to subscribed
console.log('User just subscribed!');
} else if (previousWonderPushSubscriptionState === WonderPush.SubscriptionState.UNSUBSCRIBED && event.detail.state === WonderPush.SubscriptionState.SUBSCRIBED) {
// Transitioning from unsubscribed to subscribed
console.log('User just resubscribed!');
} else if (previousWonderPushSubscriptionState === WonderPush.SubscriptionState.SUBSCRIBED && event.detail.state === WonderPush.SubscriptionState.UNSUBSCRIBED) {
// Transitioning from subscribed to unsubscribed
console.log('User just unsubscribed!');
} else if (previousWonderPushSubscriptionState === undefined && event.detail.state === WonderPush.SubscriptionState.SUBSCRIBED) {
// Initial state is subscribed
console.log('User already subscribed!');
} else if (previousWonderPushSubscriptionState === undefined && event.detail.state === WonderPush.SubscriptionState.NOT_SUBSCRIBED) {
// Initial state is not subscribed
console.log('User not subscribed (yet)!');
} else if (previousWonderPushSubscriptionState === undefined && event.detail.state === WonderPush.SubscriptionState.UNSUBSCRIBED) {
// Initial state is unsubscribed
console.log('User already unsubscribed!');
}
previousWonderPushSubscriptionState = event.detail.state;
});
session
session
The event type session
allows you to listen to the SDK creating an installation on WonderPush servers. The value of the event.detail.state
property tells you what just happened. It is WonderPush.SessionState.INIT_SUCCESS
when the installation has just been created.
This event is fired as soon as the SDK is loaded, to give you the initial state, plus everytime it changes, so you can follow the current state.
For instance you can use this to reliably get the installationId whenever it gets known:
new Promise((resolve, reject) => {
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
if (WonderPush.getSessionState() === WonderPush.SessionState.INIT_SUCCESS) {
WonderPush.getInstallationId().then(resolve, reject);
return;
}
var listener = function(event) {
if (event.detail.name !== 'session') return;
if (event.detail.state === WonderPush.SessionState.INIT_SUCCESS) {
window.removeEventListener('WonderPushEvent', listener);
WonderPush.getInstallationId().then(resolve, reject);
};
};
window.addEventListener('WonderPushEvent', listener);
});
}).then(installationId => {
// Do something with the installationId
});
trackEvent
trackEvent
The event type trackEvent
allows you to listen to every event send by the WonderPush SDK and react to it.
window.addEventListener('WonderPushEvent', function(event) {
if (!event.detail || event.detail.name !== 'trackEvent') return;
console.log('WonderPush event tracked:', event.detail.event);
});
Note that the @NOTIFICATION_OPENED
and @NOTIFICATION_RECEIVED
events are only triggered inside the Service Worker. You will not be able to listen for them from your website pages. You need to run this code in your Service Worker file.
Popups (formerly known as In-app messaging)
setInAppMessagingDisplayCallback
setInAppMessagingDisplayCallback
Setting the display callback lets you handle the display of a popup yourself. This method takes a single argument: a function that accepts two arguments:
- the popup to display
- an
InAppMessageReportingCallback
object you must call when the popup is displayed, clicked or dismissed.
Return true
when you wish to handle the display yourself, false
when you want WonderPush to handle the display.
window.WonderPush = window.WonderPush || [];
WonderPush.push(function() {
WonderPush.setInAppMessagingDisplayCallback(function(inApp, reportingCallback) {
// Display popup
// Report popup viewed
// Report any popup clicks
return true; // false if you don't want WonderPush to handle the display
});
});
See handling the display of popups yourself for details on how to implement this.
setInAppMessagesSuppressed
setInAppMessagesSuppressed
Lets you disable or enable popups.
WonderPush.push(function() {
// Disables popups
WonderPush.setInAppMessagesSuppressed(true);
});
getInAppMessagesSuppressed
getInAppMessagesSuppressed
Whether popups are disabled.
Migrating from previous versions of the Website SDK
Previous versions of the Website SDK provided functions called init
and ready
and most other functions had different names.
Migrating to this new API is automatic, you do not need to do anything to call the new functions. The old ones remain available and you can just call the new ones on the WonderPushSDK
object you're used to manipulate.
If you wish to migrate to the new way of doing things, which we recommend, perform all of these steps:
- Incorporate the
async
script tag and snippet - Remove the javascript snippet that looked like
(function(w,d,s,i,n)…
. - Change your call to
init
to use the array syntax ofpush
.
Change:
// DEPRECATED
WonderPush.init({
webKey: "YOUR_WEBKEY",
});
to:
// VALID
window.WonderPush = window.WonderPush || [];
WonderPush.push(["init", {
webKey: "YOUR_WEBKEY",
}]);
- Change your calls to
ready
to use the callback syntax ofpush
. To ease the transition, you can also simply aliasready
topush
like this:
window.WonderPush = window.WonderPush || [];
WonderPush.ready = WonderPush.push;
// You can continue to use `ready` with the same syntax as before
WonderPush.ready(function(WonderPushSDK) {
// Do something with WonderPushSDK, or WonderPush indifferently
});
Updated over 1 year ago