Flutter Push Notifications
How to set up WonderPush push notifications on Flutter cross-platform apps
Install push notifications in Flutter apps in 4 steps.
Setting up push notifications for your Flutter app is easy. Push notifications are the ideal solution to re-engage users and bring them back to your app.
Estimated setup time: 15 minutes.
Prerequisites
You'll need:
- For Android:
- A device or emulator with Google Play services installed and up-to-date.
- A Firebase account.
- Android Studio.
- For iOS:
- XCode
- An iOS device such as an iPhone or an iPad
- An iOS push credentials
If you haven't already, sign up for a free account on wonderpush.com.
To upgrade to the latest version of our SDK, follow these instructions.
Create your project
Click on New Project:
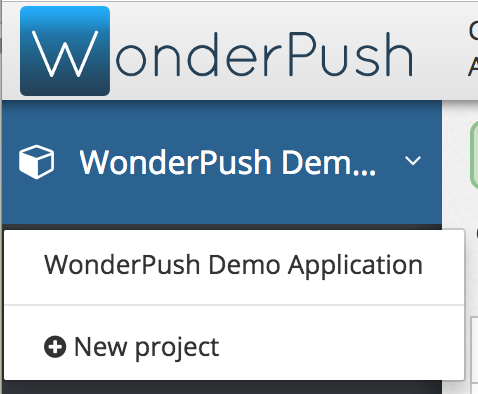
Choose a name for your project and select Android and iOS as platforms then click Create:
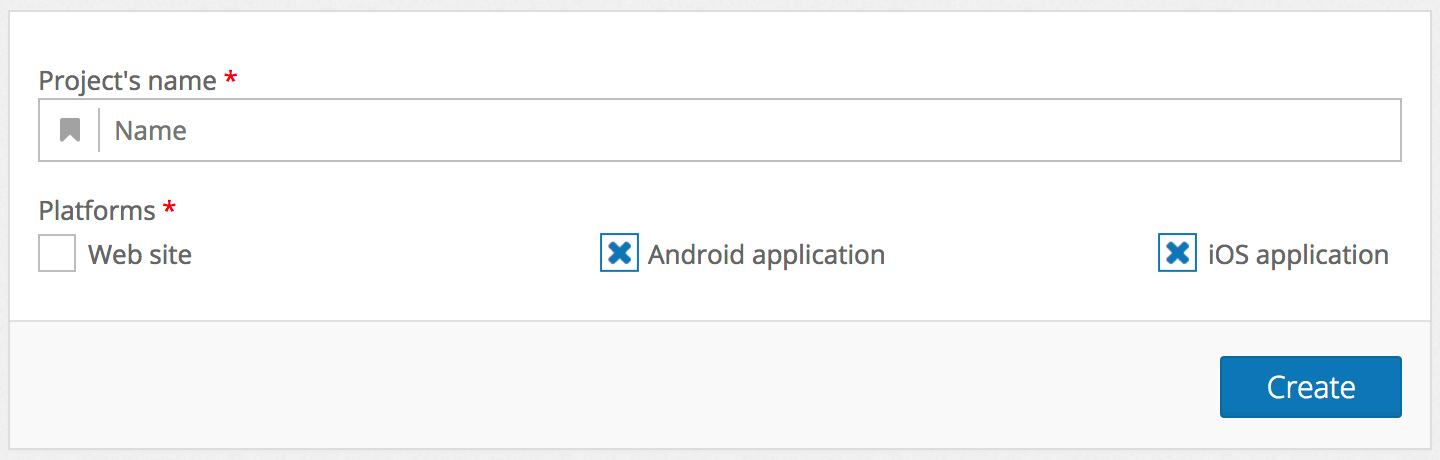
Already have a project?Just add the Android or iOS platforms to any existing project by going to Settings, selecting the first tab named after your project and clicking Edit. You'll be presented a form that lets you add a platform.
Prepare your flutter project
Create your Flutter application if you haven't already, see the Flutter guide and setup it by following given instructions.
Open pubspec.yaml
and add the following two dependencies:
dependencies:
wonderpush_flutter: ^2.0.0
wonderpush_fcm_flutter: ^1.0.1
Also make sure to remove the firebase_messaging
dependency if you have it.
Open a Terminal window in your flutter project directory and type:
flutter pub get
Let's edit lib/main.dart
to trigger a push notification prompt. You'll probably want to move this somewhere more appropriate later.
import 'package:flutter/material.dart';
// Add this line to import WonderPush at the top of the file
import 'package:wonderpush_flutter/wonderpush_flutter.dart';
void main() {
runApp(MyApp());
// Add this line to trigger a push notifications prompt
WonderPush.subscribeToNotifications();
}
Controlling the Android 13+ permission prompt timingIn order to control when the permission prompt is triggered when running on Android 13 devices, you will need to update your
android/app/build.gradle
to use:android { compileSdkVersion 33 defaultConfig { targetSdkVersion 33 } }
If you do not do so, the user will be prompted for permission right at application launch.
Setting up iOS
Step 1. Upload your push credentials
Get your Apple key with the Apple Push Notifications service (APNs) service enabled. You can list your existing keys here. Simply check the Enabled Services and note the Key ID as well as the Team ID displayed below your name in the top-right corner.
If you don't have one, here's how to create one. In a few words, head to the Apple developer website, go to Accounts, then click on Keys in the Certificates, Identifiers & Profiles column, and click the + button on the top left to add a new key.
Check Apple Push Notifications service (APNs), then click Continue:
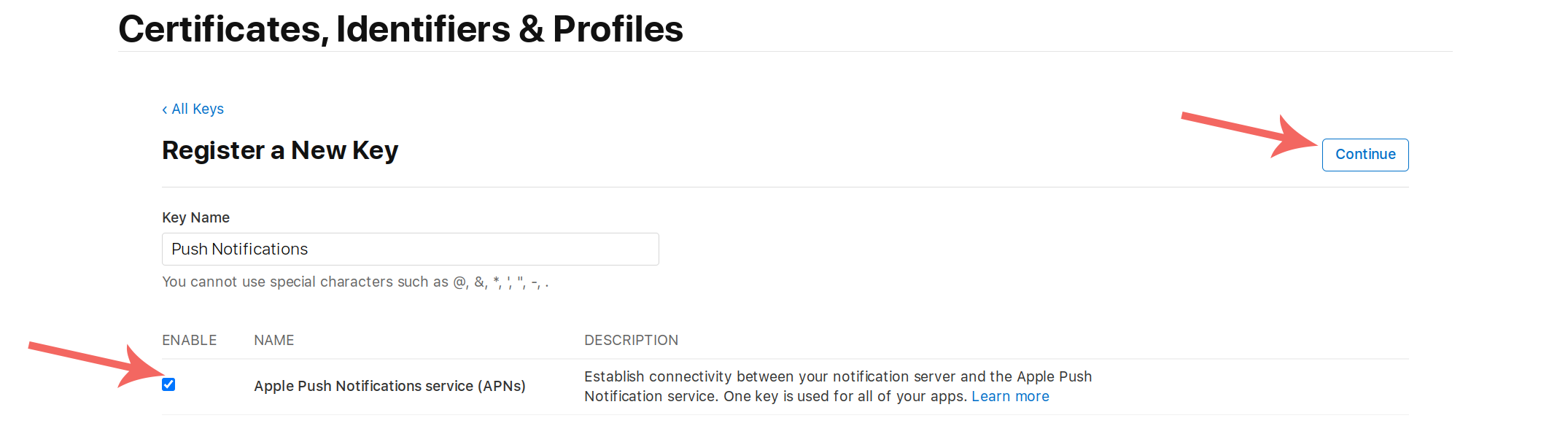
If instead you see the following red message, this means that you should instead reuse an existing key. As Apple will not let you download the key again after its registration, you will need to use for the .p8
file that you downloaded at that time.

How should Apple keys be managed for the Apple Push Notifications service (APNs)Apple imposes a maximum of 2 keys simultaneously valid for the Apple Push Notifications service (APNs). Such keys are valid for all applications (Bundle ID or App ID) on your account (Team ID).
Limiting to 2 keys enables you to have one key used for all your push notification needs across all your WonderPush projects (you'll need to upload the same key for each of your projects) or even push providers, and perform a rolling replacement of your key with no downtime.
You would create a second key, temporarily having 2 valid keys at a time, replace every WonderPush project or services that need to send push notifications, and finally revoke the previous key, leaving you again with a single existing key.
In the next screen click Register.
In the next screen, note your Key ID, and your Team ID in the top right corner.
Then click Download. Please do keep this.p8
file in a secure location (see the above callout about key management.)
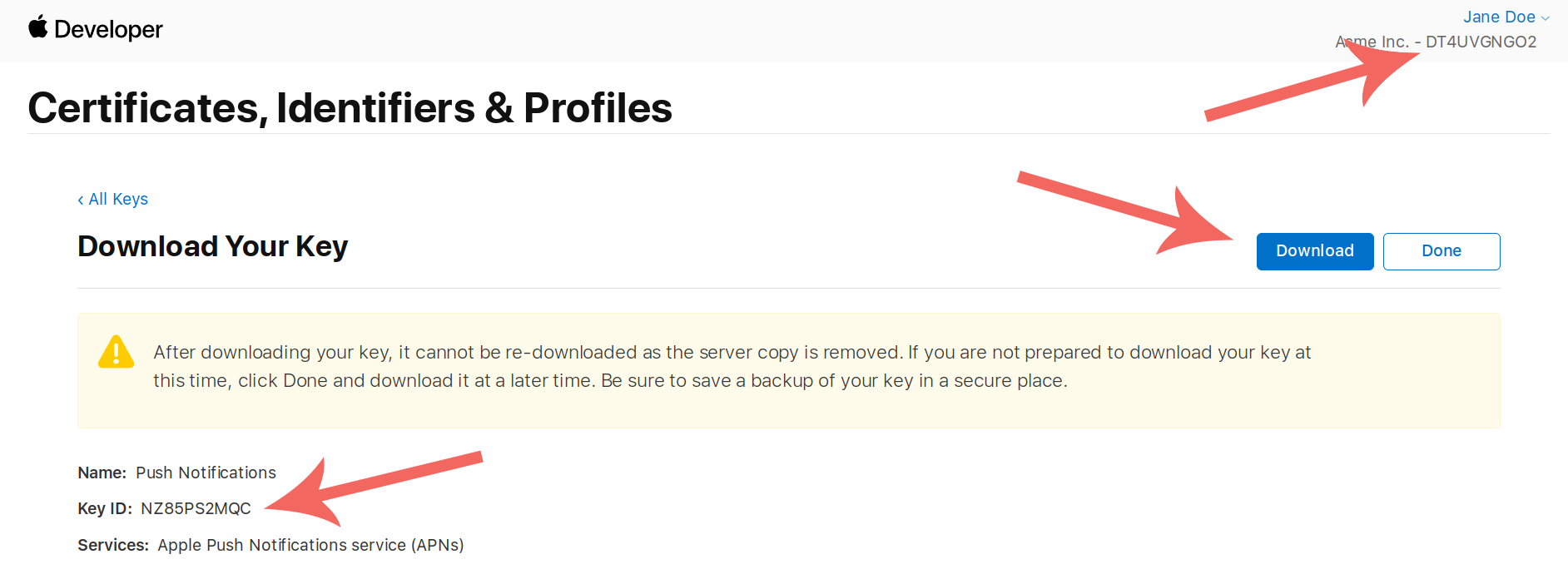
You will then need to know your Bundle ID:
- Open your project in Xcode
- Click on your project in the file browser on the left
- Click on your app target in the list
- Open the General tab
- Your Bundle identifier (aka Bundle ID) is in the Identity section
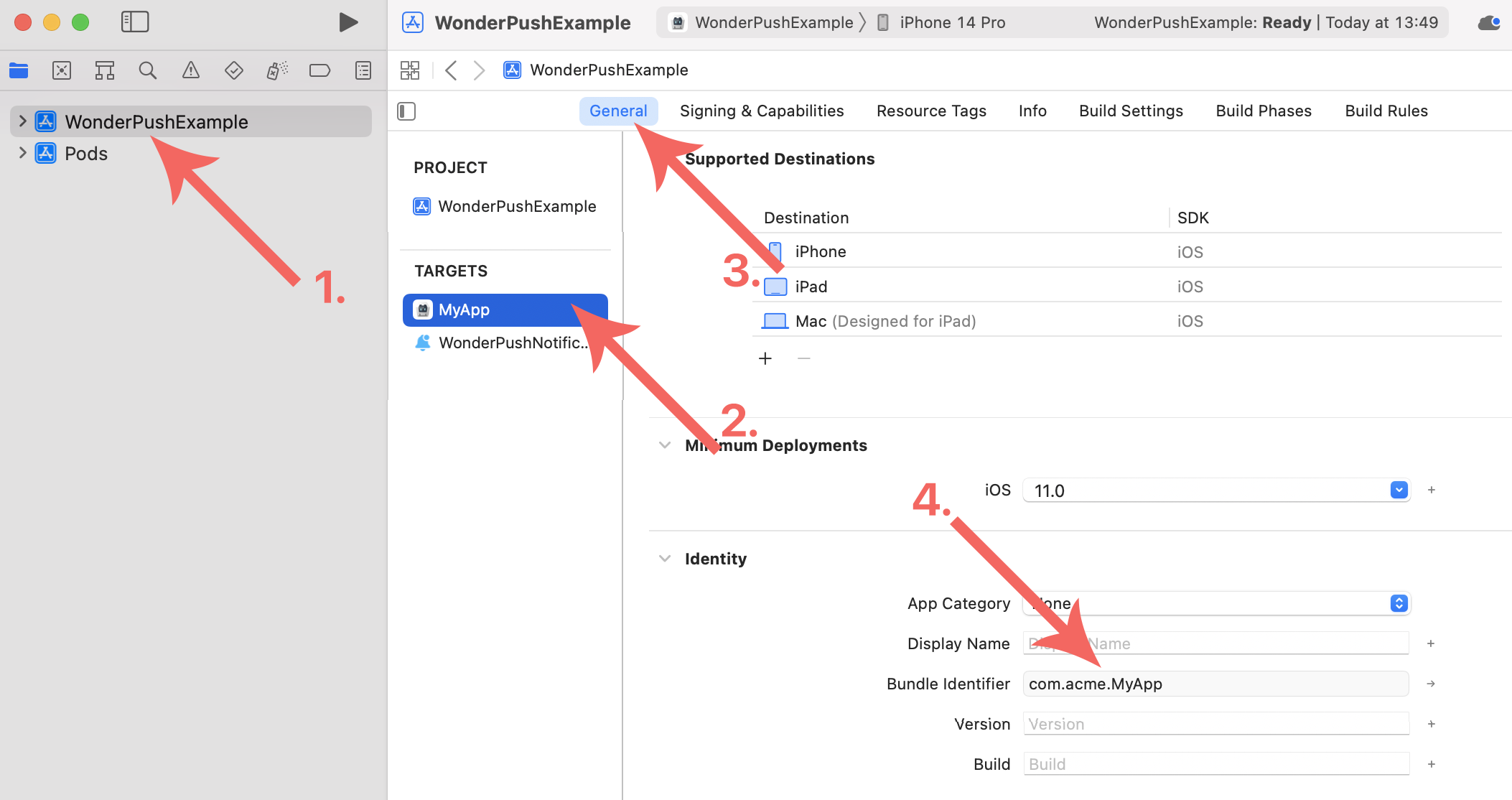
Now go to Settings / Platforms, click the iOS application section title and fill drop your .p8
file:
- Fill the Key ID, Team ID and Bundle ID if necessary. Check their values if they are pre-filled.
- Click Save.
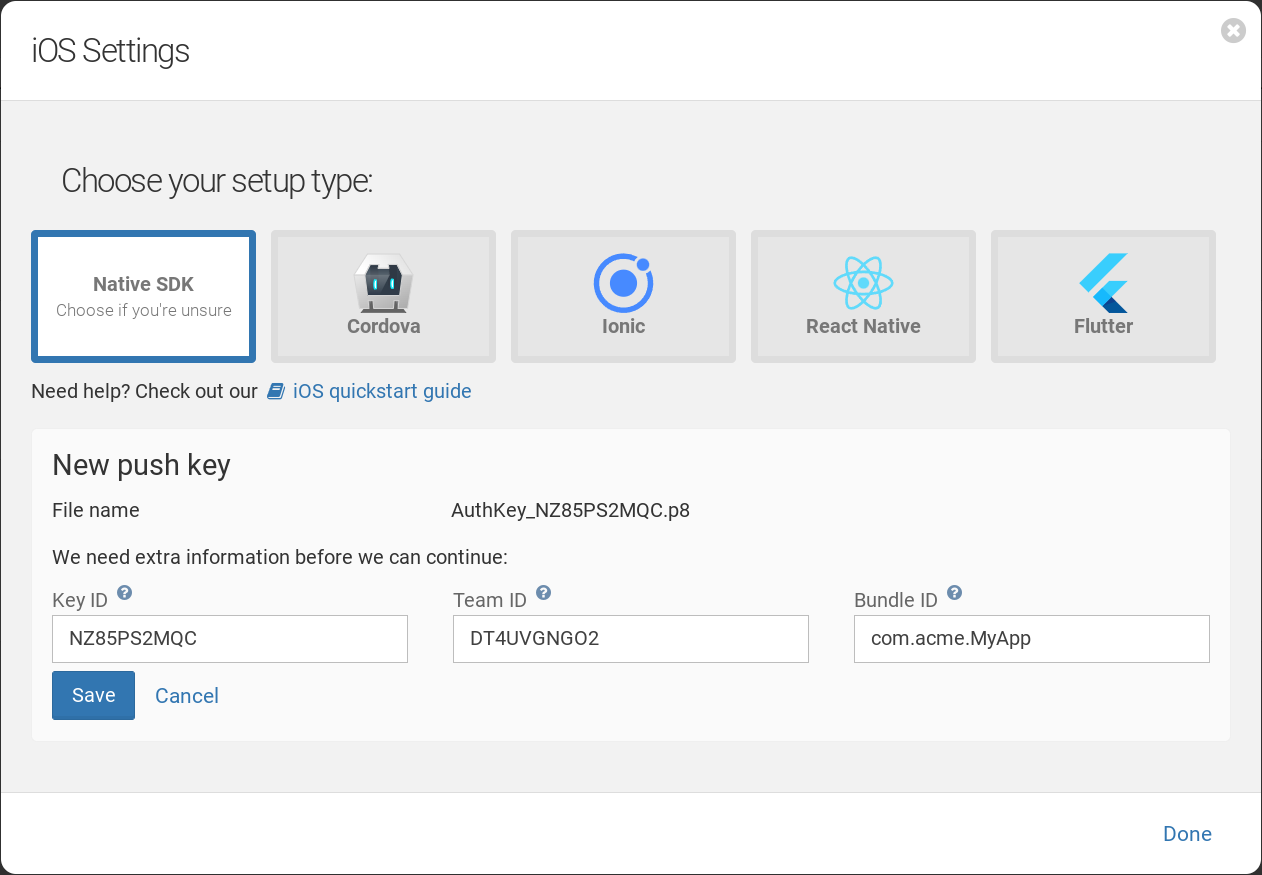
Step 2. Prepare your Xcode project for push notifications
In your flutter project directory, open the file ios/Runner.xcworkspace
with Xcode.
Be sure to open
ios/Runner.xcworkspace
, notios/Runner.xcodeproj
Make sure your deployment target is greater than or equal to iOS 9:
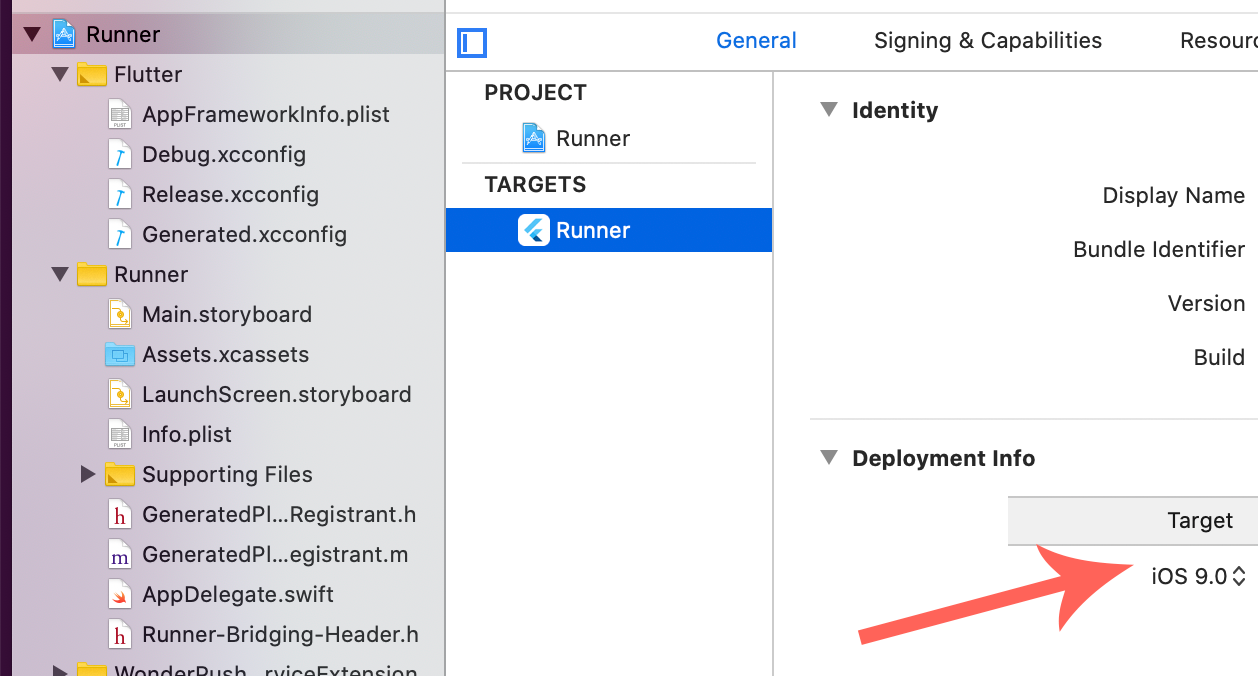
Add the following capabilities:
- Background Modes
- Push Notifications
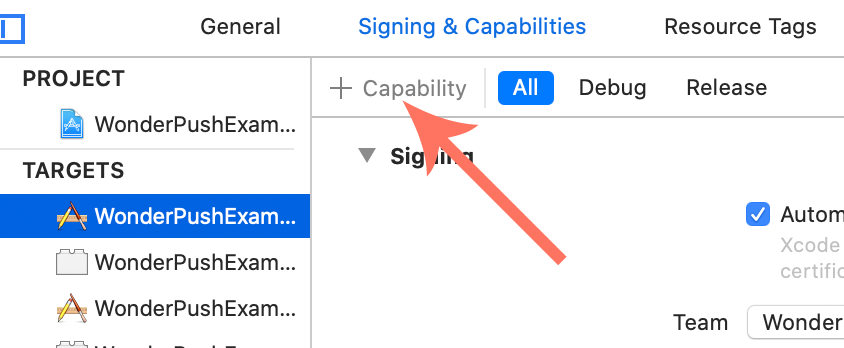
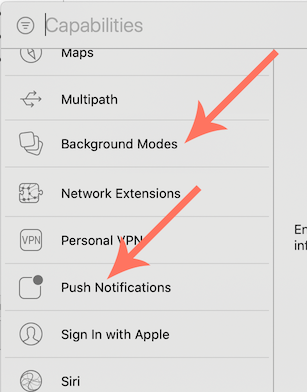
Make sure the Remote notifications background mode is checked and ensure the Push notification capability is present:
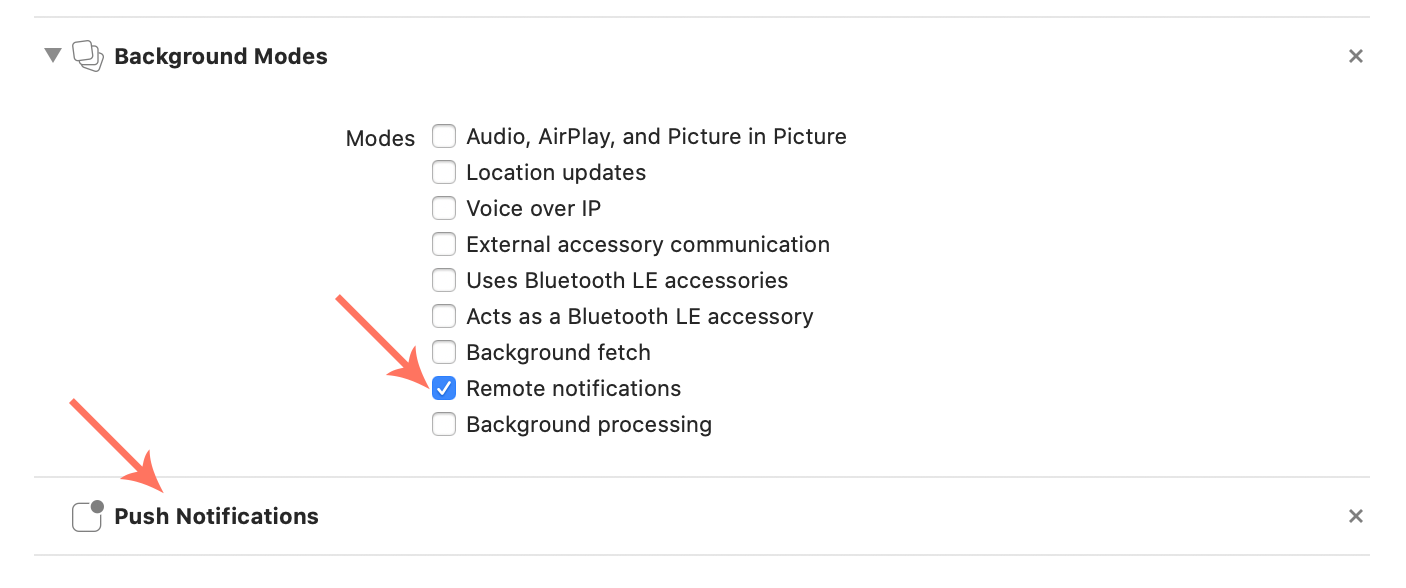
Go to the Settings page of your WonderPush dashboard and take note of your client ID and client secret:
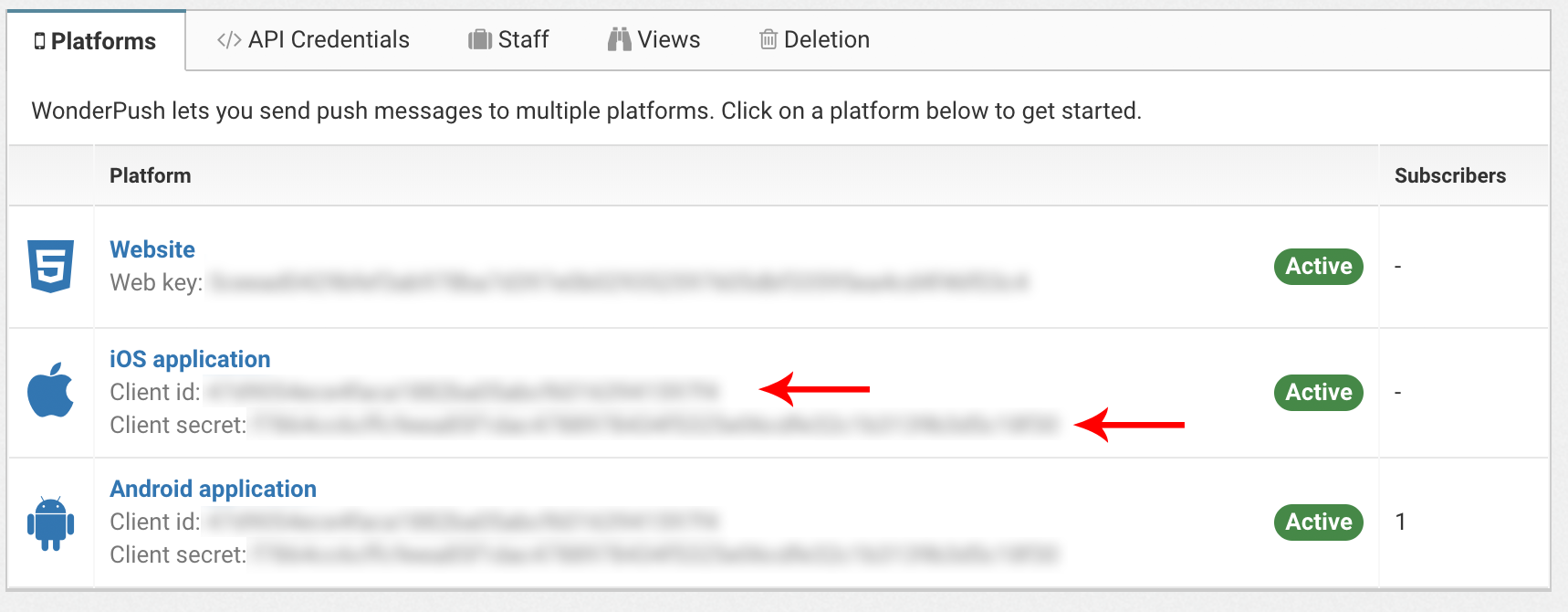
Open your AppDelegate
(usually in ios/Runner/AppDelegate.swift
) and update it to look like the following, adapting YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with the values that can be found on the Platform Settings page of your WonderPush Dashboard:
import UIKit
import Flutter
// Add these 2 lines
import WonderPush
import wonderpush_flutter
@UIApplicationMain
@objc class AppDelegate: FlutterAppDelegate {
// Add the following method
override func application(_ application: UIApplication, willFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
WonderPush.setClientId("YOUR_CLIENT_ID", secret: "YOUR_CLIENT_SECRET")
WonderPush.setupDelegate(for: application)
WonderPushPlugin.prepare()
if #available(iOS 10.0, *) {
WonderPush.setupDelegateForUserNotificationCenter()
}
return super.application(application, willFinishLaunchingWithOptions: launchOptions)
}
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
GeneratedPluginRegistrant.register(with: self)
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
}
// Add this line:
#import <WonderPush/WonderPush.h>
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// ...
[GeneratedPluginRegistrant registerWith: self];
// Add the following 5 lines
[WonderPush setClientId:@"YOUR_CLIENT_ID" secret:@"YOUR_CLIENT_SECRET"];
[WonderPush setupDelegateForApplication:application];
if (@available(iOS 10.0, *)) {
[WonderPush setupDelegateForUserNotificationCenter];
}
return [super application:application didFinishLaunchingWithOptions:launchOptions];
}
If at that stage your project doesn't compile, everything is normal: we'll run
pod install
later in this guide and that should fix it.
Step 3. Create the notification service extension
In Xcode, select File / New / Target..., and choose Notification Service Extension:
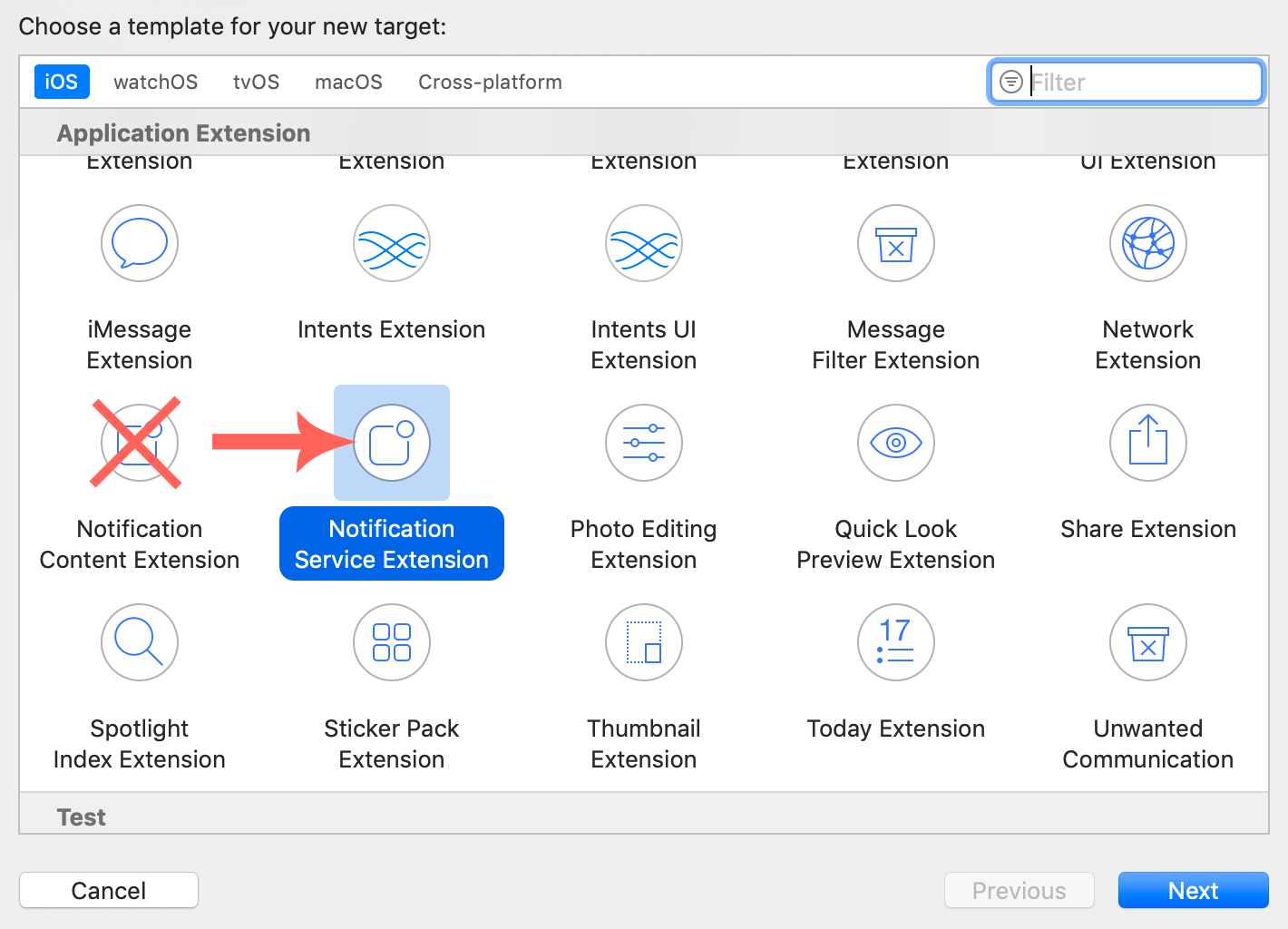
Enter WonderPushNotificationServiceExtension
as the name for your new target:
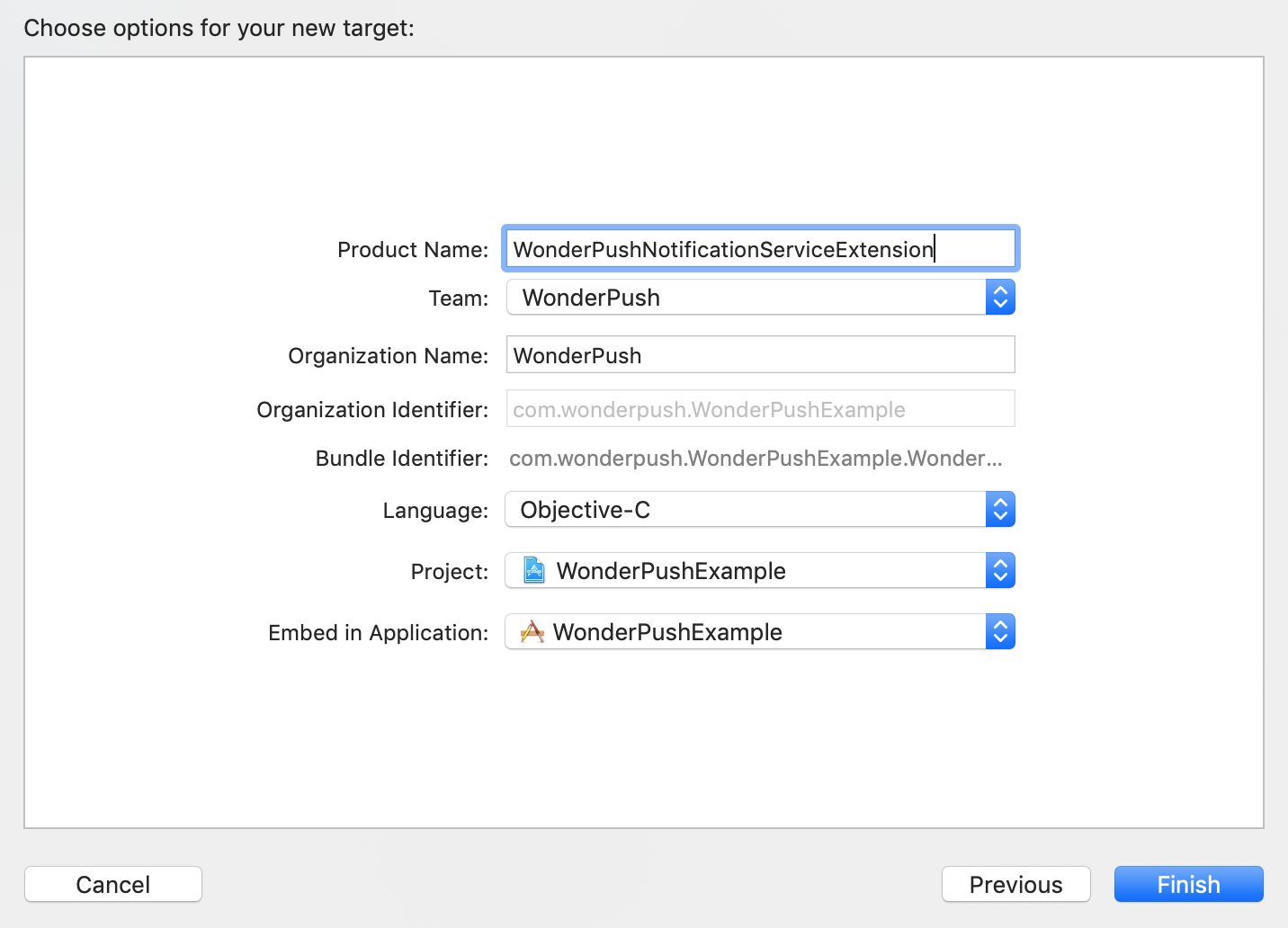
When Xcode prompts you to activate the new scheme, answer Cancel to keep Xcode building and debugging your app instead of the extension:
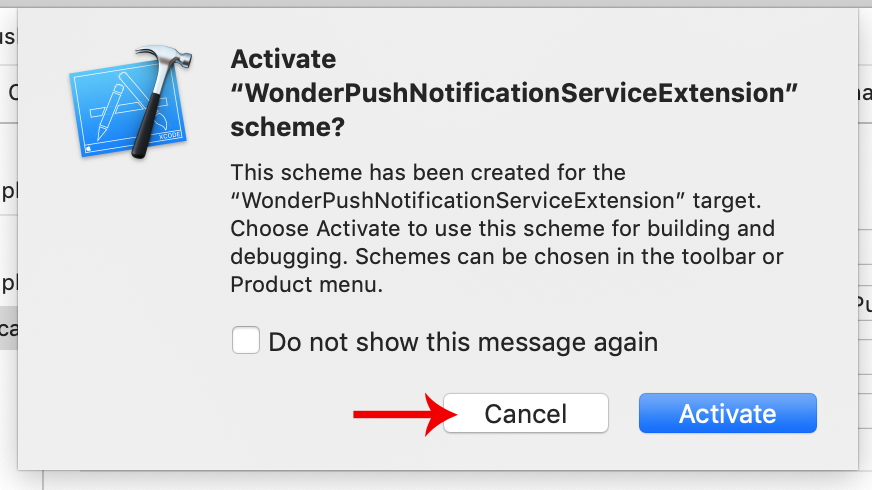
If you activate by mistake, switch back to your app's scheme from the dropdown menu located next to the play button.
Set the Deployment Target of your Notification Service Extension to 11.0
:
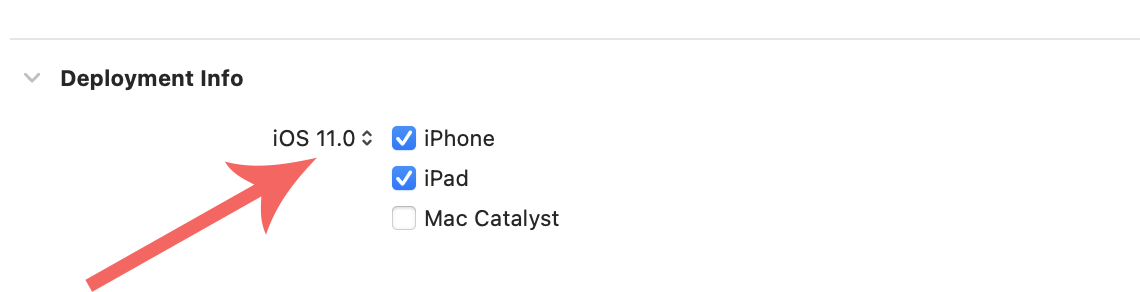
Make sure to use Automatic Signing for the extension target:
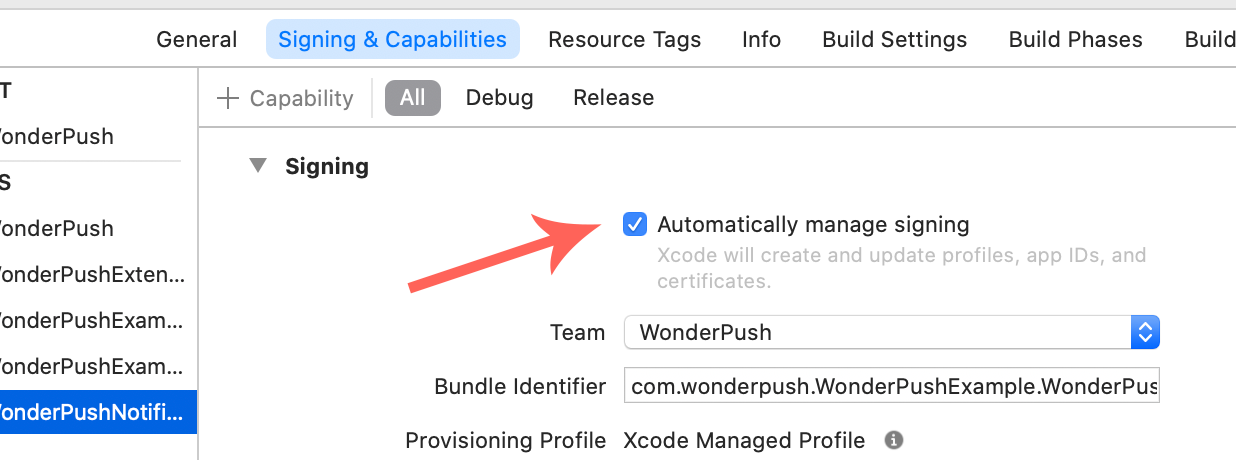
If you're getting the following error, make sure to set up Automatic Signing for the extension:
Provisioning profile "XXX" has app ID "YYY", which does not match the bundle ID "YYY.WonderPushNotificationServiceExtension".
Finally, set Enable bitcode to NO in the extension target's build settings:
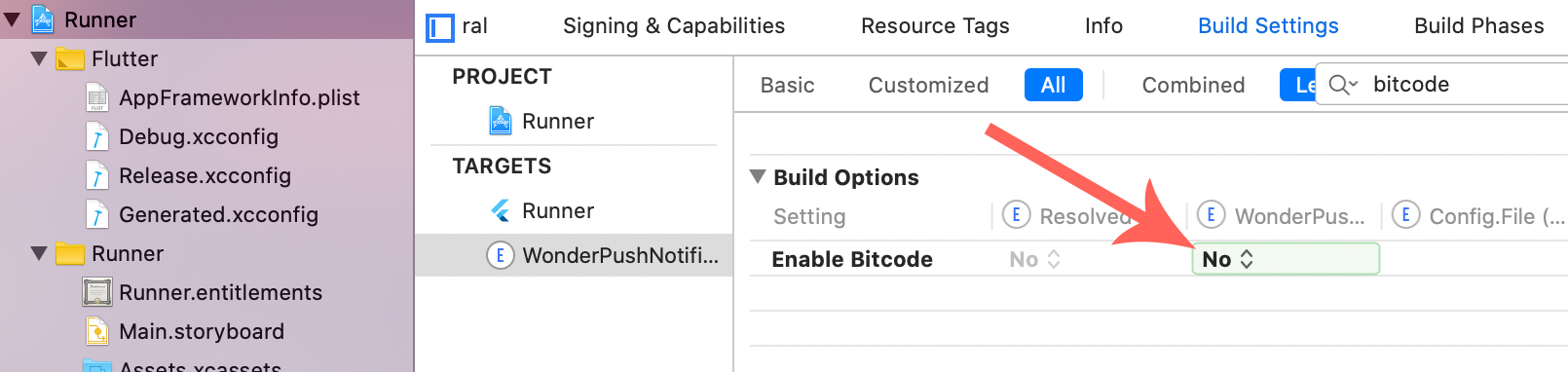
Open NotificationService.swift
or (NotificationService.h
and NotificationService.m
for Objective-C) and replace their entire contents with:
import WonderPushExtension
class NotificationService: WPNotificationServiceExtension {
override class func clientId() -> String {
return "YOUR_CLIENT_ID"
}
override class func clientSecret() -> String {
return "YOUR_CLIENT_SECRET"
}
}
#import <WonderPushExtension/WonderPushExtension.h>
@interface NotificationService : WPNotificationServiceExtension
@end
#import "NotificationService.h"
@implementation NotificationService
+ (NSString *)clientId {
return @"YOUR_CLIENT_ID";
}
+ (NSString *)clientSecret {
return @"YOUR_CLIENT_SECRET";
}
@end
Adapt YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with the values you've noted above, the same you just gave to your AppDelegate
.
Step 4. Setup CocoaPods
Open ios/Podfile
and add the following at the bottom:
target 'WonderPushNotificationServiceExtension' do
platform :ios, '10.0'
use_frameworks!
# Pods for WonderPushNotificationServiceExtension
pod 'WonderPushExtension', '~> 4.0'
end
Please close Xcode before proceeding
Open a Terminal window in your flutter project directory and type:
cd ios
pod install
If you're getting the error
Cycle inside Runner; building could produce unreliable results
and the detail of the error mentionsWonderPushNotificationServiceExtension.appex
you can try the following:
- Go to the
Build phases
screen of the mainRunner
target- Make sure the
Copy bundle resources
step is right afterLink with libraries
Congratulations!Your iOS setup should now be complete. Deploy your app on a real device (simulator can't receive push notifications), subscribe and get your first push!
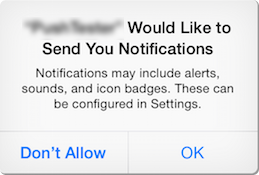
Wait a couple of minutes and receive the default welcome notification:
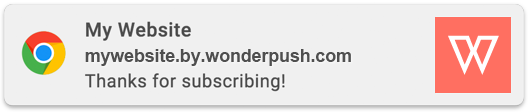
Setting up Android
Step 1. Fill your Firebase credentials in the dashboard
Follow the steps outlined in the Firebase / Filling the Firebase credentials in the WonderPush dashboard article.
Keep note of your Project number, also known as the Sender ID, as you will need in the below steps.
Step 2. Configure SDK
- Take note of your Client ID and Client Secret from the Platforms tab of the Settings page:
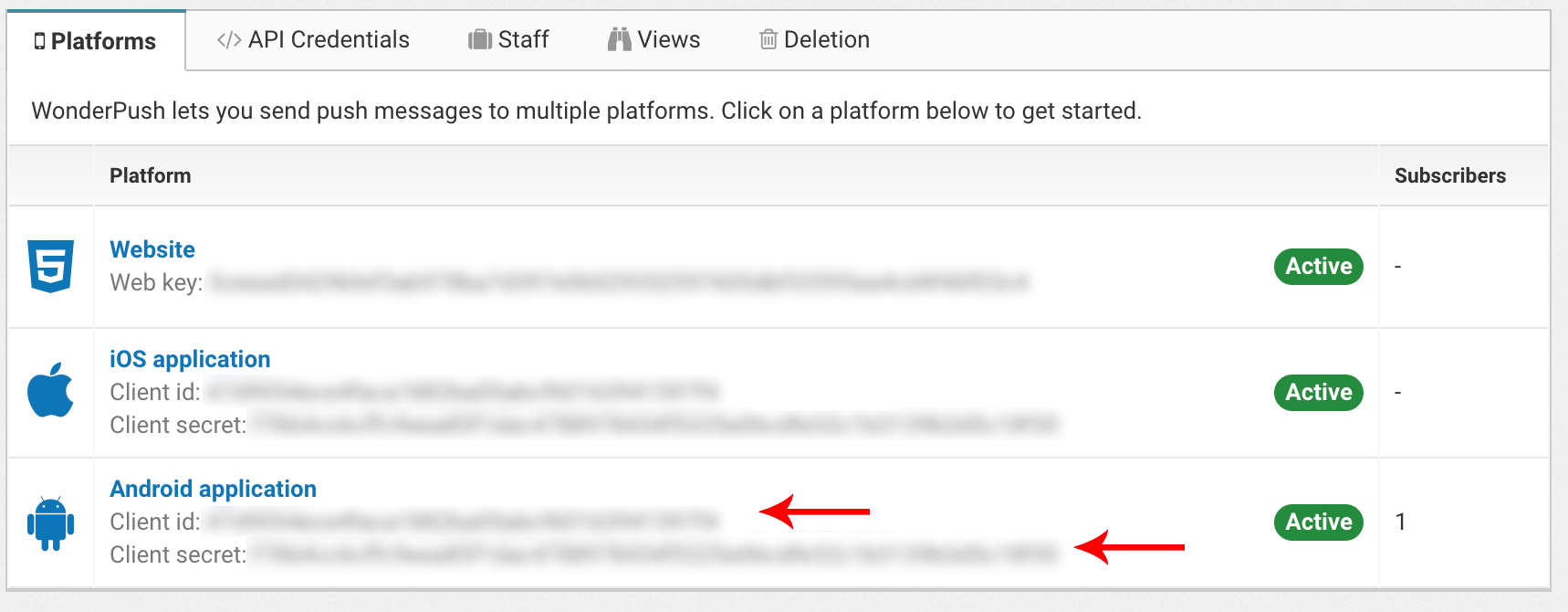
Open the Android Studio and configure the WonderPush Android SDK from your android/app/build.gradle
file:
android {
defaultConfig {
// Make sure minSdkVersion is 21 or higher
minSdkVersion 21
// Note that it's important to keep the double quotes as part of the third argument
// as this represents a string in Java code
buildConfigField 'String', 'WONDERPUSH_CLIENT_ID', '"YOUR_CLIENT_ID"'
buildConfigField 'String', 'WONDERPUSH_CLIENT_SECRET', '"YOUR_CLIENT_SECRET"'
buildConfigField 'String', 'WONDERPUSH_SENDER_ID', '"YOUR_SENDER_ID"'
buildFeatures {
buildConfig = true
}
}
}
android {
defaultConfig {
// Note that it's important to keep the double quotes as part of the third argument
// as this represents a string in Java code
buildConfigField("String", "WONDERPUSH_CLIENT_ID", "\"YOUR_CLIENT_ID\"")
buildConfigField("String", "WONDERPUSH_CLIENT_SECRET", "\"YOUR_CLIENT_SECRET\"")
buildConfigField("String", "WONDERPUSH_SENDER_ID", "\"YOUR_SENDER_ID\"")
buildFeatures {
buildConfig = true
}
}
}
Replace YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with the appropriate values you find in the Settings page.
Replace YOUR_SENDER_ID
with the Firebase Sender ID from step 1.
Click Sync now in the banner that showed up in your editor, or click the Sync project with Gradle files button in the toolbar.
Then build your project.
Solving error: Module was compiled with an incompatible version of Kotlin. The binary version of its metadata is X.Y.Z, expected version is A.B.C.in
android/settings.gradle
replaceid "org.jetbrains.kotlin.android" version "A.B.C" apply false
withid "org.jetbrains.kotlin.android" version "X.Y.Z" apply false
Congratulations, you're done!Run your app using Android studio and get your first push.
Wait a couple of minutes and receive the default welcome notification:
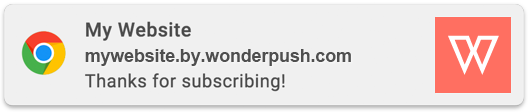
Huawei mobiles supportBecause Huawei mobiles no longer ship with Google Play Services necessary for using Firebase Cloud Messaging, you will need to follow the Huawei mobiles support guide to support them.
Updated 9 days ago